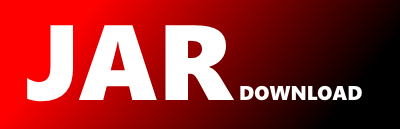
com.pulumi.aws.cognito.UserArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.cognito;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class UserArgs extends com.pulumi.resources.ResourceArgs {
public static final UserArgs Empty = new UserArgs();
/**
* A map that contains user attributes and attribute values to be set for the user.
*
*/
@Import(name="attributes")
private @Nullable Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy