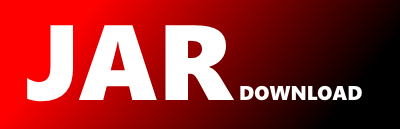
com.pulumi.aws.cloudwatch.inputs.InternetMonitorState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.cloudwatch.inputs;
import com.pulumi.aws.cloudwatch.inputs.InternetMonitorHealthEventsConfigArgs;
import com.pulumi.aws.cloudwatch.inputs.InternetMonitorInternetMeasurementsLogDeliveryArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class InternetMonitorState extends com.pulumi.resources.ResourceArgs {
public static final InternetMonitorState Empty = new InternetMonitorState();
/**
* ARN of the Monitor.
*
*/
@Import(name="arn")
private @Nullable Output arn;
/**
* @return ARN of the Monitor.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy