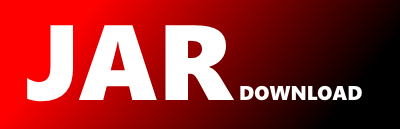
com.pulumi.aws.cfg.ConformancePackArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.cfg;
import com.pulumi.aws.cfg.inputs.ConformancePackInputParameterArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ConformancePackArgs extends com.pulumi.resources.ResourceArgs {
public static final ConformancePackArgs Empty = new ConformancePackArgs();
/**
* Amazon S3 bucket where AWS Config stores conformance pack templates. Maximum length of 63.
*
*/
@Import(name="deliveryS3Bucket")
private @Nullable Output deliveryS3Bucket;
/**
* @return Amazon S3 bucket where AWS Config stores conformance pack templates. Maximum length of 63.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy