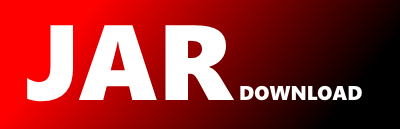
com.pulumi.akamai.inputs.GetPropertyRulesBuilderRulesV20240109BehaviorClientCertificateAuthArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of akamai Show documentation
Show all versions of akamai Show documentation
A Pulumi package for creating and managing akamai cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.akamai.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class GetPropertyRulesBuilderRulesV20240109BehaviorClientCertificateAuthArgs extends com.pulumi.resources.ResourceArgs {
public static final GetPropertyRulesBuilderRulesV20240109BehaviorClientCertificateAuthArgs Empty = new GetPropertyRulesBuilderRulesV20240109BehaviorClientCertificateAuthArgs();
/**
* Specify client certificate attributes to include in the `Client-To-Edge` authentication header that's sent to your origin server.
*
*/
@Import(name="clientCertificateAttributes")
private @Nullable Output> clientCertificateAttributes;
/**
* @return Specify client certificate attributes to include in the `Client-To-Edge` authentication header that's sent to your origin server.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy