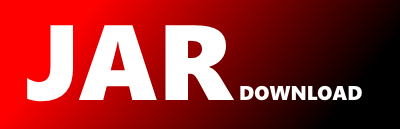
com.pulumi.aws.ec2.AmiCopy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.ec2.AmiCopyArgs;
import com.pulumi.aws.ec2.inputs.AmiCopyState;
import com.pulumi.aws.ec2.outputs.AmiCopyEbsBlockDevice;
import com.pulumi.aws.ec2.outputs.AmiCopyEphemeralBlockDevice;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The "AMI copy" resource allows duplication of an Amazon Machine Image (AMI),
* including cross-region copies.
*
* If the source AMI has associated EBS snapshots, those will also be duplicated
* along with the AMI.
*
* This is useful for taking a single AMI provisioned in one region and making
* it available in another for a multi-region deployment.
*
* Copying an AMI can take several minutes. The creation of this resource will
* block until the new AMI is available for use on new instances.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ec2.AmiCopy;
* import com.pulumi.aws.ec2.AmiCopyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new AmiCopy("example", AmiCopyArgs.builder()
* .name("example")
* .description("A copy of ami-xxxxxxxx")
* .sourceAmiId("ami-xxxxxxxx")
* .sourceAmiRegion("us-west-1")
* .tags(Map.of("Name", "HelloWorld"))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
*/
@ResourceType(type="aws:ec2/amiCopy:AmiCopy")
public class AmiCopy extends com.pulumi.resources.CustomResource {
/**
* Machine architecture for created instances. Defaults to "x86_64".
*
*/
@Export(name="architecture", refs={String.class}, tree="[0]")
private Output architecture;
/**
* @return Machine architecture for created instances. Defaults to "x86_64".
*
*/
public Output architecture() {
return this.architecture;
}
/**
* ARN of the AMI.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return ARN of the AMI.
*
*/
public Output arn() {
return this.arn;
}
/**
* Boot mode of the AMI. For more information, see [Boot modes](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/ami-boot.html) in the Amazon Elastic Compute Cloud User Guide.
*
*/
@Export(name="bootMode", refs={String.class}, tree="[0]")
private Output bootMode;
/**
* @return Boot mode of the AMI. For more information, see [Boot modes](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/ami-boot.html) in the Amazon Elastic Compute Cloud User Guide.
*
*/
public Output bootMode() {
return this.bootMode;
}
/**
* Date and time to deprecate the AMI. If you specified a value for seconds, Amazon EC2 rounds the seconds to the nearest minute. Valid values: [RFC3339 time string](https://tools.ietf.org/html/rfc3339#section-5.8) (`YYYY-MM-DDTHH:MM:SSZ`)
*
*/
@Export(name="deprecationTime", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> deprecationTime;
/**
* @return Date and time to deprecate the AMI. If you specified a value for seconds, Amazon EC2 rounds the seconds to the nearest minute. Valid values: [RFC3339 time string](https://tools.ietf.org/html/rfc3339#section-5.8) (`YYYY-MM-DDTHH:MM:SSZ`)
*
*/
public Output> deprecationTime() {
return Codegen.optional(this.deprecationTime);
}
/**
* Longer, human-readable description for the AMI.
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> description;
/**
* @return Longer, human-readable description for the AMI.
*
*/
public Output> description() {
return Codegen.optional(this.description);
}
/**
* ARN of the Outpost to which to copy the AMI.
* Only specify this parameter when copying an AMI from an AWS Region to an Outpost. The AMI must be in the Region of the destination Outpost.
*
*/
@Export(name="destinationOutpostArn", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> destinationOutpostArn;
/**
* @return ARN of the Outpost to which to copy the AMI.
* Only specify this parameter when copying an AMI from an AWS Region to an Outpost. The AMI must be in the Region of the destination Outpost.
*
*/
public Output> destinationOutpostArn() {
return Codegen.optional(this.destinationOutpostArn);
}
/**
* Nested block describing an EBS block device that should be
* attached to created instances. The structure of this block is described below.
*
*/
@Export(name="ebsBlockDevices", refs={List.class,AmiCopyEbsBlockDevice.class}, tree="[0,1]")
private Output> ebsBlockDevices;
/**
* @return Nested block describing an EBS block device that should be
* attached to created instances. The structure of this block is described below.
*
*/
public Output> ebsBlockDevices() {
return this.ebsBlockDevices;
}
/**
* Whether enhanced networking with ENA is enabled. Defaults to `false`.
*
*/
@Export(name="enaSupport", refs={Boolean.class}, tree="[0]")
private Output enaSupport;
/**
* @return Whether enhanced networking with ENA is enabled. Defaults to `false`.
*
*/
public Output enaSupport() {
return this.enaSupport;
}
/**
* Whether the destination snapshots of the copied image should be encrypted. Defaults to `false`
*
*/
@Export(name="encrypted", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> encrypted;
/**
* @return Whether the destination snapshots of the copied image should be encrypted. Defaults to `false`
*
*/
public Output> encrypted() {
return Codegen.optional(this.encrypted);
}
/**
* Nested block describing an ephemeral block device that
* should be attached to created instances. The structure of this block is described below.
*
*/
@Export(name="ephemeralBlockDevices", refs={List.class,AmiCopyEphemeralBlockDevice.class}, tree="[0,1]")
private Output> ephemeralBlockDevices;
/**
* @return Nested block describing an ephemeral block device that
* should be attached to created instances. The structure of this block is described below.
*
*/
public Output> ephemeralBlockDevices() {
return this.ephemeralBlockDevices;
}
@Export(name="hypervisor", refs={String.class}, tree="[0]")
private Output hypervisor;
public Output hypervisor() {
return this.hypervisor;
}
/**
* Path to an S3 object containing an image manifest, e.g., created
* by the `ec2-upload-bundle` command in the EC2 command line tools.
*
*/
@Export(name="imageLocation", refs={String.class}, tree="[0]")
private Output imageLocation;
/**
* @return Path to an S3 object containing an image manifest, e.g., created
* by the `ec2-upload-bundle` command in the EC2 command line tools.
*
*/
public Output imageLocation() {
return this.imageLocation;
}
@Export(name="imageOwnerAlias", refs={String.class}, tree="[0]")
private Output imageOwnerAlias;
public Output imageOwnerAlias() {
return this.imageOwnerAlias;
}
@Export(name="imageType", refs={String.class}, tree="[0]")
private Output imageType;
public Output imageType() {
return this.imageType;
}
/**
* If EC2 instances started from this image should require the use of the Instance Metadata Service V2 (IMDSv2), set this argument to `v2.0`. For more information, see [Configure instance metadata options for new instances](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/configuring-IMDS-new-instances.html#configure-IMDS-new-instances-ami-configuration).
*
*/
@Export(name="imdsSupport", refs={String.class}, tree="[0]")
private Output imdsSupport;
/**
* @return If EC2 instances started from this image should require the use of the Instance Metadata Service V2 (IMDSv2), set this argument to `v2.0`. For more information, see [Configure instance metadata options for new instances](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/configuring-IMDS-new-instances.html#configure-IMDS-new-instances-ami-configuration).
*
*/
public Output imdsSupport() {
return this.imdsSupport;
}
/**
* ID of the kernel image (AKI) that will be used as the paravirtual
* kernel in created instances.
*
*/
@Export(name="kernelId", refs={String.class}, tree="[0]")
private Output kernelId;
/**
* @return ID of the kernel image (AKI) that will be used as the paravirtual
* kernel in created instances.
*
*/
public Output kernelId() {
return this.kernelId;
}
/**
* Full ARN of the KMS Key to use when encrypting the snapshots of an image during a copy operation. If not specified, then the default AWS KMS Key will be used
*
*/
@Export(name="kmsKeyId", refs={String.class}, tree="[0]")
private Output kmsKeyId;
/**
* @return Full ARN of the KMS Key to use when encrypting the snapshots of an image during a copy operation. If not specified, then the default AWS KMS Key will be used
*
*/
public Output kmsKeyId() {
return this.kmsKeyId;
}
@Export(name="manageEbsSnapshots", refs={Boolean.class}, tree="[0]")
private Output manageEbsSnapshots;
public Output manageEbsSnapshots() {
return this.manageEbsSnapshots;
}
/**
* Region-unique name for the AMI.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Region-unique name for the AMI.
*
*/
public Output name() {
return this.name;
}
@Export(name="ownerId", refs={String.class}, tree="[0]")
private Output ownerId;
public Output ownerId() {
return this.ownerId;
}
@Export(name="platform", refs={String.class}, tree="[0]")
private Output platform;
public Output platform() {
return this.platform;
}
@Export(name="platformDetails", refs={String.class}, tree="[0]")
private Output platformDetails;
public Output platformDetails() {
return this.platformDetails;
}
@Export(name="public", refs={Boolean.class}, tree="[0]")
private Output public_;
public Output public_() {
return this.public_;
}
/**
* ID of an initrd image (ARI) that will be used when booting the
* created instances.
*
*/
@Export(name="ramdiskId", refs={String.class}, tree="[0]")
private Output ramdiskId;
/**
* @return ID of an initrd image (ARI) that will be used when booting the
* created instances.
*
*/
public Output ramdiskId() {
return this.ramdiskId;
}
/**
* Name of the root device (for example, `/dev/sda1`, or `/dev/xvda`).
*
*/
@Export(name="rootDeviceName", refs={String.class}, tree="[0]")
private Output rootDeviceName;
/**
* @return Name of the root device (for example, `/dev/sda1`, or `/dev/xvda`).
*
*/
public Output rootDeviceName() {
return this.rootDeviceName;
}
@Export(name="rootSnapshotId", refs={String.class}, tree="[0]")
private Output rootSnapshotId;
public Output rootSnapshotId() {
return this.rootSnapshotId;
}
/**
* Id of the AMI to copy. This id must be valid in the region
* given by `source_ami_region`.
*
*/
@Export(name="sourceAmiId", refs={String.class}, tree="[0]")
private Output sourceAmiId;
/**
* @return Id of the AMI to copy. This id must be valid in the region
* given by `source_ami_region`.
*
*/
public Output sourceAmiId() {
return this.sourceAmiId;
}
/**
* Region from which the AMI will be copied. This may be the
* same as the AWS provider region in order to create a copy within the same region.
*
*/
@Export(name="sourceAmiRegion", refs={String.class}, tree="[0]")
private Output sourceAmiRegion;
/**
* @return Region from which the AMI will be copied. This may be the
* same as the AWS provider region in order to create a copy within the same region.
*
*/
public Output sourceAmiRegion() {
return this.sourceAmiRegion;
}
/**
* When set to "simple" (the default), enables enhanced networking
* for created instances. No other value is supported at this time.
*
*/
@Export(name="sriovNetSupport", refs={String.class}, tree="[0]")
private Output sriovNetSupport;
/**
* @return When set to "simple" (the default), enables enhanced networking
* for created instances. No other value is supported at this time.
*
*/
public Output sriovNetSupport() {
return this.sriovNetSupport;
}
/**
* Map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy