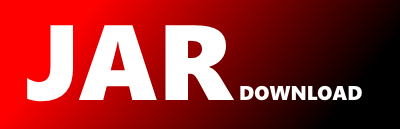
com.pulumi.aws.appautoscaling.Target Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.appautoscaling;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.appautoscaling.TargetArgs;
import com.pulumi.aws.appautoscaling.inputs.TargetState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides an Application AutoScaling ScalableTarget resource. To manage policies which get attached to the target, see the `aws.appautoscaling.Policy` resource.
*
* > **NOTE:** Scalable targets created before 2023-03-20 may not have an assigned `arn`. These resource cannot use `tags` or participate in `default_tags`. To prevent `pulumi preview` showing differences that can never be reconciled, use the `lifecycle.ignore_changes` meta-argument. See the example below.
*
* > **NOTE:** The [Application Auto Scaling service automatically attempts to manage IAM Service-Linked Roles](https://docs.aws.amazon.com/autoscaling/application/userguide/security_iam_service-with-iam.html#security_iam_service-with-iam-roles) when registering certain service namespaces for the first time. To manually manage this role, see the `aws.iam.ServiceLinkedRole` resource.
*
* ## Example Usage
*
* ### DynamoDB Table Autoscaling
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.appautoscaling.Target;
* import com.pulumi.aws.appautoscaling.TargetArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var dynamodbTableReadTarget = new Target("dynamodbTableReadTarget", TargetArgs.builder()
* .maxCapacity(100)
* .minCapacity(5)
* .resourceId(String.format("table/%s", example.name()))
* .scalableDimension("dynamodb:table:ReadCapacityUnits")
* .serviceNamespace("dynamodb")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### DynamoDB Index Autoscaling
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.appautoscaling.Target;
* import com.pulumi.aws.appautoscaling.TargetArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var dynamodbIndexReadTarget = new Target("dynamodbIndexReadTarget", TargetArgs.builder()
* .maxCapacity(100)
* .minCapacity(5)
* .resourceId(String.format("table/%s/index/%s", example.name(),indexName))
* .scalableDimension("dynamodb:index:ReadCapacityUnits")
* .serviceNamespace("dynamodb")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### ECS Service Autoscaling
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.appautoscaling.Target;
* import com.pulumi.aws.appautoscaling.TargetArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var ecsTarget = new Target("ecsTarget", TargetArgs.builder()
* .maxCapacity(4)
* .minCapacity(1)
* .resourceId(String.format("service/%s/%s", example.name(),exampleAwsEcsService.name()))
* .scalableDimension("ecs:service:DesiredCount")
* .serviceNamespace("ecs")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Aurora Read Replica Autoscaling
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.appautoscaling.Target;
* import com.pulumi.aws.appautoscaling.TargetArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var replicas = new Target("replicas", TargetArgs.builder()
* .serviceNamespace("rds")
* .scalableDimension("rds:cluster:ReadReplicaCount")
* .resourceId(String.format("cluster:%s", example.id()))
* .minCapacity(1)
* .maxCapacity(15)
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Suppressing `tags_all` Differences For Older Resources
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.appautoscaling.Target;
* import com.pulumi.aws.appautoscaling.TargetArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var ecsTarget = new Target("ecsTarget", TargetArgs.builder()
* .maxCapacity(4)
* .minCapacity(1)
* .resourceId(String.format("service/%s/%s", example.name(),exampleAwsEcsService.name()))
* .scalableDimension("ecs:service:DesiredCount")
* .serviceNamespace("ecs")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### MSK / Kafka Autoscaling
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.appautoscaling.Target;
* import com.pulumi.aws.appautoscaling.TargetArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var mskTarget = new Target("mskTarget", TargetArgs.builder()
* .serviceNamespace("kafka")
* .scalableDimension("kafka:broker-storage:VolumeSize")
* .resourceId(example.arn())
* .minCapacity(1)
* .maxCapacity(8)
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import Application AutoScaling Target using the `service-namespace` , `resource-id` and `scalable-dimension` separated by `/`. For example:
*
* ```sh
* $ pulumi import aws:appautoscaling/target:Target test-target service-namespace/resource-id/scalable-dimension
* ```
*
*/
@ResourceType(type="aws:appautoscaling/target:Target")
public class Target extends com.pulumi.resources.CustomResource {
/**
* The ARN of the scalable target.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return The ARN of the scalable target.
*
*/
public Output arn() {
return this.arn;
}
/**
* Max capacity of the scalable target.
*
*/
@Export(name="maxCapacity", refs={Integer.class}, tree="[0]")
private Output maxCapacity;
/**
* @return Max capacity of the scalable target.
*
*/
public Output maxCapacity() {
return this.maxCapacity;
}
/**
* Min capacity of the scalable target.
*
*/
@Export(name="minCapacity", refs={Integer.class}, tree="[0]")
private Output minCapacity;
/**
* @return Min capacity of the scalable target.
*
*/
public Output minCapacity() {
return this.minCapacity;
}
/**
* Resource type and unique identifier string for the resource associated with the scaling policy. Documentation can be found in the `ResourceId` parameter at: [AWS Application Auto Scaling API Reference](https://docs.aws.amazon.com/autoscaling/application/APIReference/API_RegisterScalableTarget.html#API_RegisterScalableTarget_RequestParameters)
*
*/
@Export(name="resourceId", refs={String.class}, tree="[0]")
private Output resourceId;
/**
* @return Resource type and unique identifier string for the resource associated with the scaling policy. Documentation can be found in the `ResourceId` parameter at: [AWS Application Auto Scaling API Reference](https://docs.aws.amazon.com/autoscaling/application/APIReference/API_RegisterScalableTarget.html#API_RegisterScalableTarget_RequestParameters)
*
*/
public Output resourceId() {
return this.resourceId;
}
/**
* ARN of the IAM role that allows Application AutoScaling to modify your scalable target on your behalf. This defaults to an IAM Service-Linked Role for most services and custom IAM Roles are ignored by the API for those namespaces. See the [AWS Application Auto Scaling documentation](https://docs.aws.amazon.com/autoscaling/application/userguide/security_iam_service-with-iam.html#security_iam_service-with-iam-roles) for more information about how this service interacts with IAM.
*
*/
@Export(name="roleArn", refs={String.class}, tree="[0]")
private Output roleArn;
/**
* @return ARN of the IAM role that allows Application AutoScaling to modify your scalable target on your behalf. This defaults to an IAM Service-Linked Role for most services and custom IAM Roles are ignored by the API for those namespaces. See the [AWS Application Auto Scaling documentation](https://docs.aws.amazon.com/autoscaling/application/userguide/security_iam_service-with-iam.html#security_iam_service-with-iam-roles) for more information about how this service interacts with IAM.
*
*/
public Output roleArn() {
return this.roleArn;
}
/**
* Scalable dimension of the scalable target. Documentation can be found in the `ScalableDimension` parameter at: [AWS Application Auto Scaling API Reference](https://docs.aws.amazon.com/autoscaling/application/APIReference/API_RegisterScalableTarget.html#API_RegisterScalableTarget_RequestParameters)
*
*/
@Export(name="scalableDimension", refs={String.class}, tree="[0]")
private Output scalableDimension;
/**
* @return Scalable dimension of the scalable target. Documentation can be found in the `ScalableDimension` parameter at: [AWS Application Auto Scaling API Reference](https://docs.aws.amazon.com/autoscaling/application/APIReference/API_RegisterScalableTarget.html#API_RegisterScalableTarget_RequestParameters)
*
*/
public Output scalableDimension() {
return this.scalableDimension;
}
/**
* AWS service namespace of the scalable target. Documentation can be found in the `ServiceNamespace` parameter at: [AWS Application Auto Scaling API Reference](https://docs.aws.amazon.com/autoscaling/application/APIReference/API_RegisterScalableTarget.html#API_RegisterScalableTarget_RequestParameters)
*
*/
@Export(name="serviceNamespace", refs={String.class}, tree="[0]")
private Output serviceNamespace;
/**
* @return AWS service namespace of the scalable target. Documentation can be found in the `ServiceNamespace` parameter at: [AWS Application Auto Scaling API Reference](https://docs.aws.amazon.com/autoscaling/application/APIReference/API_RegisterScalableTarget.html#API_RegisterScalableTarget_RequestParameters)
*
*/
public Output serviceNamespace() {
return this.serviceNamespace;
}
/**
* Map of tags to assign to the scalable target. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Map of tags to assign to the scalable target. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* Map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy