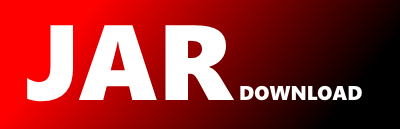
com.pulumi.akamai.outputs.GetPropertyRulesBuilderRulesV20240212BehaviorSaasDefinitions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of akamai Show documentation
Show all versions of akamai Show documentation
A Pulumi package for creating and managing akamai cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.akamai.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetPropertyRulesBuilderRulesV20240212BehaviorSaasDefinitions {
/**
* @return Specifies the request component that identifies a SaaS application.
*
*/
private @Nullable String applicationAction;
/**
* @return Enabling this allows you to identify applications using a `CNAME chain` rather than a single hostname.
*
*/
private @Nullable Boolean applicationCnameEnabled;
/**
* @return Specifies the number of CNAMEs to use in the chain.
*
*/
private @Nullable Integer applicationCnameLevel;
/**
* @return This specifies the name of the cookie that identifies the application.
*
*/
private @Nullable String applicationCookie;
/**
* @return This names the query parameter that identifies the application.
*
*/
private @Nullable String applicationQueryString;
/**
* @return Specifies a Perl-compatible regular expression with which to substitute the request's application ID.
*
*/
private @Nullable String applicationRegex;
/**
* @return Specifies a string to replace the request's application ID matched by `applicationRegex`.
*
*/
private @Nullable String applicationReplace;
/**
* @return This field is only intended for export compatibility purposes, and modifying it will not impact your use of the behavior.
*
*/
private @Nullable String applicationTitle;
/**
* @return Specifies the request component that identifies a SaaS customer.
*
*/
private @Nullable String customerAction;
/**
* @return Enabling this allows you to identify customers using a `CNAME chain` rather than a single hostname.
*
*/
private @Nullable Boolean customerCnameEnabled;
/**
* @return Specifies the number of CNAMEs to use in the chain.
*
*/
private @Nullable Integer customerCnameLevel;
/**
* @return This specifies the name of the cookie that identifies the customer.
*
*/
private @Nullable String customerCookie;
/**
* @return This names the query parameter that identifies the customer.
*
*/
private @Nullable String customerQueryString;
/**
* @return Specifies a Perl-compatible regular expression with which to substitute the request's customer ID.
*
*/
private @Nullable String customerRegex;
/**
* @return Specifies a string to replace the request's customer ID matched by `customerRegex`.
*
*/
private @Nullable String customerReplace;
/**
* @return This field is only intended for export compatibility purposes, and modifying it will not impact your use of the behavior.
*
*/
private @Nullable String customerTitle;
/**
* @return Indicates that your Akamai representative has locked this behavior or criteria so that you can't modify it. This option is for internal usage only.
*
*/
private @Nullable Boolean locked;
/**
* @return This option is for internal usage only.
*
*/
private @Nullable String templateUuid;
/**
* @return Specifies the request component that identifies a SaaS user.
*
*/
private @Nullable String usersAction;
/**
* @return Enabling this allows you to identify users using a `CNAME chain` rather than a single hostname.
*
*/
private @Nullable Boolean usersCnameEnabled;
/**
* @return Specifies the number of CNAMEs to use in the chain.
*
*/
private @Nullable Integer usersCnameLevel;
/**
* @return This specifies the name of the cookie that identifies the user.
*
*/
private @Nullable String usersCookie;
/**
* @return This names the query parameter that identifies the user.
*
*/
private @Nullable String usersQueryString;
/**
* @return Specifies a Perl-compatible regular expression with which to substitute the request's user ID.
*
*/
private @Nullable String usersRegex;
/**
* @return Specifies a string to replace the request's user ID matched by `usersRegex`.
*
*/
private @Nullable String usersReplace;
/**
* @return This field is only intended for export compatibility purposes, and modifying it will not impact your use of the behavior.
*
*/
private @Nullable String usersTitle;
/**
* @return A uuid member indicates that at least one of its component behaviors or criteria is advanced and read-only. You need to preserve this uuid as well when modifying the rule tree. This option is for internal usage only.
*
*/
private @Nullable String uuid;
private GetPropertyRulesBuilderRulesV20240212BehaviorSaasDefinitions() {}
/**
* @return Specifies the request component that identifies a SaaS application.
*
*/
public Optional applicationAction() {
return Optional.ofNullable(this.applicationAction);
}
/**
* @return Enabling this allows you to identify applications using a `CNAME chain` rather than a single hostname.
*
*/
public Optional applicationCnameEnabled() {
return Optional.ofNullable(this.applicationCnameEnabled);
}
/**
* @return Specifies the number of CNAMEs to use in the chain.
*
*/
public Optional applicationCnameLevel() {
return Optional.ofNullable(this.applicationCnameLevel);
}
/**
* @return This specifies the name of the cookie that identifies the application.
*
*/
public Optional applicationCookie() {
return Optional.ofNullable(this.applicationCookie);
}
/**
* @return This names the query parameter that identifies the application.
*
*/
public Optional applicationQueryString() {
return Optional.ofNullable(this.applicationQueryString);
}
/**
* @return Specifies a Perl-compatible regular expression with which to substitute the request's application ID.
*
*/
public Optional applicationRegex() {
return Optional.ofNullable(this.applicationRegex);
}
/**
* @return Specifies a string to replace the request's application ID matched by `applicationRegex`.
*
*/
public Optional applicationReplace() {
return Optional.ofNullable(this.applicationReplace);
}
/**
* @return This field is only intended for export compatibility purposes, and modifying it will not impact your use of the behavior.
*
*/
public Optional applicationTitle() {
return Optional.ofNullable(this.applicationTitle);
}
/**
* @return Specifies the request component that identifies a SaaS customer.
*
*/
public Optional customerAction() {
return Optional.ofNullable(this.customerAction);
}
/**
* @return Enabling this allows you to identify customers using a `CNAME chain` rather than a single hostname.
*
*/
public Optional customerCnameEnabled() {
return Optional.ofNullable(this.customerCnameEnabled);
}
/**
* @return Specifies the number of CNAMEs to use in the chain.
*
*/
public Optional customerCnameLevel() {
return Optional.ofNullable(this.customerCnameLevel);
}
/**
* @return This specifies the name of the cookie that identifies the customer.
*
*/
public Optional customerCookie() {
return Optional.ofNullable(this.customerCookie);
}
/**
* @return This names the query parameter that identifies the customer.
*
*/
public Optional customerQueryString() {
return Optional.ofNullable(this.customerQueryString);
}
/**
* @return Specifies a Perl-compatible regular expression with which to substitute the request's customer ID.
*
*/
public Optional customerRegex() {
return Optional.ofNullable(this.customerRegex);
}
/**
* @return Specifies a string to replace the request's customer ID matched by `customerRegex`.
*
*/
public Optional customerReplace() {
return Optional.ofNullable(this.customerReplace);
}
/**
* @return This field is only intended for export compatibility purposes, and modifying it will not impact your use of the behavior.
*
*/
public Optional customerTitle() {
return Optional.ofNullable(this.customerTitle);
}
/**
* @return Indicates that your Akamai representative has locked this behavior or criteria so that you can't modify it. This option is for internal usage only.
*
*/
public Optional locked() {
return Optional.ofNullable(this.locked);
}
/**
* @return This option is for internal usage only.
*
*/
public Optional templateUuid() {
return Optional.ofNullable(this.templateUuid);
}
/**
* @return Specifies the request component that identifies a SaaS user.
*
*/
public Optional usersAction() {
return Optional.ofNullable(this.usersAction);
}
/**
* @return Enabling this allows you to identify users using a `CNAME chain` rather than a single hostname.
*
*/
public Optional usersCnameEnabled() {
return Optional.ofNullable(this.usersCnameEnabled);
}
/**
* @return Specifies the number of CNAMEs to use in the chain.
*
*/
public Optional usersCnameLevel() {
return Optional.ofNullable(this.usersCnameLevel);
}
/**
* @return This specifies the name of the cookie that identifies the user.
*
*/
public Optional usersCookie() {
return Optional.ofNullable(this.usersCookie);
}
/**
* @return This names the query parameter that identifies the user.
*
*/
public Optional usersQueryString() {
return Optional.ofNullable(this.usersQueryString);
}
/**
* @return Specifies a Perl-compatible regular expression with which to substitute the request's user ID.
*
*/
public Optional usersRegex() {
return Optional.ofNullable(this.usersRegex);
}
/**
* @return Specifies a string to replace the request's user ID matched by `usersRegex`.
*
*/
public Optional usersReplace() {
return Optional.ofNullable(this.usersReplace);
}
/**
* @return This field is only intended for export compatibility purposes, and modifying it will not impact your use of the behavior.
*
*/
public Optional usersTitle() {
return Optional.ofNullable(this.usersTitle);
}
/**
* @return A uuid member indicates that at least one of its component behaviors or criteria is advanced and read-only. You need to preserve this uuid as well when modifying the rule tree. This option is for internal usage only.
*
*/
public Optional uuid() {
return Optional.ofNullable(this.uuid);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetPropertyRulesBuilderRulesV20240212BehaviorSaasDefinitions defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String applicationAction;
private @Nullable Boolean applicationCnameEnabled;
private @Nullable Integer applicationCnameLevel;
private @Nullable String applicationCookie;
private @Nullable String applicationQueryString;
private @Nullable String applicationRegex;
private @Nullable String applicationReplace;
private @Nullable String applicationTitle;
private @Nullable String customerAction;
private @Nullable Boolean customerCnameEnabled;
private @Nullable Integer customerCnameLevel;
private @Nullable String customerCookie;
private @Nullable String customerQueryString;
private @Nullable String customerRegex;
private @Nullable String customerReplace;
private @Nullable String customerTitle;
private @Nullable Boolean locked;
private @Nullable String templateUuid;
private @Nullable String usersAction;
private @Nullable Boolean usersCnameEnabled;
private @Nullable Integer usersCnameLevel;
private @Nullable String usersCookie;
private @Nullable String usersQueryString;
private @Nullable String usersRegex;
private @Nullable String usersReplace;
private @Nullable String usersTitle;
private @Nullable String uuid;
public Builder() {}
public Builder(GetPropertyRulesBuilderRulesV20240212BehaviorSaasDefinitions defaults) {
Objects.requireNonNull(defaults);
this.applicationAction = defaults.applicationAction;
this.applicationCnameEnabled = defaults.applicationCnameEnabled;
this.applicationCnameLevel = defaults.applicationCnameLevel;
this.applicationCookie = defaults.applicationCookie;
this.applicationQueryString = defaults.applicationQueryString;
this.applicationRegex = defaults.applicationRegex;
this.applicationReplace = defaults.applicationReplace;
this.applicationTitle = defaults.applicationTitle;
this.customerAction = defaults.customerAction;
this.customerCnameEnabled = defaults.customerCnameEnabled;
this.customerCnameLevel = defaults.customerCnameLevel;
this.customerCookie = defaults.customerCookie;
this.customerQueryString = defaults.customerQueryString;
this.customerRegex = defaults.customerRegex;
this.customerReplace = defaults.customerReplace;
this.customerTitle = defaults.customerTitle;
this.locked = defaults.locked;
this.templateUuid = defaults.templateUuid;
this.usersAction = defaults.usersAction;
this.usersCnameEnabled = defaults.usersCnameEnabled;
this.usersCnameLevel = defaults.usersCnameLevel;
this.usersCookie = defaults.usersCookie;
this.usersQueryString = defaults.usersQueryString;
this.usersRegex = defaults.usersRegex;
this.usersReplace = defaults.usersReplace;
this.usersTitle = defaults.usersTitle;
this.uuid = defaults.uuid;
}
@CustomType.Setter
public Builder applicationAction(@Nullable String applicationAction) {
this.applicationAction = applicationAction;
return this;
}
@CustomType.Setter
public Builder applicationCnameEnabled(@Nullable Boolean applicationCnameEnabled) {
this.applicationCnameEnabled = applicationCnameEnabled;
return this;
}
@CustomType.Setter
public Builder applicationCnameLevel(@Nullable Integer applicationCnameLevel) {
this.applicationCnameLevel = applicationCnameLevel;
return this;
}
@CustomType.Setter
public Builder applicationCookie(@Nullable String applicationCookie) {
this.applicationCookie = applicationCookie;
return this;
}
@CustomType.Setter
public Builder applicationQueryString(@Nullable String applicationQueryString) {
this.applicationQueryString = applicationQueryString;
return this;
}
@CustomType.Setter
public Builder applicationRegex(@Nullable String applicationRegex) {
this.applicationRegex = applicationRegex;
return this;
}
@CustomType.Setter
public Builder applicationReplace(@Nullable String applicationReplace) {
this.applicationReplace = applicationReplace;
return this;
}
@CustomType.Setter
public Builder applicationTitle(@Nullable String applicationTitle) {
this.applicationTitle = applicationTitle;
return this;
}
@CustomType.Setter
public Builder customerAction(@Nullable String customerAction) {
this.customerAction = customerAction;
return this;
}
@CustomType.Setter
public Builder customerCnameEnabled(@Nullable Boolean customerCnameEnabled) {
this.customerCnameEnabled = customerCnameEnabled;
return this;
}
@CustomType.Setter
public Builder customerCnameLevel(@Nullable Integer customerCnameLevel) {
this.customerCnameLevel = customerCnameLevel;
return this;
}
@CustomType.Setter
public Builder customerCookie(@Nullable String customerCookie) {
this.customerCookie = customerCookie;
return this;
}
@CustomType.Setter
public Builder customerQueryString(@Nullable String customerQueryString) {
this.customerQueryString = customerQueryString;
return this;
}
@CustomType.Setter
public Builder customerRegex(@Nullable String customerRegex) {
this.customerRegex = customerRegex;
return this;
}
@CustomType.Setter
public Builder customerReplace(@Nullable String customerReplace) {
this.customerReplace = customerReplace;
return this;
}
@CustomType.Setter
public Builder customerTitle(@Nullable String customerTitle) {
this.customerTitle = customerTitle;
return this;
}
@CustomType.Setter
public Builder locked(@Nullable Boolean locked) {
this.locked = locked;
return this;
}
@CustomType.Setter
public Builder templateUuid(@Nullable String templateUuid) {
this.templateUuid = templateUuid;
return this;
}
@CustomType.Setter
public Builder usersAction(@Nullable String usersAction) {
this.usersAction = usersAction;
return this;
}
@CustomType.Setter
public Builder usersCnameEnabled(@Nullable Boolean usersCnameEnabled) {
this.usersCnameEnabled = usersCnameEnabled;
return this;
}
@CustomType.Setter
public Builder usersCnameLevel(@Nullable Integer usersCnameLevel) {
this.usersCnameLevel = usersCnameLevel;
return this;
}
@CustomType.Setter
public Builder usersCookie(@Nullable String usersCookie) {
this.usersCookie = usersCookie;
return this;
}
@CustomType.Setter
public Builder usersQueryString(@Nullable String usersQueryString) {
this.usersQueryString = usersQueryString;
return this;
}
@CustomType.Setter
public Builder usersRegex(@Nullable String usersRegex) {
this.usersRegex = usersRegex;
return this;
}
@CustomType.Setter
public Builder usersReplace(@Nullable String usersReplace) {
this.usersReplace = usersReplace;
return this;
}
@CustomType.Setter
public Builder usersTitle(@Nullable String usersTitle) {
this.usersTitle = usersTitle;
return this;
}
@CustomType.Setter
public Builder uuid(@Nullable String uuid) {
this.uuid = uuid;
return this;
}
public GetPropertyRulesBuilderRulesV20240212BehaviorSaasDefinitions build() {
final var _resultValue = new GetPropertyRulesBuilderRulesV20240212BehaviorSaasDefinitions();
_resultValue.applicationAction = applicationAction;
_resultValue.applicationCnameEnabled = applicationCnameEnabled;
_resultValue.applicationCnameLevel = applicationCnameLevel;
_resultValue.applicationCookie = applicationCookie;
_resultValue.applicationQueryString = applicationQueryString;
_resultValue.applicationRegex = applicationRegex;
_resultValue.applicationReplace = applicationReplace;
_resultValue.applicationTitle = applicationTitle;
_resultValue.customerAction = customerAction;
_resultValue.customerCnameEnabled = customerCnameEnabled;
_resultValue.customerCnameLevel = customerCnameLevel;
_resultValue.customerCookie = customerCookie;
_resultValue.customerQueryString = customerQueryString;
_resultValue.customerRegex = customerRegex;
_resultValue.customerReplace = customerReplace;
_resultValue.customerTitle = customerTitle;
_resultValue.locked = locked;
_resultValue.templateUuid = templateUuid;
_resultValue.usersAction = usersAction;
_resultValue.usersCnameEnabled = usersCnameEnabled;
_resultValue.usersCnameLevel = usersCnameLevel;
_resultValue.usersCookie = usersCookie;
_resultValue.usersQueryString = usersQueryString;
_resultValue.usersRegex = usersRegex;
_resultValue.usersReplace = usersReplace;
_resultValue.usersTitle = usersTitle;
_resultValue.uuid = uuid;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy