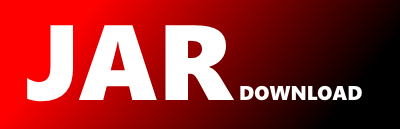
com.pulumi.aws.connect.inputs.RoutingProfileState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.connect.inputs;
import com.pulumi.aws.connect.inputs.RoutingProfileMediaConcurrencyArgs;
import com.pulumi.aws.connect.inputs.RoutingProfileQueueConfigArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class RoutingProfileState extends com.pulumi.resources.ResourceArgs {
public static final RoutingProfileState Empty = new RoutingProfileState();
/**
* The Amazon Resource Name (ARN) of the Routing Profile.
*
*/
@Import(name="arn")
private @Nullable Output arn;
/**
* @return The Amazon Resource Name (ARN) of the Routing Profile.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy