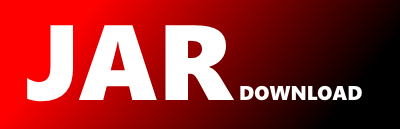
com.pulumi.alicloud.cs.outputs.GetKubernetesClustersClusterConnections Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of alicloud Show documentation
Show all versions of alicloud Show documentation
A Pulumi package for creating and managing AliCloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.alicloud.cs.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class GetKubernetesClustersClusterConnections {
/**
* @return API Server Internet endpoint.
*
*/
private String apiServerInternet;
/**
* @return API Server Intranet endpoint.
*
*/
private String apiServerIntranet;
/**
* @return Master node SSH IP address.
*
*/
private String masterPublicIp;
/**
* @return Service Access Domain.
*
*/
private String serviceDomain;
private GetKubernetesClustersClusterConnections() {}
/**
* @return API Server Internet endpoint.
*
*/
public String apiServerInternet() {
return this.apiServerInternet;
}
/**
* @return API Server Intranet endpoint.
*
*/
public String apiServerIntranet() {
return this.apiServerIntranet;
}
/**
* @return Master node SSH IP address.
*
*/
public String masterPublicIp() {
return this.masterPublicIp;
}
/**
* @return Service Access Domain.
*
*/
public String serviceDomain() {
return this.serviceDomain;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetKubernetesClustersClusterConnections defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String apiServerInternet;
private String apiServerIntranet;
private String masterPublicIp;
private String serviceDomain;
public Builder() {}
public Builder(GetKubernetesClustersClusterConnections defaults) {
Objects.requireNonNull(defaults);
this.apiServerInternet = defaults.apiServerInternet;
this.apiServerIntranet = defaults.apiServerIntranet;
this.masterPublicIp = defaults.masterPublicIp;
this.serviceDomain = defaults.serviceDomain;
}
@CustomType.Setter
public Builder apiServerInternet(String apiServerInternet) {
if (apiServerInternet == null) {
throw new MissingRequiredPropertyException("GetKubernetesClustersClusterConnections", "apiServerInternet");
}
this.apiServerInternet = apiServerInternet;
return this;
}
@CustomType.Setter
public Builder apiServerIntranet(String apiServerIntranet) {
if (apiServerIntranet == null) {
throw new MissingRequiredPropertyException("GetKubernetesClustersClusterConnections", "apiServerIntranet");
}
this.apiServerIntranet = apiServerIntranet;
return this;
}
@CustomType.Setter
public Builder masterPublicIp(String masterPublicIp) {
if (masterPublicIp == null) {
throw new MissingRequiredPropertyException("GetKubernetesClustersClusterConnections", "masterPublicIp");
}
this.masterPublicIp = masterPublicIp;
return this;
}
@CustomType.Setter
public Builder serviceDomain(String serviceDomain) {
if (serviceDomain == null) {
throw new MissingRequiredPropertyException("GetKubernetesClustersClusterConnections", "serviceDomain");
}
this.serviceDomain = serviceDomain;
return this;
}
public GetKubernetesClustersClusterConnections build() {
final var _resultValue = new GetKubernetesClustersClusterConnections();
_resultValue.apiServerInternet = apiServerInternet;
_resultValue.apiServerIntranet = apiServerIntranet;
_resultValue.masterPublicIp = masterPublicIp;
_resultValue.serviceDomain = serviceDomain;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy