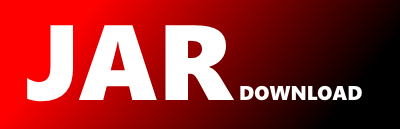
com.pulumi.aws.dms.inputs.EndpointRedisSettingsArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.dms.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class EndpointRedisSettingsArgs extends com.pulumi.resources.ResourceArgs {
public static final EndpointRedisSettingsArgs Empty = new EndpointRedisSettingsArgs();
/**
* The password provided with the auth-role and auth-token options of the AuthType setting for a Redis target endpoint.
*
*/
@Import(name="authPassword")
private @Nullable Output authPassword;
/**
* @return The password provided with the auth-role and auth-token options of the AuthType setting for a Redis target endpoint.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy