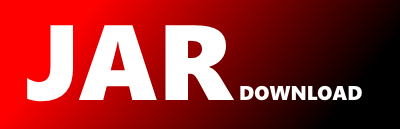
com.prowidesoftware.swift.model.mt.mt5xx.MT564 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wife Show documentation
Show all versions of wife Show documentation
Prowide Core Libraries for SWIFT (TM) messages
The newest version!
/* * This library is free software; you can redistribute it and/or * modify it under the terms of the GNU Lesser General Public * License as published by the Free Software Foundation; either * version 2.1 of the License, or (at your option) any later version. * * This library is distributed in the hope that it will be useful, * but WITHOUT ANY WARRANTY; without even the implied warranty of * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU * Lesser General Public License for more details. * */ package com.prowidesoftware.swift.model.mt.mt5xx; import java.io.Serializable; import java.util.ArrayList; import java.util.Collections; import java.util.List; import com.prowidesoftware.swift.model.*; import com.prowidesoftware.swift.model.field.*; import com.prowidesoftware.swift.model.mt.AbstractMT; /** * MT 564
if none is found.
* * * NOTE: this source code has been generated from template * * @author www.prowidesoftware.com */ public class MT564 extends AbstractMT implements Serializable { private static final long serialVersionUID = 1L; private static transient final java.util.logging.Logger log = java.util.logging.Logger.getLogger(MT564.class.getName()); /** * Creates an MT564 initialized with the parameter SwiftMessage * @param m swift message with the MT564 content */ public MT564(SwiftMessage m) { super(m); } /** * Creates an MT564 initialized with a new SwiftMessage */ public MT564() { super(); } /** * Returns this MT number * @return the message type number of this MT * @since 6.4 */ @Override public String getMessageType() { return "564"; } /** * Iterates through block4 fields and return the first one whose name matches 28E, * ornull
if none is found.
* The first occurrence of field 28E at MT564 is expected to be the only one. * * @return a Field28E object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field28E getField28E() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("28E"); if (t == null) { log.fine("field 28E not found"); return null; } else { return new Field28E(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 23G, * ornull
if none is found.
* The first occurrence of field 23G at MT564 is expected to be the only one. * * @return a Field23G object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field23G getField23G() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("23G"); if (t == null) { log.fine("field 23G not found"); return null; } else { return new Field23G(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 98A, * ornull
if none is found.
* The first occurrence of field 98A at MT564 is expected to be the only one. * * @return a Field98A object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field98A getField98A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("98A"); if (t == null) { log.fine("field 98A not found"); return null; } else { return new Field98A(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 98C, * ornull
if none is found.
* The first occurrence of field 98C at MT564 is expected to be the only one. * * @return a Field98C object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field98C getField98C() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("98C"); if (t == null) { log.fine("field 98C not found"); return null; } else { return new Field98C(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 25D, * ornull
if none is found.
* The first occurrence of field 25D at MT564 is expected to be the only one. * * @return a Field25D object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field25D getField25D() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("25D"); if (t == null) { log.fine("field 25D not found"); return null; } else { return new Field25D(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 36E, * ornull
if none is found.
* The first occurrence of field 36E at MT564 is expected to be the only one. * * @return a Field36E object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field36E getField36E() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("36E"); if (t == null) { log.fine("field 36E not found"); return null; } else { return new Field36E(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 92D, * ornull
if none is found.
* The first occurrence of field 92D at MT564 is expected to be the only one. * * @return a Field92D object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field92D getField92D() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("92D"); if (t == null) { log.fine("field 92D not found"); return null; } else { return new Field92D(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 99A, * ornull
if none is found.
* The first occurrence of field 99A at MT564 is expected to be the only one. * * @return a Field99A object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field99A getField99A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("99A"); if (t == null) { log.fine("field 99A not found"); return null; } else { return new Field99A(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 90A, * ornull
if none is found.
* The first occurrence of field 90A at MT564 is expected to be the only one. * * @return a Field90A object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field90A getField90A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("90A"); if (t == null) { log.fine("field 90A not found"); return null; } else { return new Field90A(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 90E, * ornull
if none is found.
* The first occurrence of field 90E at MT564 is expected to be the only one. * * @return a Field90E object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field90E getField90E() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("90E"); if (t == null) { log.fine("field 90E not found"); return null; } else { return new Field90E(t.getValue()); } } } /** * Iterates through block4 fields and return all occurrences of fields whose names matches 16R, * orCollections.emptyList()
if none is found.
* Multiple occurrences of field 16R at MT564 are expected at one sequence or across several sequences. * * @return a List of Field16R objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField16R() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("16R"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList()
* Multiple occurrences of field 22F at MT564 are expected at one sequence or across several sequences. * * @return a List of Field22F objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField22F() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("22F"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 13A at MT564 are expected at one sequence or across several sequences. * * @return a List of Field13A objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField13A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("13A"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 13B at MT564 are expected at one sequence or across several sequences. * * @return a List of Field13B objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField13B() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("13B"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 20C at MT564 are expected at one sequence or across several sequences. * * @return a List of Field20C objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField20C() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("20C"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 16S at MT564 are expected at one sequence or across several sequences. * * @return a List of Field16S objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField16S() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("16S"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 95P at MT564 are expected at one sequence or across several sequences. * * @return a List of Field95P objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField95P() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("95P"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 95R at MT564 are expected at one sequence or across several sequences. * * @return a List of Field95R objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField95R() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("95R"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 97A at MT564 are expected at one sequence or across several sequences. * * @return a List of Field97A objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField97A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("97A"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 97C at MT564 are expected at one sequence or across several sequences. * * @return a List of Field97C objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField97C() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("97C"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 94B at MT564 are expected at one sequence or across several sequences. * * @return a List of Field94B objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField94B() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("94B"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 94C at MT564 are expected at one sequence or across several sequences. * * @return a List of Field94C objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField94C() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("94C"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 94F at MT564 are expected at one sequence or across several sequences. * * @return a List of Field94F objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField94F() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("94F"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 35B at MT564 are expected at one sequence or across several sequences. * * @return a List of Field35B objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField35B() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("35B"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 11A at MT564 are expected at one sequence or across several sequences. * * @return a List of Field11A objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField11A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("11A"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 90B at MT564 are expected at one sequence or across several sequences. * * @return a List of Field90B objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField90B() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("90B"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 36B at MT564 are expected at one sequence or across several sequences. * * @return a List of Field36B objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField36B() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("36B"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 69A at MT564 are expected at one sequence or across several sequences. * * @return a List of Field69A objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField69A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("69A"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 69B at MT564 are expected at one sequence or across several sequences. * * @return a List of Field69B objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField69B() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("69B"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 69C at MT564 are expected at one sequence or across several sequences. * * @return a List of Field69C objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField69C() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("69C"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 69D at MT564 are expected at one sequence or across several sequences. * * @return a List of Field69D objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField69D() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("69D"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 69E at MT564 are expected at one sequence or across several sequences. * * @return a List of Field69E objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField69E() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("69E"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 69F at MT564 are expected at one sequence or across several sequences. * * @return a List of Field69F objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField69F() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("69F"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 69J at MT564 are expected at one sequence or across several sequences. * * @return a List of Field69J objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField69J() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("69J"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 97E at MT564 are expected at one sequence or across several sequences. * * @return a List of Field97E objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField97E() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("97E"); final List result = new ArrayList (); for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy