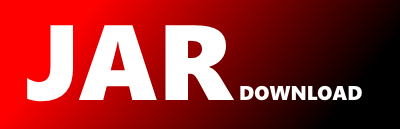
com.pulumi.akamai.inputs.GetPropertyRulesBuilderRulesV20230920BehaviorEcmsDatasetArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of akamai Show documentation
Show all versions of akamai Show documentation
A Pulumi package for creating and managing akamai cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.akamai.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class GetPropertyRulesBuilderRulesV20230920BehaviorEcmsDatasetArgs extends com.pulumi.resources.ResourceArgs {
public static final GetPropertyRulesBuilderRulesV20230920BehaviorEcmsDatasetArgs Empty = new GetPropertyRulesBuilderRulesV20230920BehaviorEcmsDatasetArgs();
/**
* Specifies a default data set for this property. If you don't configure a default database in the `ecmsDatabase` behavior, requests to objects in this data set follow the pattern: `<hostname>/datastore/<database_name>/<object_key>`.
*
*/
@Import(name="dataset")
private @Nullable Output dataset;
/**
* @return Specifies a default data set for this property. If you don't configure a default database in the `ecmsDatabase` behavior, requests to objects in this data set follow the pattern: `<hostname>/datastore/<database_name>/<object_key>`.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy