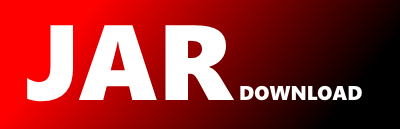
com.pulumi.akamai.inputs.CloudletsApplicationLoadBalancerState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of akamai Show documentation
Show all versions of akamai Show documentation
A Pulumi package for creating and managing akamai cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.akamai.inputs;
import com.pulumi.akamai.inputs.CloudletsApplicationLoadBalancerDataCenterArgs;
import com.pulumi.akamai.inputs.CloudletsApplicationLoadBalancerLivenessSettingsArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class CloudletsApplicationLoadBalancerState extends com.pulumi.resources.ResourceArgs {
public static final CloudletsApplicationLoadBalancerState Empty = new CloudletsApplicationLoadBalancerState();
/**
* The type of load balancing being performed. Options include WEIGHTED and PERFORMANCE
*
*/
@Import(name="balancingType")
private @Nullable Output balancingType;
/**
* @return The type of load balancing being performed. Options include WEIGHTED and PERFORMANCE
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy