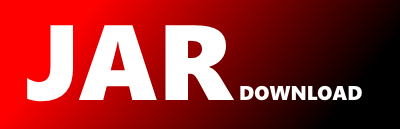
com.pulumi.aws.ec2transitgateway.InstanceState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2transitgateway;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.ec2transitgateway.InstanceStateArgs;
import com.pulumi.aws.ec2transitgateway.inputs.InstanceStateState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides an EC2 instance state resource. This allows managing an instance power state.
*
* > **NOTE on Instance State Management:** AWS does not currently have an EC2 API operation to determine an instance has finished processing user data. As a result, this resource can interfere with user data processing. For example, this resource may stop an instance while the user data script is in mid run.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ec2.Ec2Functions;
* import com.pulumi.aws.ec2.inputs.GetAmiArgs;
* import com.pulumi.aws.ec2.Instance;
* import com.pulumi.aws.ec2.InstanceArgs;
* import com.pulumi.aws.ec2transitgateway.InstanceState;
* import com.pulumi.aws.ec2transitgateway.InstanceStateArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* final var ubuntu = Ec2Functions.getAmi(GetAmiArgs.builder()
* .mostRecent(true)
* .filters(
* GetAmiFilterArgs.builder()
* .name("name")
* .values("ubuntu/images/hvm-ssd/ubuntu-focal-20.04-amd64-server-*")
* .build(),
* GetAmiFilterArgs.builder()
* .name("virtualization-type")
* .values("hvm")
* .build())
* .owners("099720109477")
* .build());
*
* var test = new Instance("test", InstanceArgs.builder()
* .ami(ubuntu.applyValue(getAmiResult -> getAmiResult.id()))
* .instanceType("t3.micro")
* .tags(Map.of("Name", "HelloWorld"))
* .build());
*
* var testInstanceState = new InstanceState("testInstanceState", InstanceStateArgs.builder()
* .instanceId(test.id())
* .state("stopped")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import `aws_ec2_instance_state` using the `instance_id` attribute. For example:
*
* ```sh
* $ pulumi import aws:ec2transitgateway/instanceState:InstanceState test i-02cae6557dfcf2f96
* ```
*
*/
@ResourceType(type="aws:ec2transitgateway/instanceState:InstanceState")
public class InstanceState extends com.pulumi.resources.CustomResource {
/**
* Whether to request a forced stop when `state` is `stopped`. Otherwise (_i.e._, `state` is `running`), ignored. When an instance is forced to stop, it does not flush file system caches or file system metadata, and you must subsequently perform file system check and repair. Not recommended for Windows instances. Defaults to `false`.
*
*/
@Export(name="force", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> force;
/**
* @return Whether to request a forced stop when `state` is `stopped`. Otherwise (_i.e._, `state` is `running`), ignored. When an instance is forced to stop, it does not flush file system caches or file system metadata, and you must subsequently perform file system check and repair. Not recommended for Windows instances. Defaults to `false`.
*
*/
public Output> force() {
return Codegen.optional(this.force);
}
/**
* ID of the instance.
*
*/
@Export(name="instanceId", refs={String.class}, tree="[0]")
private Output instanceId;
/**
* @return ID of the instance.
*
*/
public Output instanceId() {
return this.instanceId;
}
/**
* State of the instance. Valid values are `stopped`, `running`.
*
* The following arguments are optional:
*
*/
@Export(name="state", refs={String.class}, tree="[0]")
private Output state;
/**
* @return State of the instance. Valid values are `stopped`, `running`.
*
* The following arguments are optional:
*
*/
public Output state() {
return this.state;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public InstanceState(String name) {
this(name, InstanceStateArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public InstanceState(String name, InstanceStateArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public InstanceState(String name, InstanceStateArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:ec2transitgateway/instanceState:InstanceState", name, args == null ? InstanceStateArgs.Empty : args, makeResourceOptions(options, Codegen.empty()));
}
private InstanceState(String name, Output id, @Nullable InstanceStateState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:ec2transitgateway/instanceState:InstanceState", name, state, makeResourceOptions(options, id));
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static InstanceState get(String name, Output id, @Nullable InstanceStateState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new InstanceState(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy