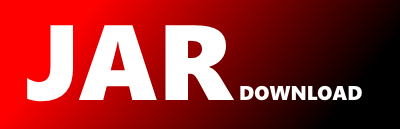
com.pulumi.alicloud.quotas.inputs.GetTemplateApplicationsArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of alicloud Show documentation
Show all versions of alicloud Show documentation
A Pulumi package for creating and managing AliCloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.alicloud.quotas.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class GetTemplateApplicationsArgs extends com.pulumi.resources.InvokeArgs {
public static final GetTemplateApplicationsArgs Empty = new GetTemplateApplicationsArgs();
/**
* The ID of the quota application batch.
*
*/
@Import(name="batchQuotaApplicationId")
private @Nullable Output batchQuotaApplicationId;
/**
* @return The ID of the quota application batch.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy