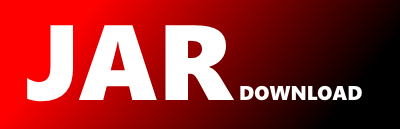
com.pulumi.akamai.inputs.GetPropertyRulesBuilderRulesV20230105 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of akamai Show documentation
Show all versions of akamai Show documentation
A Pulumi package for creating and managing akamai cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.akamai.inputs;
import com.pulumi.akamai.inputs.GetPropertyRulesBuilderRulesV20230105Behavior;
import com.pulumi.akamai.inputs.GetPropertyRulesBuilderRulesV20230105Criterion;
import com.pulumi.akamai.inputs.GetPropertyRulesBuilderRulesV20230105CustomOverride;
import com.pulumi.akamai.inputs.GetPropertyRulesBuilderRulesV20230105Variable;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class GetPropertyRulesBuilderRulesV20230105 extends com.pulumi.resources.InvokeArgs {
public static final GetPropertyRulesBuilderRulesV20230105 Empty = new GetPropertyRulesBuilderRulesV20230105();
/**
* XML metadata of the rule
*
*/
@Import(name="advancedOverride")
private @Nullable String advancedOverride;
/**
* @return XML metadata of the rule
*
*/
public Optional advancedOverride() {
return Optional.ofNullable(this.advancedOverride);
}
/**
* The list of behaviors for a rule
*
*/
@Import(name="behaviors")
private @Nullable List behaviors;
/**
* @return The list of behaviors for a rule
*
*/
public Optional> behaviors() {
return Optional.ofNullable(this.behaviors);
}
/**
* A list of child rules for a particular rule in JSON format
*
*/
@Import(name="childrens")
private @Nullable List childrens;
/**
* @return A list of child rules for a particular rule in JSON format
*
*/
public Optional> childrens() {
return Optional.ofNullable(this.childrens);
}
/**
* The comments for a rule
*
*/
@Import(name="comments")
private @Nullable String comments;
/**
* @return The comments for a rule
*
*/
public Optional comments() {
return Optional.ofNullable(this.comments);
}
/**
* States whether changes to 'criterion' objects are prohibited
*
*/
@Import(name="criteriaLocked")
private @Nullable Boolean criteriaLocked;
/**
* @return States whether changes to 'criterion' objects are prohibited
*
*/
public Optional criteriaLocked() {
return Optional.ofNullable(this.criteriaLocked);
}
/**
* States whether 'all' criteria need to match or 'any'
*
*/
@Import(name="criteriaMustSatisfy")
private @Nullable String criteriaMustSatisfy;
/**
* @return States whether 'all' criteria need to match or 'any'
*
*/
public Optional criteriaMustSatisfy() {
return Optional.ofNullable(this.criteriaMustSatisfy);
}
/**
* The list of criteria for a rule
*
*/
@Import(name="criterions")
private @Nullable List criterions;
/**
* @return The list of criteria for a rule
*
*/
public Optional> criterions() {
return Optional.ofNullable(this.criterions);
}
/**
* XML metadata of the rule
*
*/
@Import(name="customOverride")
private @Nullable GetPropertyRulesBuilderRulesV20230105CustomOverride customOverride;
/**
* @return XML metadata of the rule
*
*/
public Optional customOverride() {
return Optional.ofNullable(this.customOverride);
}
/**
* States whether a rule is secure
*
*/
@Import(name="isSecure")
private @Nullable Boolean isSecure;
/**
* @return States whether a rule is secure
*
*/
public Optional isSecure() {
return Optional.ofNullable(this.isSecure);
}
/**
* The name of a rule
*
*/
@Import(name="name", required=true)
private String name;
/**
* @return The name of a rule
*
*/
public String name() {
return this.name;
}
/**
* The template link for the rule
*
*/
@Import(name="templateLink")
private @Nullable String templateLink;
/**
* @return The template link for the rule
*
*/
public Optional templateLink() {
return Optional.ofNullable(this.templateLink);
}
/**
* The UUID of a rule template
*
*/
@Import(name="templateUuid")
private @Nullable String templateUuid;
/**
* @return The UUID of a rule template
*
*/
public Optional templateUuid() {
return Optional.ofNullable(this.templateUuid);
}
/**
* The UUID of the rule
*
*/
@Import(name="uuid")
private @Nullable String uuid;
/**
* @return The UUID of the rule
*
*/
public Optional uuid() {
return Optional.ofNullable(this.uuid);
}
/**
* A list of variables for a rule
*
*/
@Import(name="variables")
private @Nullable List variables;
/**
* @return A list of variables for a rule
*
*/
public Optional> variables() {
return Optional.ofNullable(this.variables);
}
private GetPropertyRulesBuilderRulesV20230105() {}
private GetPropertyRulesBuilderRulesV20230105(GetPropertyRulesBuilderRulesV20230105 $) {
this.advancedOverride = $.advancedOverride;
this.behaviors = $.behaviors;
this.childrens = $.childrens;
this.comments = $.comments;
this.criteriaLocked = $.criteriaLocked;
this.criteriaMustSatisfy = $.criteriaMustSatisfy;
this.criterions = $.criterions;
this.customOverride = $.customOverride;
this.isSecure = $.isSecure;
this.name = $.name;
this.templateLink = $.templateLink;
this.templateUuid = $.templateUuid;
this.uuid = $.uuid;
this.variables = $.variables;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetPropertyRulesBuilderRulesV20230105 defaults) {
return new Builder(defaults);
}
public static final class Builder {
private GetPropertyRulesBuilderRulesV20230105 $;
public Builder() {
$ = new GetPropertyRulesBuilderRulesV20230105();
}
public Builder(GetPropertyRulesBuilderRulesV20230105 defaults) {
$ = new GetPropertyRulesBuilderRulesV20230105(Objects.requireNonNull(defaults));
}
/**
* @param advancedOverride XML metadata of the rule
*
* @return builder
*
*/
public Builder advancedOverride(@Nullable String advancedOverride) {
$.advancedOverride = advancedOverride;
return this;
}
/**
* @param behaviors The list of behaviors for a rule
*
* @return builder
*
*/
public Builder behaviors(@Nullable List behaviors) {
$.behaviors = behaviors;
return this;
}
/**
* @param behaviors The list of behaviors for a rule
*
* @return builder
*
*/
public Builder behaviors(GetPropertyRulesBuilderRulesV20230105Behavior... behaviors) {
return behaviors(List.of(behaviors));
}
/**
* @param childrens A list of child rules for a particular rule in JSON format
*
* @return builder
*
*/
public Builder childrens(@Nullable List childrens) {
$.childrens = childrens;
return this;
}
/**
* @param childrens A list of child rules for a particular rule in JSON format
*
* @return builder
*
*/
public Builder childrens(String... childrens) {
return childrens(List.of(childrens));
}
/**
* @param comments The comments for a rule
*
* @return builder
*
*/
public Builder comments(@Nullable String comments) {
$.comments = comments;
return this;
}
/**
* @param criteriaLocked States whether changes to 'criterion' objects are prohibited
*
* @return builder
*
*/
public Builder criteriaLocked(@Nullable Boolean criteriaLocked) {
$.criteriaLocked = criteriaLocked;
return this;
}
/**
* @param criteriaMustSatisfy States whether 'all' criteria need to match or 'any'
*
* @return builder
*
*/
public Builder criteriaMustSatisfy(@Nullable String criteriaMustSatisfy) {
$.criteriaMustSatisfy = criteriaMustSatisfy;
return this;
}
/**
* @param criterions The list of criteria for a rule
*
* @return builder
*
*/
public Builder criterions(@Nullable List criterions) {
$.criterions = criterions;
return this;
}
/**
* @param criterions The list of criteria for a rule
*
* @return builder
*
*/
public Builder criterions(GetPropertyRulesBuilderRulesV20230105Criterion... criterions) {
return criterions(List.of(criterions));
}
/**
* @param customOverride XML metadata of the rule
*
* @return builder
*
*/
public Builder customOverride(@Nullable GetPropertyRulesBuilderRulesV20230105CustomOverride customOverride) {
$.customOverride = customOverride;
return this;
}
/**
* @param isSecure States whether a rule is secure
*
* @return builder
*
*/
public Builder isSecure(@Nullable Boolean isSecure) {
$.isSecure = isSecure;
return this;
}
/**
* @param name The name of a rule
*
* @return builder
*
*/
public Builder name(String name) {
$.name = name;
return this;
}
/**
* @param templateLink The template link for the rule
*
* @return builder
*
*/
public Builder templateLink(@Nullable String templateLink) {
$.templateLink = templateLink;
return this;
}
/**
* @param templateUuid The UUID of a rule template
*
* @return builder
*
*/
public Builder templateUuid(@Nullable String templateUuid) {
$.templateUuid = templateUuid;
return this;
}
/**
* @param uuid The UUID of the rule
*
* @return builder
*
*/
public Builder uuid(@Nullable String uuid) {
$.uuid = uuid;
return this;
}
/**
* @param variables A list of variables for a rule
*
* @return builder
*
*/
public Builder variables(@Nullable List variables) {
$.variables = variables;
return this;
}
/**
* @param variables A list of variables for a rule
*
* @return builder
*
*/
public Builder variables(GetPropertyRulesBuilderRulesV20230105Variable... variables) {
return variables(List.of(variables));
}
public GetPropertyRulesBuilderRulesV20230105 build() {
if ($.name == null) {
throw new MissingRequiredPropertyException("GetPropertyRulesBuilderRulesV20230105", "name");
}
return $;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy