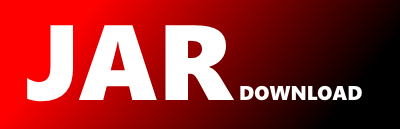
com.pulumi.aws.ec2.outputs.GetCustomerGatewayResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2.outputs;
import com.pulumi.aws.ec2.outputs.GetCustomerGatewayFilter;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import javax.annotation.Nullable;
@CustomType
public final class GetCustomerGatewayResult {
/**
* @return ARN of the customer gateway.
*
*/
private String arn;
/**
* @return Gateway's Border Gateway Protocol (BGP) Autonomous System Number (ASN).
*
*/
private Integer bgpAsn;
/**
* @return ARN for the customer gateway certificate.
*
*/
private String certificateArn;
/**
* @return Name for the customer gateway device.
*
*/
private String deviceName;
private @Nullable List filters;
private String id;
/**
* @return IP address of the gateway's Internet-routable external interface.
*
*/
private String ipAddress;
/**
* @return Map of key-value pairs assigned to the gateway.
*
*/
private Map tags;
/**
* @return Type of customer gateway. The only type AWS supports at this time is "ipsec.1".
*
*/
private String type;
private GetCustomerGatewayResult() {}
/**
* @return ARN of the customer gateway.
*
*/
public String arn() {
return this.arn;
}
/**
* @return Gateway's Border Gateway Protocol (BGP) Autonomous System Number (ASN).
*
*/
public Integer bgpAsn() {
return this.bgpAsn;
}
/**
* @return ARN for the customer gateway certificate.
*
*/
public String certificateArn() {
return this.certificateArn;
}
/**
* @return Name for the customer gateway device.
*
*/
public String deviceName() {
return this.deviceName;
}
public List filters() {
return this.filters == null ? List.of() : this.filters;
}
public String id() {
return this.id;
}
/**
* @return IP address of the gateway's Internet-routable external interface.
*
*/
public String ipAddress() {
return this.ipAddress;
}
/**
* @return Map of key-value pairs assigned to the gateway.
*
*/
public Map tags() {
return this.tags;
}
/**
* @return Type of customer gateway. The only type AWS supports at this time is "ipsec.1".
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetCustomerGatewayResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String arn;
private Integer bgpAsn;
private String certificateArn;
private String deviceName;
private @Nullable List filters;
private String id;
private String ipAddress;
private Map tags;
private String type;
public Builder() {}
public Builder(GetCustomerGatewayResult defaults) {
Objects.requireNonNull(defaults);
this.arn = defaults.arn;
this.bgpAsn = defaults.bgpAsn;
this.certificateArn = defaults.certificateArn;
this.deviceName = defaults.deviceName;
this.filters = defaults.filters;
this.id = defaults.id;
this.ipAddress = defaults.ipAddress;
this.tags = defaults.tags;
this.type = defaults.type;
}
@CustomType.Setter
public Builder arn(String arn) {
if (arn == null) {
throw new MissingRequiredPropertyException("GetCustomerGatewayResult", "arn");
}
this.arn = arn;
return this;
}
@CustomType.Setter
public Builder bgpAsn(Integer bgpAsn) {
if (bgpAsn == null) {
throw new MissingRequiredPropertyException("GetCustomerGatewayResult", "bgpAsn");
}
this.bgpAsn = bgpAsn;
return this;
}
@CustomType.Setter
public Builder certificateArn(String certificateArn) {
if (certificateArn == null) {
throw new MissingRequiredPropertyException("GetCustomerGatewayResult", "certificateArn");
}
this.certificateArn = certificateArn;
return this;
}
@CustomType.Setter
public Builder deviceName(String deviceName) {
if (deviceName == null) {
throw new MissingRequiredPropertyException("GetCustomerGatewayResult", "deviceName");
}
this.deviceName = deviceName;
return this;
}
@CustomType.Setter
public Builder filters(@Nullable List filters) {
this.filters = filters;
return this;
}
public Builder filters(GetCustomerGatewayFilter... filters) {
return filters(List.of(filters));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetCustomerGatewayResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder ipAddress(String ipAddress) {
if (ipAddress == null) {
throw new MissingRequiredPropertyException("GetCustomerGatewayResult", "ipAddress");
}
this.ipAddress = ipAddress;
return this;
}
@CustomType.Setter
public Builder tags(Map tags) {
if (tags == null) {
throw new MissingRequiredPropertyException("GetCustomerGatewayResult", "tags");
}
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetCustomerGatewayResult", "type");
}
this.type = type;
return this;
}
public GetCustomerGatewayResult build() {
final var _resultValue = new GetCustomerGatewayResult();
_resultValue.arn = arn;
_resultValue.bgpAsn = bgpAsn;
_resultValue.certificateArn = certificateArn;
_resultValue.deviceName = deviceName;
_resultValue.filters = filters;
_resultValue.id = id;
_resultValue.ipAddress = ipAddress;
_resultValue.tags = tags;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy