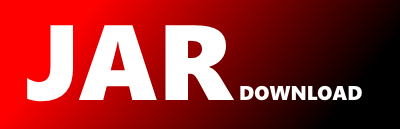
com.pulumi.aws.elastictranscoder.inputs.PresetVideoWatermarkArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.elastictranscoder.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class PresetVideoWatermarkArgs extends com.pulumi.resources.ResourceArgs {
public static final PresetVideoWatermarkArgs Empty = new PresetVideoWatermarkArgs();
/**
* The horizontal position of the watermark unless you specify a nonzero value for `horzontal_offset`.
*
*/
@Import(name="horizontalAlign")
private @Nullable Output horizontalAlign;
/**
* @return The horizontal position of the watermark unless you specify a nonzero value for `horzontal_offset`.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy