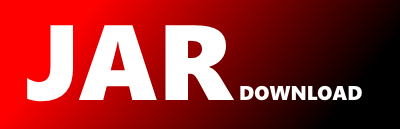
com.pulumi.akamai.outputs.GetPropertyRulesBuilderRulesV20230920CriterionRequestCookie Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of akamai Show documentation
Show all versions of akamai Show documentation
A Pulumi package for creating and managing akamai cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.akamai.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetPropertyRulesBuilderRulesV20230920CriterionRequestCookie {
/**
* @return The name of the cookie, for example, `visitor` in `visitor:anon`.
*
*/
private @Nullable String cookieName;
/**
* @return Indicates that your Akamai representative has locked this behavior or criteria so that you can't modify it. This option is for internal usage only.
*
*/
private @Nullable Boolean locked;
/**
* @return When the `value` is numeric, this field specifies the match's minimum value.
*
*/
private @Nullable Integer lowerBound;
/**
* @return Sets a case-sensitive match for the `cookieName` field.
*
*/
private @Nullable Boolean matchCaseSensitiveName;
/**
* @return Sets a case-sensitive match for the `value` field.
*
*/
private @Nullable Boolean matchCaseSensitiveValue;
/**
* @return Narrows the match criteria.
*
*/
private @Nullable String matchOperator;
/**
* @return Allows wildcards in the `cookieName` field, where `?` matches a single character and `*` matches zero or more characters.
*
*/
private @Nullable Boolean matchWildcardName;
/**
* @return Allows wildcards in the `value` field, where `?` matches a single character and `*` matches zero or more characters.
*
*/
private @Nullable Boolean matchWildcardValue;
/**
* @return This option is for internal usage only.
*
*/
private @Nullable String templateUuid;
/**
* @return When the `value` is numeric, this field specifies the match's maximum value.
*
*/
private @Nullable Integer upperBound;
/**
* @return A uuid member indicates that at least one of its component behaviors or criteria is advanced and read-only. You need to preserve this uuid as well when modifying the rule tree. This option is for internal usage only.
*
*/
private @Nullable String uuid;
/**
* @return The cookie's value, for example, `anon` in `visitor:anon`.
*
*/
private @Nullable String value;
private GetPropertyRulesBuilderRulesV20230920CriterionRequestCookie() {}
/**
* @return The name of the cookie, for example, `visitor` in `visitor:anon`.
*
*/
public Optional cookieName() {
return Optional.ofNullable(this.cookieName);
}
/**
* @return Indicates that your Akamai representative has locked this behavior or criteria so that you can't modify it. This option is for internal usage only.
*
*/
public Optional locked() {
return Optional.ofNullable(this.locked);
}
/**
* @return When the `value` is numeric, this field specifies the match's minimum value.
*
*/
public Optional lowerBound() {
return Optional.ofNullable(this.lowerBound);
}
/**
* @return Sets a case-sensitive match for the `cookieName` field.
*
*/
public Optional matchCaseSensitiveName() {
return Optional.ofNullable(this.matchCaseSensitiveName);
}
/**
* @return Sets a case-sensitive match for the `value` field.
*
*/
public Optional matchCaseSensitiveValue() {
return Optional.ofNullable(this.matchCaseSensitiveValue);
}
/**
* @return Narrows the match criteria.
*
*/
public Optional matchOperator() {
return Optional.ofNullable(this.matchOperator);
}
/**
* @return Allows wildcards in the `cookieName` field, where `?` matches a single character and `*` matches zero or more characters.
*
*/
public Optional matchWildcardName() {
return Optional.ofNullable(this.matchWildcardName);
}
/**
* @return Allows wildcards in the `value` field, where `?` matches a single character and `*` matches zero or more characters.
*
*/
public Optional matchWildcardValue() {
return Optional.ofNullable(this.matchWildcardValue);
}
/**
* @return This option is for internal usage only.
*
*/
public Optional templateUuid() {
return Optional.ofNullable(this.templateUuid);
}
/**
* @return When the `value` is numeric, this field specifies the match's maximum value.
*
*/
public Optional upperBound() {
return Optional.ofNullable(this.upperBound);
}
/**
* @return A uuid member indicates that at least one of its component behaviors or criteria is advanced and read-only. You need to preserve this uuid as well when modifying the rule tree. This option is for internal usage only.
*
*/
public Optional uuid() {
return Optional.ofNullable(this.uuid);
}
/**
* @return The cookie's value, for example, `anon` in `visitor:anon`.
*
*/
public Optional value() {
return Optional.ofNullable(this.value);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetPropertyRulesBuilderRulesV20230920CriterionRequestCookie defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String cookieName;
private @Nullable Boolean locked;
private @Nullable Integer lowerBound;
private @Nullable Boolean matchCaseSensitiveName;
private @Nullable Boolean matchCaseSensitiveValue;
private @Nullable String matchOperator;
private @Nullable Boolean matchWildcardName;
private @Nullable Boolean matchWildcardValue;
private @Nullable String templateUuid;
private @Nullable Integer upperBound;
private @Nullable String uuid;
private @Nullable String value;
public Builder() {}
public Builder(GetPropertyRulesBuilderRulesV20230920CriterionRequestCookie defaults) {
Objects.requireNonNull(defaults);
this.cookieName = defaults.cookieName;
this.locked = defaults.locked;
this.lowerBound = defaults.lowerBound;
this.matchCaseSensitiveName = defaults.matchCaseSensitiveName;
this.matchCaseSensitiveValue = defaults.matchCaseSensitiveValue;
this.matchOperator = defaults.matchOperator;
this.matchWildcardName = defaults.matchWildcardName;
this.matchWildcardValue = defaults.matchWildcardValue;
this.templateUuid = defaults.templateUuid;
this.upperBound = defaults.upperBound;
this.uuid = defaults.uuid;
this.value = defaults.value;
}
@CustomType.Setter
public Builder cookieName(@Nullable String cookieName) {
this.cookieName = cookieName;
return this;
}
@CustomType.Setter
public Builder locked(@Nullable Boolean locked) {
this.locked = locked;
return this;
}
@CustomType.Setter
public Builder lowerBound(@Nullable Integer lowerBound) {
this.lowerBound = lowerBound;
return this;
}
@CustomType.Setter
public Builder matchCaseSensitiveName(@Nullable Boolean matchCaseSensitiveName) {
this.matchCaseSensitiveName = matchCaseSensitiveName;
return this;
}
@CustomType.Setter
public Builder matchCaseSensitiveValue(@Nullable Boolean matchCaseSensitiveValue) {
this.matchCaseSensitiveValue = matchCaseSensitiveValue;
return this;
}
@CustomType.Setter
public Builder matchOperator(@Nullable String matchOperator) {
this.matchOperator = matchOperator;
return this;
}
@CustomType.Setter
public Builder matchWildcardName(@Nullable Boolean matchWildcardName) {
this.matchWildcardName = matchWildcardName;
return this;
}
@CustomType.Setter
public Builder matchWildcardValue(@Nullable Boolean matchWildcardValue) {
this.matchWildcardValue = matchWildcardValue;
return this;
}
@CustomType.Setter
public Builder templateUuid(@Nullable String templateUuid) {
this.templateUuid = templateUuid;
return this;
}
@CustomType.Setter
public Builder upperBound(@Nullable Integer upperBound) {
this.upperBound = upperBound;
return this;
}
@CustomType.Setter
public Builder uuid(@Nullable String uuid) {
this.uuid = uuid;
return this;
}
@CustomType.Setter
public Builder value(@Nullable String value) {
this.value = value;
return this;
}
public GetPropertyRulesBuilderRulesV20230920CriterionRequestCookie build() {
final var _resultValue = new GetPropertyRulesBuilderRulesV20230920CriterionRequestCookie();
_resultValue.cookieName = cookieName;
_resultValue.locked = locked;
_resultValue.lowerBound = lowerBound;
_resultValue.matchCaseSensitiveName = matchCaseSensitiveName;
_resultValue.matchCaseSensitiveValue = matchCaseSensitiveValue;
_resultValue.matchOperator = matchOperator;
_resultValue.matchWildcardName = matchWildcardName;
_resultValue.matchWildcardValue = matchWildcardValue;
_resultValue.templateUuid = templateUuid;
_resultValue.upperBound = upperBound;
_resultValue.uuid = uuid;
_resultValue.value = value;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy