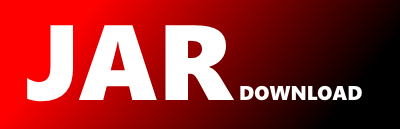
com.pulumi.aws.backup.inputs.PlanRuleArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.backup.inputs;
import com.pulumi.aws.backup.inputs.PlanRuleCopyActionArgs;
import com.pulumi.aws.backup.inputs.PlanRuleLifecycleArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class PlanRuleArgs extends com.pulumi.resources.ResourceArgs {
public static final PlanRuleArgs Empty = new PlanRuleArgs();
/**
* The amount of time in minutes AWS Backup attempts a backup before canceling the job and returning an error.
*
*/
@Import(name="completionWindow")
private @Nullable Output completionWindow;
/**
* @return The amount of time in minutes AWS Backup attempts a backup before canceling the job and returning an error.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy