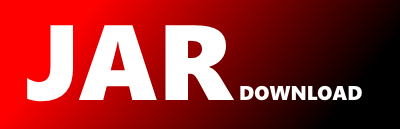
com.pulumi.aws.ec2.inputs.LaunchTemplateState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2.inputs;
import com.pulumi.aws.ec2.inputs.LaunchTemplateBlockDeviceMappingArgs;
import com.pulumi.aws.ec2.inputs.LaunchTemplateCapacityReservationSpecificationArgs;
import com.pulumi.aws.ec2.inputs.LaunchTemplateCpuOptionsArgs;
import com.pulumi.aws.ec2.inputs.LaunchTemplateCreditSpecificationArgs;
import com.pulumi.aws.ec2.inputs.LaunchTemplateElasticGpuSpecificationArgs;
import com.pulumi.aws.ec2.inputs.LaunchTemplateElasticInferenceAcceleratorArgs;
import com.pulumi.aws.ec2.inputs.LaunchTemplateEnclaveOptionsArgs;
import com.pulumi.aws.ec2.inputs.LaunchTemplateHibernationOptionsArgs;
import com.pulumi.aws.ec2.inputs.LaunchTemplateIamInstanceProfileArgs;
import com.pulumi.aws.ec2.inputs.LaunchTemplateInstanceMarketOptionsArgs;
import com.pulumi.aws.ec2.inputs.LaunchTemplateInstanceRequirementsArgs;
import com.pulumi.aws.ec2.inputs.LaunchTemplateLicenseSpecificationArgs;
import com.pulumi.aws.ec2.inputs.LaunchTemplateMaintenanceOptionsArgs;
import com.pulumi.aws.ec2.inputs.LaunchTemplateMetadataOptionsArgs;
import com.pulumi.aws.ec2.inputs.LaunchTemplateMonitoringArgs;
import com.pulumi.aws.ec2.inputs.LaunchTemplateNetworkInterfaceArgs;
import com.pulumi.aws.ec2.inputs.LaunchTemplatePlacementArgs;
import com.pulumi.aws.ec2.inputs.LaunchTemplatePrivateDnsNameOptionsArgs;
import com.pulumi.aws.ec2.inputs.LaunchTemplateTagSpecificationArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class LaunchTemplateState extends com.pulumi.resources.ResourceArgs {
public static final LaunchTemplateState Empty = new LaunchTemplateState();
/**
* Amazon Resource Name (ARN) of the launch template.
*
*/
@Import(name="arn")
private @Nullable Output arn;
/**
* @return Amazon Resource Name (ARN) of the launch template.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy