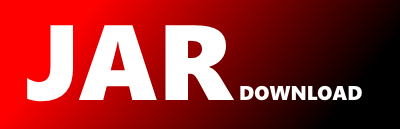
com.pulumi.aws.ecr.outputs.GetRepositoryResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ecr.outputs;
import com.pulumi.aws.ecr.outputs.GetRepositoryEncryptionConfiguration;
import com.pulumi.aws.ecr.outputs.GetRepositoryImageScanningConfiguration;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class GetRepositoryResult {
/**
* @return Full ARN of the repository.
*
*/
private String arn;
/**
* @return Encryption configuration for the repository. See Encryption Configuration below.
*
*/
private List encryptionConfigurations;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
/**
* @return Configuration block that defines image scanning configuration for the repository. See Image Scanning Configuration below.
*
*/
private List imageScanningConfigurations;
/**
* @return The tag mutability setting for the repository.
*
*/
private String imageTagMutability;
/**
* @return List of image tags associated with the most recently pushed image in the repository.
*
*/
private List mostRecentImageTags;
private String name;
private String registryId;
/**
* @return URL of the repository (in the form `aws_account_id.dkr.ecr.region.amazonaws.com/repositoryName`).
*
*/
private String repositoryUrl;
/**
* @return Map of tags assigned to the resource.
*
*/
private Map tags;
private GetRepositoryResult() {}
/**
* @return Full ARN of the repository.
*
*/
public String arn() {
return this.arn;
}
/**
* @return Encryption configuration for the repository. See Encryption Configuration below.
*
*/
public List encryptionConfigurations() {
return this.encryptionConfigurations;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
/**
* @return Configuration block that defines image scanning configuration for the repository. See Image Scanning Configuration below.
*
*/
public List imageScanningConfigurations() {
return this.imageScanningConfigurations;
}
/**
* @return The tag mutability setting for the repository.
*
*/
public String imageTagMutability() {
return this.imageTagMutability;
}
/**
* @return List of image tags associated with the most recently pushed image in the repository.
*
*/
public List mostRecentImageTags() {
return this.mostRecentImageTags;
}
public String name() {
return this.name;
}
public String registryId() {
return this.registryId;
}
/**
* @return URL of the repository (in the form `aws_account_id.dkr.ecr.region.amazonaws.com/repositoryName`).
*
*/
public String repositoryUrl() {
return this.repositoryUrl;
}
/**
* @return Map of tags assigned to the resource.
*
*/
public Map tags() {
return this.tags;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetRepositoryResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String arn;
private List encryptionConfigurations;
private String id;
private List imageScanningConfigurations;
private String imageTagMutability;
private List mostRecentImageTags;
private String name;
private String registryId;
private String repositoryUrl;
private Map tags;
public Builder() {}
public Builder(GetRepositoryResult defaults) {
Objects.requireNonNull(defaults);
this.arn = defaults.arn;
this.encryptionConfigurations = defaults.encryptionConfigurations;
this.id = defaults.id;
this.imageScanningConfigurations = defaults.imageScanningConfigurations;
this.imageTagMutability = defaults.imageTagMutability;
this.mostRecentImageTags = defaults.mostRecentImageTags;
this.name = defaults.name;
this.registryId = defaults.registryId;
this.repositoryUrl = defaults.repositoryUrl;
this.tags = defaults.tags;
}
@CustomType.Setter
public Builder arn(String arn) {
if (arn == null) {
throw new MissingRequiredPropertyException("GetRepositoryResult", "arn");
}
this.arn = arn;
return this;
}
@CustomType.Setter
public Builder encryptionConfigurations(List encryptionConfigurations) {
if (encryptionConfigurations == null) {
throw new MissingRequiredPropertyException("GetRepositoryResult", "encryptionConfigurations");
}
this.encryptionConfigurations = encryptionConfigurations;
return this;
}
public Builder encryptionConfigurations(GetRepositoryEncryptionConfiguration... encryptionConfigurations) {
return encryptionConfigurations(List.of(encryptionConfigurations));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetRepositoryResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder imageScanningConfigurations(List imageScanningConfigurations) {
if (imageScanningConfigurations == null) {
throw new MissingRequiredPropertyException("GetRepositoryResult", "imageScanningConfigurations");
}
this.imageScanningConfigurations = imageScanningConfigurations;
return this;
}
public Builder imageScanningConfigurations(GetRepositoryImageScanningConfiguration... imageScanningConfigurations) {
return imageScanningConfigurations(List.of(imageScanningConfigurations));
}
@CustomType.Setter
public Builder imageTagMutability(String imageTagMutability) {
if (imageTagMutability == null) {
throw new MissingRequiredPropertyException("GetRepositoryResult", "imageTagMutability");
}
this.imageTagMutability = imageTagMutability;
return this;
}
@CustomType.Setter
public Builder mostRecentImageTags(List mostRecentImageTags) {
if (mostRecentImageTags == null) {
throw new MissingRequiredPropertyException("GetRepositoryResult", "mostRecentImageTags");
}
this.mostRecentImageTags = mostRecentImageTags;
return this;
}
public Builder mostRecentImageTags(String... mostRecentImageTags) {
return mostRecentImageTags(List.of(mostRecentImageTags));
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetRepositoryResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder registryId(String registryId) {
if (registryId == null) {
throw new MissingRequiredPropertyException("GetRepositoryResult", "registryId");
}
this.registryId = registryId;
return this;
}
@CustomType.Setter
public Builder repositoryUrl(String repositoryUrl) {
if (repositoryUrl == null) {
throw new MissingRequiredPropertyException("GetRepositoryResult", "repositoryUrl");
}
this.repositoryUrl = repositoryUrl;
return this;
}
@CustomType.Setter
public Builder tags(Map tags) {
if (tags == null) {
throw new MissingRequiredPropertyException("GetRepositoryResult", "tags");
}
this.tags = tags;
return this;
}
public GetRepositoryResult build() {
final var _resultValue = new GetRepositoryResult();
_resultValue.arn = arn;
_resultValue.encryptionConfigurations = encryptionConfigurations;
_resultValue.id = id;
_resultValue.imageScanningConfigurations = imageScanningConfigurations;
_resultValue.imageTagMutability = imageTagMutability;
_resultValue.mostRecentImageTags = mostRecentImageTags;
_resultValue.name = name;
_resultValue.registryId = registryId;
_resultValue.repositoryUrl = repositoryUrl;
_resultValue.tags = tags;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy