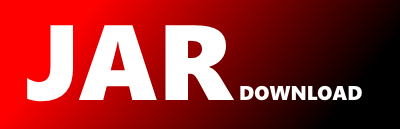
com.pulumi.aws.ec2.outputs.GetRouteTableRoute Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class GetRouteTableRoute {
/**
* @return ID of the Carrier Gateway.
*
*/
private String carrierGatewayId;
/**
* @return CIDR block of the route.
*
*/
private String cidrBlock;
/**
* @return ARN of the core network.
*
*/
private String coreNetworkArn;
/**
* @return The ID of a managed prefix list destination of the route.
*
*/
private String destinationPrefixListId;
/**
* @return ID of the Egress Only Internet Gateway.
*
*/
private String egressOnlyGatewayId;
/**
* @return ID of an Internet Gateway or Virtual Private Gateway which is connected to the Route Table (not exported if not passed as a parameter).
*
*/
private String gatewayId;
/**
* @return EC2 instance ID.
*
*/
private String instanceId;
/**
* @return IPv6 CIDR block of the route.
*
*/
private String ipv6CidrBlock;
/**
* @return Local Gateway ID.
*
*/
private String localGatewayId;
/**
* @return NAT Gateway ID.
*
*/
private String natGatewayId;
/**
* @return ID of the elastic network interface (eni) to use.
*
*/
private String networkInterfaceId;
/**
* @return EC2 Transit Gateway ID.
*
*/
private String transitGatewayId;
/**
* @return VPC Endpoint ID.
*
*/
private String vpcEndpointId;
/**
* @return VPC Peering ID.
*
*/
private String vpcPeeringConnectionId;
private GetRouteTableRoute() {}
/**
* @return ID of the Carrier Gateway.
*
*/
public String carrierGatewayId() {
return this.carrierGatewayId;
}
/**
* @return CIDR block of the route.
*
*/
public String cidrBlock() {
return this.cidrBlock;
}
/**
* @return ARN of the core network.
*
*/
public String coreNetworkArn() {
return this.coreNetworkArn;
}
/**
* @return The ID of a managed prefix list destination of the route.
*
*/
public String destinationPrefixListId() {
return this.destinationPrefixListId;
}
/**
* @return ID of the Egress Only Internet Gateway.
*
*/
public String egressOnlyGatewayId() {
return this.egressOnlyGatewayId;
}
/**
* @return ID of an Internet Gateway or Virtual Private Gateway which is connected to the Route Table (not exported if not passed as a parameter).
*
*/
public String gatewayId() {
return this.gatewayId;
}
/**
* @return EC2 instance ID.
*
*/
public String instanceId() {
return this.instanceId;
}
/**
* @return IPv6 CIDR block of the route.
*
*/
public String ipv6CidrBlock() {
return this.ipv6CidrBlock;
}
/**
* @return Local Gateway ID.
*
*/
public String localGatewayId() {
return this.localGatewayId;
}
/**
* @return NAT Gateway ID.
*
*/
public String natGatewayId() {
return this.natGatewayId;
}
/**
* @return ID of the elastic network interface (eni) to use.
*
*/
public String networkInterfaceId() {
return this.networkInterfaceId;
}
/**
* @return EC2 Transit Gateway ID.
*
*/
public String transitGatewayId() {
return this.transitGatewayId;
}
/**
* @return VPC Endpoint ID.
*
*/
public String vpcEndpointId() {
return this.vpcEndpointId;
}
/**
* @return VPC Peering ID.
*
*/
public String vpcPeeringConnectionId() {
return this.vpcPeeringConnectionId;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetRouteTableRoute defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String carrierGatewayId;
private String cidrBlock;
private String coreNetworkArn;
private String destinationPrefixListId;
private String egressOnlyGatewayId;
private String gatewayId;
private String instanceId;
private String ipv6CidrBlock;
private String localGatewayId;
private String natGatewayId;
private String networkInterfaceId;
private String transitGatewayId;
private String vpcEndpointId;
private String vpcPeeringConnectionId;
public Builder() {}
public Builder(GetRouteTableRoute defaults) {
Objects.requireNonNull(defaults);
this.carrierGatewayId = defaults.carrierGatewayId;
this.cidrBlock = defaults.cidrBlock;
this.coreNetworkArn = defaults.coreNetworkArn;
this.destinationPrefixListId = defaults.destinationPrefixListId;
this.egressOnlyGatewayId = defaults.egressOnlyGatewayId;
this.gatewayId = defaults.gatewayId;
this.instanceId = defaults.instanceId;
this.ipv6CidrBlock = defaults.ipv6CidrBlock;
this.localGatewayId = defaults.localGatewayId;
this.natGatewayId = defaults.natGatewayId;
this.networkInterfaceId = defaults.networkInterfaceId;
this.transitGatewayId = defaults.transitGatewayId;
this.vpcEndpointId = defaults.vpcEndpointId;
this.vpcPeeringConnectionId = defaults.vpcPeeringConnectionId;
}
@CustomType.Setter
public Builder carrierGatewayId(String carrierGatewayId) {
if (carrierGatewayId == null) {
throw new MissingRequiredPropertyException("GetRouteTableRoute", "carrierGatewayId");
}
this.carrierGatewayId = carrierGatewayId;
return this;
}
@CustomType.Setter
public Builder cidrBlock(String cidrBlock) {
if (cidrBlock == null) {
throw new MissingRequiredPropertyException("GetRouteTableRoute", "cidrBlock");
}
this.cidrBlock = cidrBlock;
return this;
}
@CustomType.Setter
public Builder coreNetworkArn(String coreNetworkArn) {
if (coreNetworkArn == null) {
throw new MissingRequiredPropertyException("GetRouteTableRoute", "coreNetworkArn");
}
this.coreNetworkArn = coreNetworkArn;
return this;
}
@CustomType.Setter
public Builder destinationPrefixListId(String destinationPrefixListId) {
if (destinationPrefixListId == null) {
throw new MissingRequiredPropertyException("GetRouteTableRoute", "destinationPrefixListId");
}
this.destinationPrefixListId = destinationPrefixListId;
return this;
}
@CustomType.Setter
public Builder egressOnlyGatewayId(String egressOnlyGatewayId) {
if (egressOnlyGatewayId == null) {
throw new MissingRequiredPropertyException("GetRouteTableRoute", "egressOnlyGatewayId");
}
this.egressOnlyGatewayId = egressOnlyGatewayId;
return this;
}
@CustomType.Setter
public Builder gatewayId(String gatewayId) {
if (gatewayId == null) {
throw new MissingRequiredPropertyException("GetRouteTableRoute", "gatewayId");
}
this.gatewayId = gatewayId;
return this;
}
@CustomType.Setter
public Builder instanceId(String instanceId) {
if (instanceId == null) {
throw new MissingRequiredPropertyException("GetRouteTableRoute", "instanceId");
}
this.instanceId = instanceId;
return this;
}
@CustomType.Setter
public Builder ipv6CidrBlock(String ipv6CidrBlock) {
if (ipv6CidrBlock == null) {
throw new MissingRequiredPropertyException("GetRouteTableRoute", "ipv6CidrBlock");
}
this.ipv6CidrBlock = ipv6CidrBlock;
return this;
}
@CustomType.Setter
public Builder localGatewayId(String localGatewayId) {
if (localGatewayId == null) {
throw new MissingRequiredPropertyException("GetRouteTableRoute", "localGatewayId");
}
this.localGatewayId = localGatewayId;
return this;
}
@CustomType.Setter
public Builder natGatewayId(String natGatewayId) {
if (natGatewayId == null) {
throw new MissingRequiredPropertyException("GetRouteTableRoute", "natGatewayId");
}
this.natGatewayId = natGatewayId;
return this;
}
@CustomType.Setter
public Builder networkInterfaceId(String networkInterfaceId) {
if (networkInterfaceId == null) {
throw new MissingRequiredPropertyException("GetRouteTableRoute", "networkInterfaceId");
}
this.networkInterfaceId = networkInterfaceId;
return this;
}
@CustomType.Setter
public Builder transitGatewayId(String transitGatewayId) {
if (transitGatewayId == null) {
throw new MissingRequiredPropertyException("GetRouteTableRoute", "transitGatewayId");
}
this.transitGatewayId = transitGatewayId;
return this;
}
@CustomType.Setter
public Builder vpcEndpointId(String vpcEndpointId) {
if (vpcEndpointId == null) {
throw new MissingRequiredPropertyException("GetRouteTableRoute", "vpcEndpointId");
}
this.vpcEndpointId = vpcEndpointId;
return this;
}
@CustomType.Setter
public Builder vpcPeeringConnectionId(String vpcPeeringConnectionId) {
if (vpcPeeringConnectionId == null) {
throw new MissingRequiredPropertyException("GetRouteTableRoute", "vpcPeeringConnectionId");
}
this.vpcPeeringConnectionId = vpcPeeringConnectionId;
return this;
}
public GetRouteTableRoute build() {
final var _resultValue = new GetRouteTableRoute();
_resultValue.carrierGatewayId = carrierGatewayId;
_resultValue.cidrBlock = cidrBlock;
_resultValue.coreNetworkArn = coreNetworkArn;
_resultValue.destinationPrefixListId = destinationPrefixListId;
_resultValue.egressOnlyGatewayId = egressOnlyGatewayId;
_resultValue.gatewayId = gatewayId;
_resultValue.instanceId = instanceId;
_resultValue.ipv6CidrBlock = ipv6CidrBlock;
_resultValue.localGatewayId = localGatewayId;
_resultValue.natGatewayId = natGatewayId;
_resultValue.networkInterfaceId = networkInterfaceId;
_resultValue.transitGatewayId = transitGatewayId;
_resultValue.vpcEndpointId = vpcEndpointId;
_resultValue.vpcPeeringConnectionId = vpcPeeringConnectionId;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy