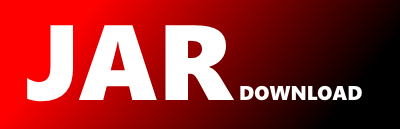
com.pulumi.akamai.inputs.GetPropertyRulesBuilderRulesV20240109BehaviorOriginFailureRecoveryPolicy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of akamai Show documentation
Show all versions of akamai Show documentation
A Pulumi package for creating and managing akamai cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.akamai.inputs;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class GetPropertyRulesBuilderRulesV20240109BehaviorOriginFailureRecoveryPolicy extends com.pulumi.resources.InvokeArgs {
public static final GetPropertyRulesBuilderRulesV20240109BehaviorOriginFailureRecoveryPolicy Empty = new GetPropertyRulesBuilderRulesV20240109BehaviorOriginFailureRecoveryPolicy();
/**
* Synchronizes content between the primary and backup origins, byte for byte.
*
*/
@Import(name="binaryEquivalentContent")
private @Nullable Boolean binaryEquivalentContent;
/**
* @return Synchronizes content between the primary and backup origins, byte for byte.
*
*/
public Optional binaryEquivalentContent() {
return Optional.ofNullable(this.binaryEquivalentContent);
}
/**
* Temporarily blocks an origin IP address that experienced a certain number of failures. When an IP address is blocked, the `configName` established for `originResponsivenessRecoveryConfigName` is applied.
*
*/
@Import(name="enableIpAvoidance")
private @Nullable Boolean enableIpAvoidance;
/**
* @return Temporarily blocks an origin IP address that experienced a certain number of failures. When an IP address is blocked, the `configName` established for `originResponsivenessRecoveryConfigName` is applied.
*
*/
public Optional enableIpAvoidance() {
return Optional.ofNullable(this.enableIpAvoidance);
}
/**
* Activates and configures a recovery policy.
*
*/
@Import(name="enabled")
private @Nullable Boolean enabled;
/**
* @return Activates and configures a recovery policy.
*
*/
public Optional enabled() {
return Optional.ofNullable(this.enabled);
}
/**
* Defines the number of failures that need to occur to an origin address before it's blocked.
*
*/
@Import(name="ipAvoidanceErrorThreshold")
private @Nullable Integer ipAvoidanceErrorThreshold;
/**
* @return Defines the number of failures that need to occur to an origin address before it's blocked.
*
*/
public Optional ipAvoidanceErrorThreshold() {
return Optional.ofNullable(this.ipAvoidanceErrorThreshold);
}
/**
* Defines the number of seconds after which the IP address is removed from the blocklist.
*
*/
@Import(name="ipAvoidanceRetryInterval")
private @Nullable Integer ipAvoidanceRetryInterval;
/**
* @return Defines the number of seconds after which the IP address is removed from the blocklist.
*
*/
public Optional ipAvoidanceRetryInterval() {
return Optional.ofNullable(this.ipAvoidanceRetryInterval);
}
/**
* Indicates that your Akamai representative has locked this behavior or criteria so that you can't modify it. This option is for internal usage only.
*
*/
@Import(name="locked")
private @Nullable Boolean locked;
/**
* @return Indicates that your Akamai representative has locked this behavior or criteria so that you can't modify it. This option is for internal usage only.
*
*/
public Optional locked() {
return Optional.ofNullable(this.locked);
}
/**
* Enables continuous monitoring of connectivity to the origin. If necessary, applies retry or recovery actions.
*
*/
@Import(name="monitorOriginResponsiveness")
private @Nullable Boolean monitorOriginResponsiveness;
/**
* @return Enables continuous monitoring of connectivity to the origin. If necessary, applies retry or recovery actions.
*
*/
public Optional monitorOriginResponsiveness() {
return Optional.ofNullable(this.monitorOriginResponsiveness);
}
/**
* Defines the origin response codes that trigger a subsequent retry or recovery action. Specify a single code entry (`501`) or a range (`501:504`). If you configure other `monitorStatusCodes*` and `monitorResponseCodes*` options, you can't use the same codes here.
*
*/
@Import(name="monitorResponseCodes1s")
private @Nullable List monitorResponseCodes1s;
/**
* @return Defines the origin response codes that trigger a subsequent retry or recovery action. Specify a single code entry (`501`) or a range (`501:504`). If you configure other `monitorStatusCodes*` and `monitorResponseCodes*` options, you can't use the same codes here.
*
*/
public Optional> monitorResponseCodes1s() {
return Optional.ofNullable(this.monitorResponseCodes1s);
}
/**
* Defines the origin response codes that trigger a subsequent retry or recovery action. Specify a single code entry (`501`) or a range (`501:504`). If you configure other `monitorStatusCodes*` and `monitorResponseCodes*` options, you can't use the same codes here.
*
*/
@Import(name="monitorResponseCodes2s")
private @Nullable List monitorResponseCodes2s;
/**
* @return Defines the origin response codes that trigger a subsequent retry or recovery action. Specify a single code entry (`501`) or a range (`501:504`). If you configure other `monitorStatusCodes*` and `monitorResponseCodes*` options, you can't use the same codes here.
*
*/
public Optional> monitorResponseCodes2s() {
return Optional.ofNullable(this.monitorResponseCodes2s);
}
/**
* Defines the origin response codes that trigger a subsequent retry or recovery action. Specify a single code entry (`501`) or a range (`501:504`). If you configure other `monitorStatusCodes*` and `monitorResponseCodes*` options, you can't use the same codes here..
*
*/
@Import(name="monitorResponseCodes3s")
private @Nullable List monitorResponseCodes3s;
/**
* @return Defines the origin response codes that trigger a subsequent retry or recovery action. Specify a single code entry (`501`) or a range (`501:504`). If you configure other `monitorStatusCodes*` and `monitorResponseCodes*` options, you can't use the same codes here..
*
*/
public Optional> monitorResponseCodes3s() {
return Optional.ofNullable(this.monitorResponseCodes3s);
}
/**
* Enables continuous monitoring for the specific origin status codes that trigger retry or recovery actions.
*
*/
@Import(name="monitorStatusCodes1")
private @Nullable Boolean monitorStatusCodes1;
/**
* @return Enables continuous monitoring for the specific origin status codes that trigger retry or recovery actions.
*
*/
public Optional monitorStatusCodes1() {
return Optional.ofNullable(this.monitorStatusCodes1);
}
/**
* Enables the recovery action for the response codes you define.
*
*/
@Import(name="monitorStatusCodes1EnableRecovery")
private @Nullable Boolean monitorStatusCodes1EnableRecovery;
/**
* @return Enables the recovery action for the response codes you define.
*
*/
public Optional monitorStatusCodes1EnableRecovery() {
return Optional.ofNullable(this.monitorStatusCodes1EnableRecovery);
}
/**
* When the defined response codes apply, attempts a retry on the same origin before executing the recovery method.
*
*/
@Import(name="monitorStatusCodes1EnableRetry")
private @Nullable Boolean monitorStatusCodes1EnableRetry;
/**
* @return When the defined response codes apply, attempts a retry on the same origin before executing the recovery method.
*
*/
public Optional monitorStatusCodes1EnableRetry() {
return Optional.ofNullable(this.monitorStatusCodes1EnableRetry);
}
/**
* Specifies a recovery configuration using the `configName` you defined in the `recoveryConfig` match criteria. Specify 3 to 20 alphanumeric characters or dashes. Ensure that you use the `recoveryConfig` match criteria to apply this option.
*
*/
@Import(name="monitorStatusCodes1RecoveryConfigName")
private @Nullable String monitorStatusCodes1RecoveryConfigName;
/**
* @return Specifies a recovery configuration using the `configName` you defined in the `recoveryConfig` match criteria. Specify 3 to 20 alphanumeric characters or dashes. Ensure that you use the `recoveryConfig` match criteria to apply this option.
*
*/
public Optional monitorStatusCodes1RecoveryConfigName() {
return Optional.ofNullable(this.monitorStatusCodes1RecoveryConfigName);
}
/**
* Enables continuous monitoring for the specific origin status codes that trigger retry or recovery actions.
*
*/
@Import(name="monitorStatusCodes2")
private @Nullable Boolean monitorStatusCodes2;
/**
* @return Enables continuous monitoring for the specific origin status codes that trigger retry or recovery actions.
*
*/
public Optional monitorStatusCodes2() {
return Optional.ofNullable(this.monitorStatusCodes2);
}
/**
* Enables the recovery action for the response codes you define.
*
*/
@Import(name="monitorStatusCodes2EnableRecovery")
private @Nullable Boolean monitorStatusCodes2EnableRecovery;
/**
* @return Enables the recovery action for the response codes you define.
*
*/
public Optional monitorStatusCodes2EnableRecovery() {
return Optional.ofNullable(this.monitorStatusCodes2EnableRecovery);
}
/**
* When the defined response codes apply, attempts a retry on the same origin before executing the recovery method.
*
*/
@Import(name="monitorStatusCodes2EnableRetry")
private @Nullable Boolean monitorStatusCodes2EnableRetry;
/**
* @return When the defined response codes apply, attempts a retry on the same origin before executing the recovery method.
*
*/
public Optional monitorStatusCodes2EnableRetry() {
return Optional.ofNullable(this.monitorStatusCodes2EnableRetry);
}
/**
* Specifies a recovery configuration using the `configName` you defined in the `recoveryConfig` match criteria. Specify 3 to 20 alphanumeric characters or dashes. Ensure that you use the `recoveryConfig` match criteria to apply this option.
*
*/
@Import(name="monitorStatusCodes2RecoveryConfigName")
private @Nullable String monitorStatusCodes2RecoveryConfigName;
/**
* @return Specifies a recovery configuration using the `configName` you defined in the `recoveryConfig` match criteria. Specify 3 to 20 alphanumeric characters or dashes. Ensure that you use the `recoveryConfig` match criteria to apply this option.
*
*/
public Optional monitorStatusCodes2RecoveryConfigName() {
return Optional.ofNullable(this.monitorStatusCodes2RecoveryConfigName);
}
/**
* Enables continuous monitoring for the specific origin status codes that trigger retry or recovery actions.
*
*/
@Import(name="monitorStatusCodes3")
private @Nullable Boolean monitorStatusCodes3;
/**
* @return Enables continuous monitoring for the specific origin status codes that trigger retry or recovery actions.
*
*/
public Optional monitorStatusCodes3() {
return Optional.ofNullable(this.monitorStatusCodes3);
}
/**
* Enables the recovery action for the response codes you define.
*
*/
@Import(name="monitorStatusCodes3EnableRecovery")
private @Nullable Boolean monitorStatusCodes3EnableRecovery;
/**
* @return Enables the recovery action for the response codes you define.
*
*/
public Optional monitorStatusCodes3EnableRecovery() {
return Optional.ofNullable(this.monitorStatusCodes3EnableRecovery);
}
/**
* When the defined response codes apply, attempts a retry on the same origin before executing the recovery method.
*
*/
@Import(name="monitorStatusCodes3EnableRetry")
private @Nullable Boolean monitorStatusCodes3EnableRetry;
/**
* @return When the defined response codes apply, attempts a retry on the same origin before executing the recovery method.
*
*/
public Optional monitorStatusCodes3EnableRetry() {
return Optional.ofNullable(this.monitorStatusCodes3EnableRetry);
}
/**
* Specifies a recovery configuration using the `configName` you defined in the `recoveryConfig` match criteria. Specify 3 to 20 alphanumeric characters or dashes. Ensure that you use the `recoveryConfig` match criteria to apply this option.
*
*/
@Import(name="monitorStatusCodes3RecoveryConfigName")
private @Nullable String monitorStatusCodes3RecoveryConfigName;
/**
* @return Specifies a recovery configuration using the `configName` you defined in the `recoveryConfig` match criteria. Specify 3 to 20 alphanumeric characters or dashes. Ensure that you use the `recoveryConfig` match criteria to apply this option.
*
*/
public Optional monitorStatusCodes3RecoveryConfigName() {
return Optional.ofNullable(this.monitorStatusCodes3RecoveryConfigName);
}
/**
* Specify a custom timeout, from 1 to 10 seconds.
*
*/
@Import(name="originResponsivenessCustomTimeout")
private @Nullable Integer originResponsivenessCustomTimeout;
/**
* @return Specify a custom timeout, from 1 to 10 seconds.
*
*/
public Optional originResponsivenessCustomTimeout() {
return Optional.ofNullable(this.originResponsivenessCustomTimeout);
}
/**
* Enables a recovery action for a specific failure condition.
*
*/
@Import(name="originResponsivenessEnableRecovery")
private @Nullable Boolean originResponsivenessEnableRecovery;
/**
* @return Enables a recovery action for a specific failure condition.
*
*/
public Optional originResponsivenessEnableRecovery() {
return Optional.ofNullable(this.originResponsivenessEnableRecovery);
}
/**
* If a specific failure condition applies, attempts a retry on the same origin before executing the recovery method.
*
*/
@Import(name="originResponsivenessEnableRetry")
private @Nullable Boolean originResponsivenessEnableRetry;
/**
* @return If a specific failure condition applies, attempts a retry on the same origin before executing the recovery method.
*
*/
public Optional originResponsivenessEnableRetry() {
return Optional.ofNullable(this.originResponsivenessEnableRetry);
}
/**
* This field is only intended for export compatibility purposes, and modifying it will not impact your use of the behavior.
*
*/
@Import(name="originResponsivenessMonitoring")
private @Nullable String originResponsivenessMonitoring;
/**
* @return This field is only intended for export compatibility purposes, and modifying it will not impact your use of the behavior.
*
*/
public Optional originResponsivenessMonitoring() {
return Optional.ofNullable(this.originResponsivenessMonitoring);
}
/**
* Specifies a recovery configuration using the `configName` you defined in the `recoveryConfig` match criteria. Specify 3 to 20 alphanumeric characters or dashes. Ensure that you use the `recoveryConfig` match criteria to apply this option.
*
*/
@Import(name="originResponsivenessRecoveryConfigName")
private @Nullable String originResponsivenessRecoveryConfigName;
/**
* @return Specifies a recovery configuration using the `configName` you defined in the `recoveryConfig` match criteria. Specify 3 to 20 alphanumeric characters or dashes. Ensure that you use the `recoveryConfig` match criteria to apply this option.
*
*/
public Optional originResponsivenessRecoveryConfigName() {
return Optional.ofNullable(this.originResponsivenessRecoveryConfigName);
}
/**
* The timeout threshold that triggers a retry or recovery action.
*
*/
@Import(name="originResponsivenessTimeout")
private @Nullable String originResponsivenessTimeout;
/**
* @return The timeout threshold that triggers a retry or recovery action.
*
*/
public Optional originResponsivenessTimeout() {
return Optional.ofNullable(this.originResponsivenessTimeout);
}
/**
* This field is only intended for export compatibility purposes, and modifying it will not impact your use of the behavior.
*
*/
@Import(name="statusCodeMonitoring1")
private @Nullable String statusCodeMonitoring1;
/**
* @return This field is only intended for export compatibility purposes, and modifying it will not impact your use of the behavior.
*
*/
public Optional statusCodeMonitoring1() {
return Optional.ofNullable(this.statusCodeMonitoring1);
}
/**
* This field is only intended for export compatibility purposes, and modifying it will not impact your use of the behavior.
*
*/
@Import(name="statusCodeMonitoring2")
private @Nullable String statusCodeMonitoring2;
/**
* @return This field is only intended for export compatibility purposes, and modifying it will not impact your use of the behavior.
*
*/
public Optional statusCodeMonitoring2() {
return Optional.ofNullable(this.statusCodeMonitoring2);
}
/**
* This field is only intended for export compatibility purposes, and modifying it will not impact your use of the behavior.
*
*/
@Import(name="statusCodeMonitoring3")
private @Nullable String statusCodeMonitoring3;
/**
* @return This field is only intended for export compatibility purposes, and modifying it will not impact your use of the behavior.
*
*/
public Optional statusCodeMonitoring3() {
return Optional.ofNullable(this.statusCodeMonitoring3);
}
/**
* This option is for internal usage only.
*
*/
@Import(name="templateUuid")
private @Nullable String templateUuid;
/**
* @return This option is for internal usage only.
*
*/
public Optional templateUuid() {
return Optional.ofNullable(this.templateUuid);
}
/**
* This field is only intended for export compatibility purposes, and modifying it will not impact your use of the behavior.
*
*/
@Import(name="tuningParameters")
private @Nullable String tuningParameters;
/**
* @return This field is only intended for export compatibility purposes, and modifying it will not impact your use of the behavior.
*
*/
public Optional tuningParameters() {
return Optional.ofNullable(this.tuningParameters);
}
/**
* A uuid member indicates that at least one of its component behaviors or criteria is advanced and read-only. You need to preserve this uuid as well when modifying the rule tree. This option is for internal usage only.
*
*/
@Import(name="uuid")
private @Nullable String uuid;
/**
* @return A uuid member indicates that at least one of its component behaviors or criteria is advanced and read-only. You need to preserve this uuid as well when modifying the rule tree. This option is for internal usage only.
*
*/
public Optional uuid() {
return Optional.ofNullable(this.uuid);
}
private GetPropertyRulesBuilderRulesV20240109BehaviorOriginFailureRecoveryPolicy() {}
private GetPropertyRulesBuilderRulesV20240109BehaviorOriginFailureRecoveryPolicy(GetPropertyRulesBuilderRulesV20240109BehaviorOriginFailureRecoveryPolicy $) {
this.binaryEquivalentContent = $.binaryEquivalentContent;
this.enableIpAvoidance = $.enableIpAvoidance;
this.enabled = $.enabled;
this.ipAvoidanceErrorThreshold = $.ipAvoidanceErrorThreshold;
this.ipAvoidanceRetryInterval = $.ipAvoidanceRetryInterval;
this.locked = $.locked;
this.monitorOriginResponsiveness = $.monitorOriginResponsiveness;
this.monitorResponseCodes1s = $.monitorResponseCodes1s;
this.monitorResponseCodes2s = $.monitorResponseCodes2s;
this.monitorResponseCodes3s = $.monitorResponseCodes3s;
this.monitorStatusCodes1 = $.monitorStatusCodes1;
this.monitorStatusCodes1EnableRecovery = $.monitorStatusCodes1EnableRecovery;
this.monitorStatusCodes1EnableRetry = $.monitorStatusCodes1EnableRetry;
this.monitorStatusCodes1RecoveryConfigName = $.monitorStatusCodes1RecoveryConfigName;
this.monitorStatusCodes2 = $.monitorStatusCodes2;
this.monitorStatusCodes2EnableRecovery = $.monitorStatusCodes2EnableRecovery;
this.monitorStatusCodes2EnableRetry = $.monitorStatusCodes2EnableRetry;
this.monitorStatusCodes2RecoveryConfigName = $.monitorStatusCodes2RecoveryConfigName;
this.monitorStatusCodes3 = $.monitorStatusCodes3;
this.monitorStatusCodes3EnableRecovery = $.monitorStatusCodes3EnableRecovery;
this.monitorStatusCodes3EnableRetry = $.monitorStatusCodes3EnableRetry;
this.monitorStatusCodes3RecoveryConfigName = $.monitorStatusCodes3RecoveryConfigName;
this.originResponsivenessCustomTimeout = $.originResponsivenessCustomTimeout;
this.originResponsivenessEnableRecovery = $.originResponsivenessEnableRecovery;
this.originResponsivenessEnableRetry = $.originResponsivenessEnableRetry;
this.originResponsivenessMonitoring = $.originResponsivenessMonitoring;
this.originResponsivenessRecoveryConfigName = $.originResponsivenessRecoveryConfigName;
this.originResponsivenessTimeout = $.originResponsivenessTimeout;
this.statusCodeMonitoring1 = $.statusCodeMonitoring1;
this.statusCodeMonitoring2 = $.statusCodeMonitoring2;
this.statusCodeMonitoring3 = $.statusCodeMonitoring3;
this.templateUuid = $.templateUuid;
this.tuningParameters = $.tuningParameters;
this.uuid = $.uuid;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetPropertyRulesBuilderRulesV20240109BehaviorOriginFailureRecoveryPolicy defaults) {
return new Builder(defaults);
}
public static final class Builder {
private GetPropertyRulesBuilderRulesV20240109BehaviorOriginFailureRecoveryPolicy $;
public Builder() {
$ = new GetPropertyRulesBuilderRulesV20240109BehaviorOriginFailureRecoveryPolicy();
}
public Builder(GetPropertyRulesBuilderRulesV20240109BehaviorOriginFailureRecoveryPolicy defaults) {
$ = new GetPropertyRulesBuilderRulesV20240109BehaviorOriginFailureRecoveryPolicy(Objects.requireNonNull(defaults));
}
/**
* @param binaryEquivalentContent Synchronizes content between the primary and backup origins, byte for byte.
*
* @return builder
*
*/
public Builder binaryEquivalentContent(@Nullable Boolean binaryEquivalentContent) {
$.binaryEquivalentContent = binaryEquivalentContent;
return this;
}
/**
* @param enableIpAvoidance Temporarily blocks an origin IP address that experienced a certain number of failures. When an IP address is blocked, the `configName` established for `originResponsivenessRecoveryConfigName` is applied.
*
* @return builder
*
*/
public Builder enableIpAvoidance(@Nullable Boolean enableIpAvoidance) {
$.enableIpAvoidance = enableIpAvoidance;
return this;
}
/**
* @param enabled Activates and configures a recovery policy.
*
* @return builder
*
*/
public Builder enabled(@Nullable Boolean enabled) {
$.enabled = enabled;
return this;
}
/**
* @param ipAvoidanceErrorThreshold Defines the number of failures that need to occur to an origin address before it's blocked.
*
* @return builder
*
*/
public Builder ipAvoidanceErrorThreshold(@Nullable Integer ipAvoidanceErrorThreshold) {
$.ipAvoidanceErrorThreshold = ipAvoidanceErrorThreshold;
return this;
}
/**
* @param ipAvoidanceRetryInterval Defines the number of seconds after which the IP address is removed from the blocklist.
*
* @return builder
*
*/
public Builder ipAvoidanceRetryInterval(@Nullable Integer ipAvoidanceRetryInterval) {
$.ipAvoidanceRetryInterval = ipAvoidanceRetryInterval;
return this;
}
/**
* @param locked Indicates that your Akamai representative has locked this behavior or criteria so that you can't modify it. This option is for internal usage only.
*
* @return builder
*
*/
public Builder locked(@Nullable Boolean locked) {
$.locked = locked;
return this;
}
/**
* @param monitorOriginResponsiveness Enables continuous monitoring of connectivity to the origin. If necessary, applies retry or recovery actions.
*
* @return builder
*
*/
public Builder monitorOriginResponsiveness(@Nullable Boolean monitorOriginResponsiveness) {
$.monitorOriginResponsiveness = monitorOriginResponsiveness;
return this;
}
/**
* @param monitorResponseCodes1s Defines the origin response codes that trigger a subsequent retry or recovery action. Specify a single code entry (`501`) or a range (`501:504`). If you configure other `monitorStatusCodes*` and `monitorResponseCodes*` options, you can't use the same codes here.
*
* @return builder
*
*/
public Builder monitorResponseCodes1s(@Nullable List monitorResponseCodes1s) {
$.monitorResponseCodes1s = monitorResponseCodes1s;
return this;
}
/**
* @param monitorResponseCodes1s Defines the origin response codes that trigger a subsequent retry or recovery action. Specify a single code entry (`501`) or a range (`501:504`). If you configure other `monitorStatusCodes*` and `monitorResponseCodes*` options, you can't use the same codes here.
*
* @return builder
*
*/
public Builder monitorResponseCodes1s(String... monitorResponseCodes1s) {
return monitorResponseCodes1s(List.of(monitorResponseCodes1s));
}
/**
* @param monitorResponseCodes2s Defines the origin response codes that trigger a subsequent retry or recovery action. Specify a single code entry (`501`) or a range (`501:504`). If you configure other `monitorStatusCodes*` and `monitorResponseCodes*` options, you can't use the same codes here.
*
* @return builder
*
*/
public Builder monitorResponseCodes2s(@Nullable List monitorResponseCodes2s) {
$.monitorResponseCodes2s = monitorResponseCodes2s;
return this;
}
/**
* @param monitorResponseCodes2s Defines the origin response codes that trigger a subsequent retry or recovery action. Specify a single code entry (`501`) or a range (`501:504`). If you configure other `monitorStatusCodes*` and `monitorResponseCodes*` options, you can't use the same codes here.
*
* @return builder
*
*/
public Builder monitorResponseCodes2s(String... monitorResponseCodes2s) {
return monitorResponseCodes2s(List.of(monitorResponseCodes2s));
}
/**
* @param monitorResponseCodes3s Defines the origin response codes that trigger a subsequent retry or recovery action. Specify a single code entry (`501`) or a range (`501:504`). If you configure other `monitorStatusCodes*` and `monitorResponseCodes*` options, you can't use the same codes here..
*
* @return builder
*
*/
public Builder monitorResponseCodes3s(@Nullable List monitorResponseCodes3s) {
$.monitorResponseCodes3s = monitorResponseCodes3s;
return this;
}
/**
* @param monitorResponseCodes3s Defines the origin response codes that trigger a subsequent retry or recovery action. Specify a single code entry (`501`) or a range (`501:504`). If you configure other `monitorStatusCodes*` and `monitorResponseCodes*` options, you can't use the same codes here..
*
* @return builder
*
*/
public Builder monitorResponseCodes3s(String... monitorResponseCodes3s) {
return monitorResponseCodes3s(List.of(monitorResponseCodes3s));
}
/**
* @param monitorStatusCodes1 Enables continuous monitoring for the specific origin status codes that trigger retry or recovery actions.
*
* @return builder
*
*/
public Builder monitorStatusCodes1(@Nullable Boolean monitorStatusCodes1) {
$.monitorStatusCodes1 = monitorStatusCodes1;
return this;
}
/**
* @param monitorStatusCodes1EnableRecovery Enables the recovery action for the response codes you define.
*
* @return builder
*
*/
public Builder monitorStatusCodes1EnableRecovery(@Nullable Boolean monitorStatusCodes1EnableRecovery) {
$.monitorStatusCodes1EnableRecovery = monitorStatusCodes1EnableRecovery;
return this;
}
/**
* @param monitorStatusCodes1EnableRetry When the defined response codes apply, attempts a retry on the same origin before executing the recovery method.
*
* @return builder
*
*/
public Builder monitorStatusCodes1EnableRetry(@Nullable Boolean monitorStatusCodes1EnableRetry) {
$.monitorStatusCodes1EnableRetry = monitorStatusCodes1EnableRetry;
return this;
}
/**
* @param monitorStatusCodes1RecoveryConfigName Specifies a recovery configuration using the `configName` you defined in the `recoveryConfig` match criteria. Specify 3 to 20 alphanumeric characters or dashes. Ensure that you use the `recoveryConfig` match criteria to apply this option.
*
* @return builder
*
*/
public Builder monitorStatusCodes1RecoveryConfigName(@Nullable String monitorStatusCodes1RecoveryConfigName) {
$.monitorStatusCodes1RecoveryConfigName = monitorStatusCodes1RecoveryConfigName;
return this;
}
/**
* @param monitorStatusCodes2 Enables continuous monitoring for the specific origin status codes that trigger retry or recovery actions.
*
* @return builder
*
*/
public Builder monitorStatusCodes2(@Nullable Boolean monitorStatusCodes2) {
$.monitorStatusCodes2 = monitorStatusCodes2;
return this;
}
/**
* @param monitorStatusCodes2EnableRecovery Enables the recovery action for the response codes you define.
*
* @return builder
*
*/
public Builder monitorStatusCodes2EnableRecovery(@Nullable Boolean monitorStatusCodes2EnableRecovery) {
$.monitorStatusCodes2EnableRecovery = monitorStatusCodes2EnableRecovery;
return this;
}
/**
* @param monitorStatusCodes2EnableRetry When the defined response codes apply, attempts a retry on the same origin before executing the recovery method.
*
* @return builder
*
*/
public Builder monitorStatusCodes2EnableRetry(@Nullable Boolean monitorStatusCodes2EnableRetry) {
$.monitorStatusCodes2EnableRetry = monitorStatusCodes2EnableRetry;
return this;
}
/**
* @param monitorStatusCodes2RecoveryConfigName Specifies a recovery configuration using the `configName` you defined in the `recoveryConfig` match criteria. Specify 3 to 20 alphanumeric characters or dashes. Ensure that you use the `recoveryConfig` match criteria to apply this option.
*
* @return builder
*
*/
public Builder monitorStatusCodes2RecoveryConfigName(@Nullable String monitorStatusCodes2RecoveryConfigName) {
$.monitorStatusCodes2RecoveryConfigName = monitorStatusCodes2RecoveryConfigName;
return this;
}
/**
* @param monitorStatusCodes3 Enables continuous monitoring for the specific origin status codes that trigger retry or recovery actions.
*
* @return builder
*
*/
public Builder monitorStatusCodes3(@Nullable Boolean monitorStatusCodes3) {
$.monitorStatusCodes3 = monitorStatusCodes3;
return this;
}
/**
* @param monitorStatusCodes3EnableRecovery Enables the recovery action for the response codes you define.
*
* @return builder
*
*/
public Builder monitorStatusCodes3EnableRecovery(@Nullable Boolean monitorStatusCodes3EnableRecovery) {
$.monitorStatusCodes3EnableRecovery = monitorStatusCodes3EnableRecovery;
return this;
}
/**
* @param monitorStatusCodes3EnableRetry When the defined response codes apply, attempts a retry on the same origin before executing the recovery method.
*
* @return builder
*
*/
public Builder monitorStatusCodes3EnableRetry(@Nullable Boolean monitorStatusCodes3EnableRetry) {
$.monitorStatusCodes3EnableRetry = monitorStatusCodes3EnableRetry;
return this;
}
/**
* @param monitorStatusCodes3RecoveryConfigName Specifies a recovery configuration using the `configName` you defined in the `recoveryConfig` match criteria. Specify 3 to 20 alphanumeric characters or dashes. Ensure that you use the `recoveryConfig` match criteria to apply this option.
*
* @return builder
*
*/
public Builder monitorStatusCodes3RecoveryConfigName(@Nullable String monitorStatusCodes3RecoveryConfigName) {
$.monitorStatusCodes3RecoveryConfigName = monitorStatusCodes3RecoveryConfigName;
return this;
}
/**
* @param originResponsivenessCustomTimeout Specify a custom timeout, from 1 to 10 seconds.
*
* @return builder
*
*/
public Builder originResponsivenessCustomTimeout(@Nullable Integer originResponsivenessCustomTimeout) {
$.originResponsivenessCustomTimeout = originResponsivenessCustomTimeout;
return this;
}
/**
* @param originResponsivenessEnableRecovery Enables a recovery action for a specific failure condition.
*
* @return builder
*
*/
public Builder originResponsivenessEnableRecovery(@Nullable Boolean originResponsivenessEnableRecovery) {
$.originResponsivenessEnableRecovery = originResponsivenessEnableRecovery;
return this;
}
/**
* @param originResponsivenessEnableRetry If a specific failure condition applies, attempts a retry on the same origin before executing the recovery method.
*
* @return builder
*
*/
public Builder originResponsivenessEnableRetry(@Nullable Boolean originResponsivenessEnableRetry) {
$.originResponsivenessEnableRetry = originResponsivenessEnableRetry;
return this;
}
/**
* @param originResponsivenessMonitoring This field is only intended for export compatibility purposes, and modifying it will not impact your use of the behavior.
*
* @return builder
*
*/
public Builder originResponsivenessMonitoring(@Nullable String originResponsivenessMonitoring) {
$.originResponsivenessMonitoring = originResponsivenessMonitoring;
return this;
}
/**
* @param originResponsivenessRecoveryConfigName Specifies a recovery configuration using the `configName` you defined in the `recoveryConfig` match criteria. Specify 3 to 20 alphanumeric characters or dashes. Ensure that you use the `recoveryConfig` match criteria to apply this option.
*
* @return builder
*
*/
public Builder originResponsivenessRecoveryConfigName(@Nullable String originResponsivenessRecoveryConfigName) {
$.originResponsivenessRecoveryConfigName = originResponsivenessRecoveryConfigName;
return this;
}
/**
* @param originResponsivenessTimeout The timeout threshold that triggers a retry or recovery action.
*
* @return builder
*
*/
public Builder originResponsivenessTimeout(@Nullable String originResponsivenessTimeout) {
$.originResponsivenessTimeout = originResponsivenessTimeout;
return this;
}
/**
* @param statusCodeMonitoring1 This field is only intended for export compatibility purposes, and modifying it will not impact your use of the behavior.
*
* @return builder
*
*/
public Builder statusCodeMonitoring1(@Nullable String statusCodeMonitoring1) {
$.statusCodeMonitoring1 = statusCodeMonitoring1;
return this;
}
/**
* @param statusCodeMonitoring2 This field is only intended for export compatibility purposes, and modifying it will not impact your use of the behavior.
*
* @return builder
*
*/
public Builder statusCodeMonitoring2(@Nullable String statusCodeMonitoring2) {
$.statusCodeMonitoring2 = statusCodeMonitoring2;
return this;
}
/**
* @param statusCodeMonitoring3 This field is only intended for export compatibility purposes, and modifying it will not impact your use of the behavior.
*
* @return builder
*
*/
public Builder statusCodeMonitoring3(@Nullable String statusCodeMonitoring3) {
$.statusCodeMonitoring3 = statusCodeMonitoring3;
return this;
}
/**
* @param templateUuid This option is for internal usage only.
*
* @return builder
*
*/
public Builder templateUuid(@Nullable String templateUuid) {
$.templateUuid = templateUuid;
return this;
}
/**
* @param tuningParameters This field is only intended for export compatibility purposes, and modifying it will not impact your use of the behavior.
*
* @return builder
*
*/
public Builder tuningParameters(@Nullable String tuningParameters) {
$.tuningParameters = tuningParameters;
return this;
}
/**
* @param uuid A uuid member indicates that at least one of its component behaviors or criteria is advanced and read-only. You need to preserve this uuid as well when modifying the rule tree. This option is for internal usage only.
*
* @return builder
*
*/
public Builder uuid(@Nullable String uuid) {
$.uuid = uuid;
return this;
}
public GetPropertyRulesBuilderRulesV20240109BehaviorOriginFailureRecoveryPolicy build() {
return $;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy