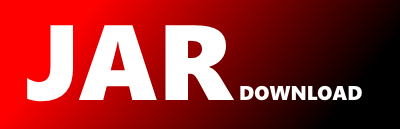
com.pulumi.aws.cfg.OrganizationManagedRule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.cfg;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.cfg.OrganizationManagedRuleArgs;
import com.pulumi.aws.cfg.inputs.OrganizationManagedRuleState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Manages a Config Organization Managed Rule. More information about these rules can be found in the [Enabling AWS Config Rules Across all Accounts in Your Organization](https://docs.aws.amazon.com/config/latest/developerguide/config-rule-multi-account-deployment.html) and [AWS Config Managed Rules](https://docs.aws.amazon.com/config/latest/developerguide/evaluate-config_use-managed-rules.html) documentation. For working with Organization Custom Rules (those invoking a custom Lambda Function), see the `aws.cfg.OrganizationCustomRule` resource.
*
* > **NOTE:** This resource must be created in the Organization master account and rules will include the master account unless its ID is added to the `excluded_accounts` argument.
*
* > **NOTE:** Every Organization account except those configured in the `excluded_accounts` argument must have a Configuration Recorder with proper IAM permissions before the rule will successfully create or update. See also the `aws.cfg.Recorder` resource.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.organizations.Organization;
* import com.pulumi.aws.organizations.OrganizationArgs;
* import com.pulumi.aws.cfg.OrganizationManagedRule;
* import com.pulumi.aws.cfg.OrganizationManagedRuleArgs;
* import com.pulumi.resources.CustomResourceOptions;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Organization("example", OrganizationArgs.builder()
* .awsServiceAccessPrincipals("config-multiaccountsetup.amazonaws.com")
* .featureSet("ALL")
* .build());
*
* var exampleOrganizationManagedRule = new OrganizationManagedRule("exampleOrganizationManagedRule", OrganizationManagedRuleArgs.builder()
* .name("example")
* .ruleIdentifier("IAM_PASSWORD_POLICY")
* .build(), CustomResourceOptions.builder()
* .dependsOn(example)
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import Config Organization Managed Rules using the name. For example:
*
* ```sh
* $ pulumi import aws:cfg/organizationManagedRule:OrganizationManagedRule example example
* ```
*
*/
@ResourceType(type="aws:cfg/organizationManagedRule:OrganizationManagedRule")
public class OrganizationManagedRule extends com.pulumi.resources.CustomResource {
/**
* Amazon Resource Name (ARN) of the rule
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return Amazon Resource Name (ARN) of the rule
*
*/
public Output arn() {
return this.arn;
}
/**
* Description of the rule
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> description;
/**
* @return Description of the rule
*
*/
public Output> description() {
return Codegen.optional(this.description);
}
/**
* List of AWS account identifiers to exclude from the rule
*
*/
@Export(name="excludedAccounts", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> excludedAccounts;
/**
* @return List of AWS account identifiers to exclude from the rule
*
*/
public Output>> excludedAccounts() {
return Codegen.optional(this.excludedAccounts);
}
/**
* A string in JSON format that is passed to the AWS Config Rule Lambda Function
*
*/
@Export(name="inputParameters", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> inputParameters;
/**
* @return A string in JSON format that is passed to the AWS Config Rule Lambda Function
*
*/
public Output> inputParameters() {
return Codegen.optional(this.inputParameters);
}
/**
* The maximum frequency with which AWS Config runs evaluations for a rule, if the rule is triggered at a periodic frequency. Defaults to `TwentyFour_Hours` for periodic frequency triggered rules. Valid values: `One_Hour`, `Three_Hours`, `Six_Hours`, `Twelve_Hours`, or `TwentyFour_Hours`.
*
*/
@Export(name="maximumExecutionFrequency", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> maximumExecutionFrequency;
/**
* @return The maximum frequency with which AWS Config runs evaluations for a rule, if the rule is triggered at a periodic frequency. Defaults to `TwentyFour_Hours` for periodic frequency triggered rules. Valid values: `One_Hour`, `Three_Hours`, `Six_Hours`, `Twelve_Hours`, or `TwentyFour_Hours`.
*
*/
public Output> maximumExecutionFrequency() {
return Codegen.optional(this.maximumExecutionFrequency);
}
/**
* The name of the rule
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the rule
*
*/
public Output name() {
return this.name;
}
/**
* Identifier of the AWS resource to evaluate
*
*/
@Export(name="resourceIdScope", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> resourceIdScope;
/**
* @return Identifier of the AWS resource to evaluate
*
*/
public Output> resourceIdScope() {
return Codegen.optional(this.resourceIdScope);
}
/**
* List of types of AWS resources to evaluate
*
*/
@Export(name="resourceTypesScopes", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> resourceTypesScopes;
/**
* @return List of types of AWS resources to evaluate
*
*/
public Output>> resourceTypesScopes() {
return Codegen.optional(this.resourceTypesScopes);
}
/**
* Identifier of an available AWS Config Managed Rule to call. For available values, see the [List of AWS Config Managed Rules](https://docs.aws.amazon.com/config/latest/developerguide/managed-rules-by-aws-config.html) documentation
*
*/
@Export(name="ruleIdentifier", refs={String.class}, tree="[0]")
private Output ruleIdentifier;
/**
* @return Identifier of an available AWS Config Managed Rule to call. For available values, see the [List of AWS Config Managed Rules](https://docs.aws.amazon.com/config/latest/developerguide/managed-rules-by-aws-config.html) documentation
*
*/
public Output ruleIdentifier() {
return this.ruleIdentifier;
}
/**
* Tag key of AWS resources to evaluate
*
*/
@Export(name="tagKeyScope", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> tagKeyScope;
/**
* @return Tag key of AWS resources to evaluate
*
*/
public Output> tagKeyScope() {
return Codegen.optional(this.tagKeyScope);
}
/**
* Tag value of AWS resources to evaluate
*
*/
@Export(name="tagValueScope", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> tagValueScope;
/**
* @return Tag value of AWS resources to evaluate
*
*/
public Output> tagValueScope() {
return Codegen.optional(this.tagValueScope);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public OrganizationManagedRule(String name) {
this(name, OrganizationManagedRuleArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public OrganizationManagedRule(String name, OrganizationManagedRuleArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public OrganizationManagedRule(String name, OrganizationManagedRuleArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:cfg/organizationManagedRule:OrganizationManagedRule", name, args == null ? OrganizationManagedRuleArgs.Empty : args, makeResourceOptions(options, Codegen.empty()));
}
private OrganizationManagedRule(String name, Output id, @Nullable OrganizationManagedRuleState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:cfg/organizationManagedRule:OrganizationManagedRule", name, state, makeResourceOptions(options, id));
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static OrganizationManagedRule get(String name, Output id, @Nullable OrganizationManagedRuleState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new OrganizationManagedRule(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy