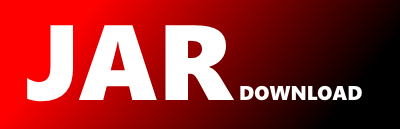
com.pulumi.akamai.outputs.GetPropertyRulesBuilderRulesV20230920BehaviorVisitorPrioritizationFifo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of akamai Show documentation
Show all versions of akamai Show documentation
A Pulumi package for creating and managing akamai cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.akamai.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetPropertyRulesBuilderRulesV20230920BehaviorVisitorPrioritizationFifo {
/**
* @return This field is only intended for export compatibility purposes, and modifying it will not impact your use of the behavior.
*
*/
private @Nullable String accessTitle;
/**
* @return This identifies the Visitor Prioritization FIFO shared policy to use with this behavior. You can list available shared policies with the `Cloudlets API`.
*
*/
private @Nullable Integer cloudletSharedPolicy;
/**
* @return This specifies a domain for all session cookies. In case you configure many property hostnames, this may be their common domain. Make sure the user agent accepts the custom domain for any request matching the `visitorPrioritizationFifo` behavior. Don't use top level domains (TLDs).
*
*/
private @Nullable String customCookieDomain;
/**
* @return This specifies how to set the domain used to establish a session with the visitor.
*
*/
private @Nullable String domainConfig;
/**
* @return Indicates that your Akamai representative has locked this behavior or criteria so that you can't modify it. This option is for internal usage only.
*
*/
private @Nullable Boolean locked;
/**
* @return Whether the queue session should prolong automatically when the `sessionDuration` expires and the visitor remains active.
*
*/
private @Nullable Boolean sessionAutoProlong;
/**
* @return Specifies the number of seconds users remain in the waiting room queue.
*
*/
private @Nullable Integer sessionDuration;
/**
* @return This option is for internal usage only.
*
*/
private @Nullable String templateUuid;
/**
* @return A uuid member indicates that at least one of its component behaviors or criteria is advanced and read-only. You need to preserve this uuid as well when modifying the rule tree. This option is for internal usage only.
*
*/
private @Nullable String uuid;
/**
* @return This specifies the base paths to static resources such as `JavaScript`, `CSS`, or image files for the `Waiting Room Main Page` requests. The option supports the `*` wildcard wildcard that matches zero or more characters. Requests matching any of these paths aren't blocked, but marked as Waiting Room Assets and passed through to the origin. See the `visitorPrioritizationRequest` match criteria to further customize these requests.
*
*/
private @Nullable List waitingRoomAssetsPaths;
/**
* @return This specifies the path to the waiting room main page on the origin server, for example `/vp/waiting-room.html`. When the request is marked as `Waiting Room Main Page` and blocked, the visitor enters the waiting room. The behavior sets the outgoing request path to the `waitingRoomPath` and modifies the cache key accordingly. See the `visitorPrioritizationRequest` match criteria to further customize these requests.
*
*/
private @Nullable String waitingRoomPath;
/**
* @return This field is only intended for export compatibility purposes, and modifying it will not impact your use of the behavior.
*
*/
private @Nullable String waitingRoomTitle;
private GetPropertyRulesBuilderRulesV20230920BehaviorVisitorPrioritizationFifo() {}
/**
* @return This field is only intended for export compatibility purposes, and modifying it will not impact your use of the behavior.
*
*/
public Optional accessTitle() {
return Optional.ofNullable(this.accessTitle);
}
/**
* @return This identifies the Visitor Prioritization FIFO shared policy to use with this behavior. You can list available shared policies with the `Cloudlets API`.
*
*/
public Optional cloudletSharedPolicy() {
return Optional.ofNullable(this.cloudletSharedPolicy);
}
/**
* @return This specifies a domain for all session cookies. In case you configure many property hostnames, this may be their common domain. Make sure the user agent accepts the custom domain for any request matching the `visitorPrioritizationFifo` behavior. Don't use top level domains (TLDs).
*
*/
public Optional customCookieDomain() {
return Optional.ofNullable(this.customCookieDomain);
}
/**
* @return This specifies how to set the domain used to establish a session with the visitor.
*
*/
public Optional domainConfig() {
return Optional.ofNullable(this.domainConfig);
}
/**
* @return Indicates that your Akamai representative has locked this behavior or criteria so that you can't modify it. This option is for internal usage only.
*
*/
public Optional locked() {
return Optional.ofNullable(this.locked);
}
/**
* @return Whether the queue session should prolong automatically when the `sessionDuration` expires and the visitor remains active.
*
*/
public Optional sessionAutoProlong() {
return Optional.ofNullable(this.sessionAutoProlong);
}
/**
* @return Specifies the number of seconds users remain in the waiting room queue.
*
*/
public Optional sessionDuration() {
return Optional.ofNullable(this.sessionDuration);
}
/**
* @return This option is for internal usage only.
*
*/
public Optional templateUuid() {
return Optional.ofNullable(this.templateUuid);
}
/**
* @return A uuid member indicates that at least one of its component behaviors or criteria is advanced and read-only. You need to preserve this uuid as well when modifying the rule tree. This option is for internal usage only.
*
*/
public Optional uuid() {
return Optional.ofNullable(this.uuid);
}
/**
* @return This specifies the base paths to static resources such as `JavaScript`, `CSS`, or image files for the `Waiting Room Main Page` requests. The option supports the `*` wildcard wildcard that matches zero or more characters. Requests matching any of these paths aren't blocked, but marked as Waiting Room Assets and passed through to the origin. See the `visitorPrioritizationRequest` match criteria to further customize these requests.
*
*/
public List waitingRoomAssetsPaths() {
return this.waitingRoomAssetsPaths == null ? List.of() : this.waitingRoomAssetsPaths;
}
/**
* @return This specifies the path to the waiting room main page on the origin server, for example `/vp/waiting-room.html`. When the request is marked as `Waiting Room Main Page` and blocked, the visitor enters the waiting room. The behavior sets the outgoing request path to the `waitingRoomPath` and modifies the cache key accordingly. See the `visitorPrioritizationRequest` match criteria to further customize these requests.
*
*/
public Optional waitingRoomPath() {
return Optional.ofNullable(this.waitingRoomPath);
}
/**
* @return This field is only intended for export compatibility purposes, and modifying it will not impact your use of the behavior.
*
*/
public Optional waitingRoomTitle() {
return Optional.ofNullable(this.waitingRoomTitle);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetPropertyRulesBuilderRulesV20230920BehaviorVisitorPrioritizationFifo defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String accessTitle;
private @Nullable Integer cloudletSharedPolicy;
private @Nullable String customCookieDomain;
private @Nullable String domainConfig;
private @Nullable Boolean locked;
private @Nullable Boolean sessionAutoProlong;
private @Nullable Integer sessionDuration;
private @Nullable String templateUuid;
private @Nullable String uuid;
private @Nullable List waitingRoomAssetsPaths;
private @Nullable String waitingRoomPath;
private @Nullable String waitingRoomTitle;
public Builder() {}
public Builder(GetPropertyRulesBuilderRulesV20230920BehaviorVisitorPrioritizationFifo defaults) {
Objects.requireNonNull(defaults);
this.accessTitle = defaults.accessTitle;
this.cloudletSharedPolicy = defaults.cloudletSharedPolicy;
this.customCookieDomain = defaults.customCookieDomain;
this.domainConfig = defaults.domainConfig;
this.locked = defaults.locked;
this.sessionAutoProlong = defaults.sessionAutoProlong;
this.sessionDuration = defaults.sessionDuration;
this.templateUuid = defaults.templateUuid;
this.uuid = defaults.uuid;
this.waitingRoomAssetsPaths = defaults.waitingRoomAssetsPaths;
this.waitingRoomPath = defaults.waitingRoomPath;
this.waitingRoomTitle = defaults.waitingRoomTitle;
}
@CustomType.Setter
public Builder accessTitle(@Nullable String accessTitle) {
this.accessTitle = accessTitle;
return this;
}
@CustomType.Setter
public Builder cloudletSharedPolicy(@Nullable Integer cloudletSharedPolicy) {
this.cloudletSharedPolicy = cloudletSharedPolicy;
return this;
}
@CustomType.Setter
public Builder customCookieDomain(@Nullable String customCookieDomain) {
this.customCookieDomain = customCookieDomain;
return this;
}
@CustomType.Setter
public Builder domainConfig(@Nullable String domainConfig) {
this.domainConfig = domainConfig;
return this;
}
@CustomType.Setter
public Builder locked(@Nullable Boolean locked) {
this.locked = locked;
return this;
}
@CustomType.Setter
public Builder sessionAutoProlong(@Nullable Boolean sessionAutoProlong) {
this.sessionAutoProlong = sessionAutoProlong;
return this;
}
@CustomType.Setter
public Builder sessionDuration(@Nullable Integer sessionDuration) {
this.sessionDuration = sessionDuration;
return this;
}
@CustomType.Setter
public Builder templateUuid(@Nullable String templateUuid) {
this.templateUuid = templateUuid;
return this;
}
@CustomType.Setter
public Builder uuid(@Nullable String uuid) {
this.uuid = uuid;
return this;
}
@CustomType.Setter
public Builder waitingRoomAssetsPaths(@Nullable List waitingRoomAssetsPaths) {
this.waitingRoomAssetsPaths = waitingRoomAssetsPaths;
return this;
}
public Builder waitingRoomAssetsPaths(String... waitingRoomAssetsPaths) {
return waitingRoomAssetsPaths(List.of(waitingRoomAssetsPaths));
}
@CustomType.Setter
public Builder waitingRoomPath(@Nullable String waitingRoomPath) {
this.waitingRoomPath = waitingRoomPath;
return this;
}
@CustomType.Setter
public Builder waitingRoomTitle(@Nullable String waitingRoomTitle) {
this.waitingRoomTitle = waitingRoomTitle;
return this;
}
public GetPropertyRulesBuilderRulesV20230920BehaviorVisitorPrioritizationFifo build() {
final var _resultValue = new GetPropertyRulesBuilderRulesV20230920BehaviorVisitorPrioritizationFifo();
_resultValue.accessTitle = accessTitle;
_resultValue.cloudletSharedPolicy = cloudletSharedPolicy;
_resultValue.customCookieDomain = customCookieDomain;
_resultValue.domainConfig = domainConfig;
_resultValue.locked = locked;
_resultValue.sessionAutoProlong = sessionAutoProlong;
_resultValue.sessionDuration = sessionDuration;
_resultValue.templateUuid = templateUuid;
_resultValue.uuid = uuid;
_resultValue.waitingRoomAssetsPaths = waitingRoomAssetsPaths;
_resultValue.waitingRoomPath = waitingRoomPath;
_resultValue.waitingRoomTitle = waitingRoomTitle;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy