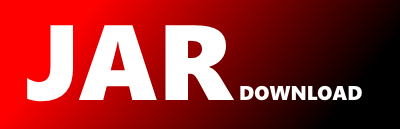
com.pulumi.aws.ec2.inputs.RouteTableRouteArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class RouteTableRouteArgs extends com.pulumi.resources.ResourceArgs {
public static final RouteTableRouteArgs Empty = new RouteTableRouteArgs();
/**
* Identifier of a carrier gateway. This attribute can only be used when the VPC contains a subnet which is associated with a Wavelength Zone.
*
*/
@Import(name="carrierGatewayId")
private @Nullable Output carrierGatewayId;
/**
* @return Identifier of a carrier gateway. This attribute can only be used when the VPC contains a subnet which is associated with a Wavelength Zone.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy