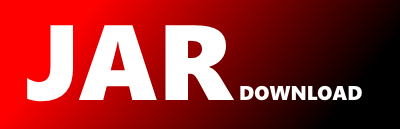
com.prowidesoftware.swift.model.mt.mt6xx.MT601 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wife Show documentation
Show all versions of wife Show documentation
Prowide Core Libraries for SWIFT (TM) messages
The newest version!
/*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
*/
package com.prowidesoftware.swift.model.mt.mt6xx;
import java.io.Serializable;
import com.prowidesoftware.swift.model.*;
import com.prowidesoftware.swift.model.field.*;
import com.prowidesoftware.swift.model.mt.AbstractMT;
/**
* MT 601
*
*
* NOTE: this source code has been generated from template
*
* @author www.prowidesoftware.com
*/
public class MT601 extends AbstractMT implements Serializable {
private static final long serialVersionUID = 1L;
private static transient final java.util.logging.Logger log = java.util.logging.Logger.getLogger(MT601.class.getName());
/**
* Creates an MT601 initialized with the parameter SwiftMessage
* @param m swift message with the MT601 content
*/
public MT601(SwiftMessage m) {
super(m);
}
/**
* Creates an MT601 initialized with a new SwiftMessage
*/
public MT601() {
super();
}
/**
* Returns this MT number
* @return the message type number of this MT
* @since 6.4
*/
@Override
public String getMessageType() {
return "601";
}
/**
* Iterates through block4 fields and return the first one whose name matches 20,
* or null
if none is found.
* The first occurrence of field 20 at MT601 is expected to be the only one.
*
* @return a Field20 object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field20 getField20() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("20");
if (t == null) {
log.fine("field 20 not found");
return null;
} else {
return new Field20(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 21,
* or null
if none is found.
* The first occurrence of field 21 at MT601 is expected to be the only one.
*
* @return a Field21 object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field21 getField21() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("21");
if (t == null) {
log.fine("field 21 not found");
return null;
} else {
return new Field21(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 22,
* or null
if none is found.
* The first occurrence of field 22 at MT601 is expected to be the only one.
*
* @return a Field22 object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field22 getField22() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("22");
if (t == null) {
log.fine("field 22 not found");
return null;
} else {
return new Field22(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 94A,
* or null
if none is found.
* The first occurrence of field 94A at MT601 is expected to be the only one.
*
* @return a Field94A object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field94A getField94A() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("94A");
if (t == null) {
log.fine("field 94A not found");
return null;
} else {
return new Field94A(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 82A,
* or null
if none is found.
* The first occurrence of field 82A at MT601 is expected to be the only one.
*
* @return a Field82A object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field82A getField82A() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("82A");
if (t == null) {
log.fine("field 82A not found");
return null;
} else {
return new Field82A(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 82D,
* or null
if none is found.
* The first occurrence of field 82D at MT601 is expected to be the only one.
*
* @return a Field82D object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field82D getField82D() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("82D");
if (t == null) {
log.fine("field 82D not found");
return null;
} else {
return new Field82D(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 82J,
* or null
if none is found.
* The first occurrence of field 82J at MT601 is expected to be the only one.
*
* @return a Field82J object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field82J getField82J() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("82J");
if (t == null) {
log.fine("field 82J not found");
return null;
} else {
return new Field82J(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 87A,
* or null
if none is found.
* The first occurrence of field 87A at MT601 is expected to be the only one.
*
* @return a Field87A object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field87A getField87A() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("87A");
if (t == null) {
log.fine("field 87A not found");
return null;
} else {
return new Field87A(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 87D,
* or null
if none is found.
* The first occurrence of field 87D at MT601 is expected to be the only one.
*
* @return a Field87D object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field87D getField87D() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("87D");
if (t == null) {
log.fine("field 87D not found");
return null;
} else {
return new Field87D(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 87J,
* or null
if none is found.
* The first occurrence of field 87J at MT601 is expected to be the only one.
*
* @return a Field87J object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field87J getField87J() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("87J");
if (t == null) {
log.fine("field 87J not found");
return null;
} else {
return new Field87J(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 83A,
* or null
if none is found.
* The first occurrence of field 83A at MT601 is expected to be the only one.
*
* @return a Field83A object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field83A getField83A() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("83A");
if (t == null) {
log.fine("field 83A not found");
return null;
} else {
return new Field83A(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 83D,
* or null
if none is found.
* The first occurrence of field 83D at MT601 is expected to be the only one.
*
* @return a Field83D object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field83D getField83D() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("83D");
if (t == null) {
log.fine("field 83D not found");
return null;
} else {
return new Field83D(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 83J,
* or null
if none is found.
* The first occurrence of field 83J at MT601 is expected to be the only one.
*
* @return a Field83J object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field83J getField83J() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("83J");
if (t == null) {
log.fine("field 83J not found");
return null;
} else {
return new Field83J(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 23,
* or null
if none is found.
* The first occurrence of field 23 at MT601 is expected to be the only one.
*
* @return a Field23 object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field23 getField23() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("23");
if (t == null) {
log.fine("field 23 not found");
return null;
} else {
return new Field23(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 30,
* or null
if none is found.
* The first occurrence of field 30 at MT601 is expected to be the only one.
*
* @return a Field30 object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field30 getField30() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("30");
if (t == null) {
log.fine("field 30 not found");
return null;
} else {
return new Field30(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 26C,
* or null
if none is found.
* The first occurrence of field 26C at MT601 is expected to be the only one.
*
* @return a Field26C object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field26C getField26C() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("26C");
if (t == null) {
log.fine("field 26C not found");
return null;
} else {
return new Field26C(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 31C,
* or null
if none is found.
* The first occurrence of field 31C at MT601 is expected to be the only one.
*
* @return a Field31C object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field31C getField31C() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("31C");
if (t == null) {
log.fine("field 31C not found");
return null;
} else {
return new Field31C(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 31G,
* or null
if none is found.
* The first occurrence of field 31G at MT601 is expected to be the only one.
*
* @return a Field31G object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field31G getField31G() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("31G");
if (t == null) {
log.fine("field 31G not found");
return null;
} else {
return new Field31G(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 31E,
* or null
if none is found.
* The first occurrence of field 31E at MT601 is expected to be the only one.
*
* @return a Field31E object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field31E getField31E() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("31E");
if (t == null) {
log.fine("field 31E not found");
return null;
} else {
return new Field31E(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 32F,
* or null
if none is found.
* The first occurrence of field 32F at MT601 is expected to be the only one.
*
* @return a Field32F object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field32F getField32F() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("32F");
if (t == null) {
log.fine("field 32F not found");
return null;
} else {
return new Field32F(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 32B,
* or null
if none is found.
* The first occurrence of field 32B at MT601 is expected to be the only one.
*
* @return a Field32B object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field32B getField32B() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("32B");
if (t == null) {
log.fine("field 32B not found");
return null;
} else {
return new Field32B(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 33B,
* or null
if none is found.
* The first occurrence of field 33B at MT601 is expected to be the only one.
*
* @return a Field33B object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field33B getField33B() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("33B");
if (t == null) {
log.fine("field 33B not found");
return null;
} else {
return new Field33B(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 34P,
* or null
if none is found.
* The first occurrence of field 34P at MT601 is expected to be the only one.
*
* @return a Field34P object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field34P getField34P() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("34P");
if (t == null) {
log.fine("field 34P not found");
return null;
} else {
return new Field34P(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 34R,
* or null
if none is found.
* The first occurrence of field 34R at MT601 is expected to be the only one.
*
* @return a Field34R object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field34R getField34R() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("34R");
if (t == null) {
log.fine("field 34R not found");
return null;
} else {
return new Field34R(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 53A,
* or null
if none is found.
* The first occurrence of field 53A at MT601 is expected to be the only one.
*
* @return a Field53A object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field53A getField53A() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("53A");
if (t == null) {
log.fine("field 53A not found");
return null;
} else {
return new Field53A(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 53B,
* or null
if none is found.
* The first occurrence of field 53B at MT601 is expected to be the only one.
*
* @return a Field53B object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field53B getField53B() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("53B");
if (t == null) {
log.fine("field 53B not found");
return null;
} else {
return new Field53B(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 53D,
* or null
if none is found.
* The first occurrence of field 53D at MT601 is expected to be the only one.
*
* @return a Field53D object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field53D getField53D() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("53D");
if (t == null) {
log.fine("field 53D not found");
return null;
} else {
return new Field53D(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 56A,
* or null
if none is found.
* The first occurrence of field 56A at MT601 is expected to be the only one.
*
* @return a Field56A object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field56A getField56A() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("56A");
if (t == null) {
log.fine("field 56A not found");
return null;
} else {
return new Field56A(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 56B,
* or null
if none is found.
* The first occurrence of field 56B at MT601 is expected to be the only one.
*
* @return a Field56B object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field56B getField56B() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("56B");
if (t == null) {
log.fine("field 56B not found");
return null;
} else {
return new Field56B(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 56D,
* or null
if none is found.
* The first occurrence of field 56D at MT601 is expected to be the only one.
*
* @return a Field56D object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field56D getField56D() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("56D");
if (t == null) {
log.fine("field 56D not found");
return null;
} else {
return new Field56D(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 57A,
* or null
if none is found.
* The first occurrence of field 57A at MT601 is expected to be the only one.
*
* @return a Field57A object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field57A getField57A() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("57A");
if (t == null) {
log.fine("field 57A not found");
return null;
} else {
return new Field57A(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 57B,
* or null
if none is found.
* The first occurrence of field 57B at MT601 is expected to be the only one.
*
* @return a Field57B object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field57B getField57B() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("57B");
if (t == null) {
log.fine("field 57B not found");
return null;
} else {
return new Field57B(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 57D,
* or null
if none is found.
* The first occurrence of field 57D at MT601 is expected to be the only one.
*
* @return a Field57D object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field57D getField57D() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("57D");
if (t == null) {
log.fine("field 57D not found");
return null;
} else {
return new Field57D(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 77H,
* or null
if none is found.
* The first occurrence of field 77H at MT601 is expected to be the only one.
*
* @return a Field77H object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field77H getField77H() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("77H");
if (t == null) {
log.fine("field 77H not found");
return null;
} else {
return new Field77H(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 77D,
* or null
if none is found.
* The first occurrence of field 77D at MT601 is expected to be the only one.
*
* @return a Field77D object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field77D getField77D() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("77D");
if (t == null) {
log.fine("field 77D not found");
return null;
} else {
return new Field77D(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 14C,
* or null
if none is found.
* The first occurrence of field 14C at MT601 is expected to be the only one.
*
* @return a Field14C object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field14C getField14C() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("14C");
if (t == null) {
log.fine("field 14C not found");
return null;
} else {
return new Field14C(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 72,
* or null
if none is found.
* The first occurrence of field 72 at MT601 is expected to be the only one.
*
* @return a Field72 object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field72 getField72() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("72");
if (t == null) {
log.fine("field 72 not found");
return null;
} else {
return new Field72(t.getValue());
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy