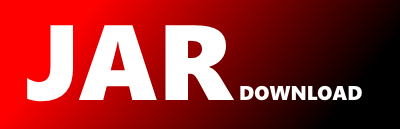
com.pulumi.akamai.outputs.GetImagingPolicyImagePolicyPostBreakpointTransformationCompositeImageTextImage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of akamai Show documentation
Show all versions of akamai Show documentation
A Pulumi package for creating and managing akamai cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.akamai.outputs;
import com.pulumi.akamai.outputs.GetImagingPolicyImagePolicyPostBreakpointTransformation;
import com.pulumi.core.annotations.CustomType;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetImagingPolicyImagePolicyPostBreakpointTransformationCompositeImageTextImage {
/**
* @return The main fill color of the text.
*
*/
private @Nullable String fill;
/**
* @return The main fill color of the text.
*
*/
private @Nullable String fillVar;
/**
* @return The size in pixels to render the text.
*
*/
private @Nullable String size;
/**
* @return The size in pixels to render the text.
*
*/
private @Nullable String sizeVar;
/**
* @return The color for the outline of the text.
*
*/
private @Nullable String stroke;
/**
* @return The thickness in points for the outline of the text.
*
*/
private @Nullable String strokeSize;
/**
* @return The thickness in points for the outline of the text.
*
*/
private @Nullable String strokeSizeVar;
/**
* @return The color for the outline of the text.
*
*/
private @Nullable String strokeVar;
/**
* @return The line of text to render.
*
*/
private @Nullable String text;
/**
* @return The line of text to render.
*
*/
private @Nullable String textVar;
private @Nullable GetImagingPolicyImagePolicyPostBreakpointTransformation transformation;
/**
* @return The font family to apply to the text image. This may be a URL to a TrueType or WOFF (v1) typeface, or a string that refers to one of the standard built-in browser fonts.
*
*/
private @Nullable String typeface;
/**
* @return The font family to apply to the text image. This may be a URL to a TrueType or WOFF (v1) typeface, or a string that refers to one of the standard built-in browser fonts.
*
*/
private @Nullable String typefaceVar;
private GetImagingPolicyImagePolicyPostBreakpointTransformationCompositeImageTextImage() {}
/**
* @return The main fill color of the text.
*
*/
public Optional fill() {
return Optional.ofNullable(this.fill);
}
/**
* @return The main fill color of the text.
*
*/
public Optional fillVar() {
return Optional.ofNullable(this.fillVar);
}
/**
* @return The size in pixels to render the text.
*
*/
public Optional size() {
return Optional.ofNullable(this.size);
}
/**
* @return The size in pixels to render the text.
*
*/
public Optional sizeVar() {
return Optional.ofNullable(this.sizeVar);
}
/**
* @return The color for the outline of the text.
*
*/
public Optional stroke() {
return Optional.ofNullable(this.stroke);
}
/**
* @return The thickness in points for the outline of the text.
*
*/
public Optional strokeSize() {
return Optional.ofNullable(this.strokeSize);
}
/**
* @return The thickness in points for the outline of the text.
*
*/
public Optional strokeSizeVar() {
return Optional.ofNullable(this.strokeSizeVar);
}
/**
* @return The color for the outline of the text.
*
*/
public Optional strokeVar() {
return Optional.ofNullable(this.strokeVar);
}
/**
* @return The line of text to render.
*
*/
public Optional text() {
return Optional.ofNullable(this.text);
}
/**
* @return The line of text to render.
*
*/
public Optional textVar() {
return Optional.ofNullable(this.textVar);
}
public Optional transformation() {
return Optional.ofNullable(this.transformation);
}
/**
* @return The font family to apply to the text image. This may be a URL to a TrueType or WOFF (v1) typeface, or a string that refers to one of the standard built-in browser fonts.
*
*/
public Optional typeface() {
return Optional.ofNullable(this.typeface);
}
/**
* @return The font family to apply to the text image. This may be a URL to a TrueType or WOFF (v1) typeface, or a string that refers to one of the standard built-in browser fonts.
*
*/
public Optional typefaceVar() {
return Optional.ofNullable(this.typefaceVar);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetImagingPolicyImagePolicyPostBreakpointTransformationCompositeImageTextImage defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String fill;
private @Nullable String fillVar;
private @Nullable String size;
private @Nullable String sizeVar;
private @Nullable String stroke;
private @Nullable String strokeSize;
private @Nullable String strokeSizeVar;
private @Nullable String strokeVar;
private @Nullable String text;
private @Nullable String textVar;
private @Nullable GetImagingPolicyImagePolicyPostBreakpointTransformation transformation;
private @Nullable String typeface;
private @Nullable String typefaceVar;
public Builder() {}
public Builder(GetImagingPolicyImagePolicyPostBreakpointTransformationCompositeImageTextImage defaults) {
Objects.requireNonNull(defaults);
this.fill = defaults.fill;
this.fillVar = defaults.fillVar;
this.size = defaults.size;
this.sizeVar = defaults.sizeVar;
this.stroke = defaults.stroke;
this.strokeSize = defaults.strokeSize;
this.strokeSizeVar = defaults.strokeSizeVar;
this.strokeVar = defaults.strokeVar;
this.text = defaults.text;
this.textVar = defaults.textVar;
this.transformation = defaults.transformation;
this.typeface = defaults.typeface;
this.typefaceVar = defaults.typefaceVar;
}
@CustomType.Setter
public Builder fill(@Nullable String fill) {
this.fill = fill;
return this;
}
@CustomType.Setter
public Builder fillVar(@Nullable String fillVar) {
this.fillVar = fillVar;
return this;
}
@CustomType.Setter
public Builder size(@Nullable String size) {
this.size = size;
return this;
}
@CustomType.Setter
public Builder sizeVar(@Nullable String sizeVar) {
this.sizeVar = sizeVar;
return this;
}
@CustomType.Setter
public Builder stroke(@Nullable String stroke) {
this.stroke = stroke;
return this;
}
@CustomType.Setter
public Builder strokeSize(@Nullable String strokeSize) {
this.strokeSize = strokeSize;
return this;
}
@CustomType.Setter
public Builder strokeSizeVar(@Nullable String strokeSizeVar) {
this.strokeSizeVar = strokeSizeVar;
return this;
}
@CustomType.Setter
public Builder strokeVar(@Nullable String strokeVar) {
this.strokeVar = strokeVar;
return this;
}
@CustomType.Setter
public Builder text(@Nullable String text) {
this.text = text;
return this;
}
@CustomType.Setter
public Builder textVar(@Nullable String textVar) {
this.textVar = textVar;
return this;
}
@CustomType.Setter
public Builder transformation(@Nullable GetImagingPolicyImagePolicyPostBreakpointTransformation transformation) {
this.transformation = transformation;
return this;
}
@CustomType.Setter
public Builder typeface(@Nullable String typeface) {
this.typeface = typeface;
return this;
}
@CustomType.Setter
public Builder typefaceVar(@Nullable String typefaceVar) {
this.typefaceVar = typefaceVar;
return this;
}
public GetImagingPolicyImagePolicyPostBreakpointTransformationCompositeImageTextImage build() {
final var _resultValue = new GetImagingPolicyImagePolicyPostBreakpointTransformationCompositeImageTextImage();
_resultValue.fill = fill;
_resultValue.fillVar = fillVar;
_resultValue.size = size;
_resultValue.sizeVar = sizeVar;
_resultValue.stroke = stroke;
_resultValue.strokeSize = strokeSize;
_resultValue.strokeSizeVar = strokeSizeVar;
_resultValue.strokeVar = strokeVar;
_resultValue.text = text;
_resultValue.textVar = textVar;
_resultValue.transformation = transformation;
_resultValue.typeface = typeface;
_resultValue.typefaceVar = typefaceVar;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy