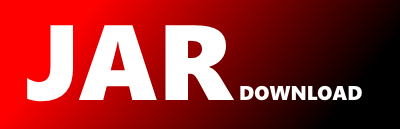
com.prowidesoftware.swift.model.mt.mt7xx.MT798_794 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wife Show documentation
Show all versions of wife Show documentation
Prowide Core Libraries for SWIFT (TM) messages
The newest version!
/*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
*/
package com.prowidesoftware.swift.model.mt.mt7xx;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import com.prowidesoftware.swift.model.*;
import com.prowidesoftware.swift.model.field.*;
import com.prowidesoftware.swift.model.mt.AbstractMT;
/**
* MT 798_794
*
*
* NOTE: this source code has been generated from template
*
* @author www.prowidesoftware.com
*/
public class MT798_794 extends AbstractMT implements Serializable {
private static final long serialVersionUID = 1L;
private static transient final java.util.logging.Logger log = java.util.logging.Logger.getLogger(MT798_794.class.getName());
/**
* Creates an MT798_794 initialized with the parameter SwiftMessage
* @param m swift message with the MT798_794 content
*/
public MT798_794(SwiftMessage m) {
super(m);
}
/**
* Creates an MT798_794 initialized with a new SwiftMessage
*/
public MT798_794() {
super();
}
/**
* Returns this MT number
* @return the message type number of this MT
* @since 6.4
*/
@Override
public String getMessageType() {
return "798794";
}
/**
* Iterates through block4 fields and return the first one whose name matches 12,
* or null
if none is found.
* The first occurrence of field 12 at MT798_794 is expected to be the only one.
*
* @return a Field12 object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field12 getField12() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("12");
if (t == null) {
log.fine("field 12 not found");
return null;
} else {
return new Field12(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 77E,
* or null
if none is found.
* The first occurrence of field 77E at MT798_794 is expected to be the only one.
*
* @return a Field77E object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field77E getField77E() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("77E");
if (t == null) {
log.fine("field 77E not found");
return null;
} else {
return new Field77E(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 27A,
* or null
if none is found.
* The first occurrence of field 27A at MT798_794 is expected to be the only one.
*
* @return a Field27A object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field27A getField27A() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("27A");
if (t == null) {
log.fine("field 27A not found");
return null;
} else {
return new Field27A(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 21P,
* or null
if none is found.
* The first occurrence of field 21P at MT798_794 is expected to be the only one.
*
* @return a Field21P object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field21P getField21P() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("21P");
if (t == null) {
log.fine("field 21P not found");
return null;
} else {
return new Field21P(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 21A,
* or null
if none is found.
* The first occurrence of field 21A at MT798_794 is expected to be the only one.
*
* @return a Field21A object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field21A getField21A() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("21A");
if (t == null) {
log.fine("field 21A not found");
return null;
} else {
return new Field21A(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 31C,
* or null
if none is found.
* The first occurrence of field 31C at MT798_794 is expected to be the only one.
*
* @return a Field31C object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field31C getField31C() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("31C");
if (t == null) {
log.fine("field 31C not found");
return null;
} else {
return new Field31C(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 13E,
* or null
if none is found.
* The first occurrence of field 13E at MT798_794 is expected to be the only one.
*
* @return a Field13E object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field13E getField13E() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("13E");
if (t == null) {
log.fine("field 13E not found");
return null;
} else {
return new Field13E(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 52A,
* or null
if none is found.
* The first occurrence of field 52A at MT798_794 is expected to be the only one.
*
* @return a Field52A object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field52A getField52A() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("52A");
if (t == null) {
log.fine("field 52A not found");
return null;
} else {
return new Field52A(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 52D,
* or null
if none is found.
* The first occurrence of field 52D at MT798_794 is expected to be the only one.
*
* @return a Field52D object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field52D getField52D() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("52D");
if (t == null) {
log.fine("field 52D not found");
return null;
} else {
return new Field52D(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 29B,
* or null
if none is found.
* The first occurrence of field 29B at MT798_794 is expected to be the only one.
*
* @return a Field29B object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field29B getField29B() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("29B");
if (t == null) {
log.fine("field 29B not found");
return null;
} else {
return new Field29B(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 58A,
* or null
if none is found.
* The first occurrence of field 58A at MT798_794 is expected to be the only one.
*
* @return a Field58A object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field58A getField58A() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("58A");
if (t == null) {
log.fine("field 58A not found");
return null;
} else {
return new Field58A(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 58D,
* or null
if none is found.
* The first occurrence of field 58D at MT798_794 is expected to be the only one.
*
* @return a Field58D object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field58D getField58D() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("58D");
if (t == null) {
log.fine("field 58D not found");
return null;
} else {
return new Field58D(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 29D,
* or null
if none is found.
* The first occurrence of field 29D at MT798_794 is expected to be the only one.
*
* @return a Field29D object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field29D getField29D() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("29D");
if (t == null) {
log.fine("field 29D not found");
return null;
} else {
return new Field29D(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 32B,
* or null
if none is found.
* The first occurrence of field 32B at MT798_794 is expected to be the only one.
*
* @return a Field32B object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field32B getField32B() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("32B");
if (t == null) {
log.fine("field 32B not found");
return null;
} else {
return new Field32B(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 72C,
* or null
if none is found.
* The first occurrence of field 72C at MT798_794 is expected to be the only one.
*
* @return a Field72C object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field72C getField72C() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("72C");
if (t == null) {
log.fine("field 72C not found");
return null;
} else {
return new Field72C(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return all occurrences of fields whose names matches 20,
* or Collections.emptyList()
if none is found.
* Multiple occurrences of field 20 at MT798_794 are expected at one sequence or across several sequences.
*
* @return a List of Field20 objects or Collections.emptyList()
if none is not found
* @see SwiftTagListBlock#getTagsByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public List getField20() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return Collections.emptyList();
} else {
final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("20");
final List result = new ArrayList();
for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy