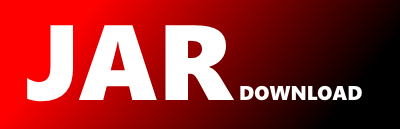
com.pulumi.aws.glacier.VaultLock Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.glacier;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.glacier.VaultLockArgs;
import com.pulumi.aws.glacier.inputs.VaultLockState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* ## Example Usage
*
* ### Testing Glacier Vault Lock Policy
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.glacier.Vault;
* import com.pulumi.aws.glacier.VaultArgs;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyDocumentArgs;
* import com.pulumi.aws.glacier.VaultLock;
* import com.pulumi.aws.glacier.VaultLockArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var exampleVault = new Vault("exampleVault", VaultArgs.builder()
* .name("example")
* .build());
*
* final var example = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .actions("glacier:DeleteArchive")
* .effect("Deny")
* .resources(exampleVault.arn())
* .conditions(GetPolicyDocumentStatementConditionArgs.builder()
* .test("NumericLessThanEquals")
* .variable("glacier:ArchiveAgeinDays")
* .values("365")
* .build())
* .build())
* .build());
*
* var exampleVaultLock = new VaultLock("exampleVaultLock", VaultLockArgs.builder()
* .completeLock(false)
* .policy(example.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult).applyValue(example -> example.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json())))
* .vaultName(exampleVault.name())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Permanently Applying Glacier Vault Lock Policy
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.glacier.VaultLock;
* import com.pulumi.aws.glacier.VaultLockArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new VaultLock("example", VaultLockArgs.builder()
* .completeLock(true)
* .policy(exampleAwsIamPolicyDocument.json())
* .vaultName(exampleAwsGlacierVault.name())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import Glacier Vault Locks using the Glacier Vault name. For example:
*
* ```sh
* $ pulumi import aws:glacier/vaultLock:VaultLock example example-vault
* ```
*
*/
@ResourceType(type="aws:glacier/vaultLock:VaultLock")
public class VaultLock extends com.pulumi.resources.CustomResource {
/**
* Boolean whether to permanently apply this Glacier Lock Policy. Once completed, this cannot be undone. If set to `false`, the Glacier Lock Policy remains in a testing mode for 24 hours. After that time, the Glacier Lock Policy is automatically removed by Glacier and the this provider resource will show as needing recreation. Changing this from `false` to `true` will show as resource recreation, which is expected. Changing this from `true` to `false` is not possible unless the Glacier Vault is recreated at the same time.
*
*/
@Export(name="completeLock", refs={Boolean.class}, tree="[0]")
private Output completeLock;
/**
* @return Boolean whether to permanently apply this Glacier Lock Policy. Once completed, this cannot be undone. If set to `false`, the Glacier Lock Policy remains in a testing mode for 24 hours. After that time, the Glacier Lock Policy is automatically removed by Glacier and the this provider resource will show as needing recreation. Changing this from `false` to `true` will show as resource recreation, which is expected. Changing this from `true` to `false` is not possible unless the Glacier Vault is recreated at the same time.
*
*/
public Output completeLock() {
return this.completeLock;
}
/**
* Allow this provider to ignore the error returned when attempting to delete the Glacier Lock Policy. This can be used to delete or recreate the Glacier Vault via this provider, for example, if the Glacier Vault Lock policy permits that action. This should only be used in conjunction with `complete_lock` being set to `true`.
*
*/
@Export(name="ignoreDeletionError", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> ignoreDeletionError;
/**
* @return Allow this provider to ignore the error returned when attempting to delete the Glacier Lock Policy. This can be used to delete or recreate the Glacier Vault via this provider, for example, if the Glacier Vault Lock policy permits that action. This should only be used in conjunction with `complete_lock` being set to `true`.
*
*/
public Output> ignoreDeletionError() {
return Codegen.optional(this.ignoreDeletionError);
}
/**
* JSON string containing the IAM policy to apply as the Glacier Vault Lock policy.
*
*/
@Export(name="policy", refs={String.class}, tree="[0]")
private Output policy;
/**
* @return JSON string containing the IAM policy to apply as the Glacier Vault Lock policy.
*
*/
public Output policy() {
return this.policy;
}
/**
* The name of the Glacier Vault.
*
*/
@Export(name="vaultName", refs={String.class}, tree="[0]")
private Output vaultName;
/**
* @return The name of the Glacier Vault.
*
*/
public Output vaultName() {
return this.vaultName;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public VaultLock(String name) {
this(name, VaultLockArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public VaultLock(String name, VaultLockArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public VaultLock(String name, VaultLockArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:glacier/vaultLock:VaultLock", name, args == null ? VaultLockArgs.Empty : args, makeResourceOptions(options, Codegen.empty()));
}
private VaultLock(String name, Output id, @Nullable VaultLockState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:glacier/vaultLock:VaultLock", name, state, makeResourceOptions(options, id));
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static VaultLock get(String name, Output id, @Nullable VaultLockState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new VaultLock(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy