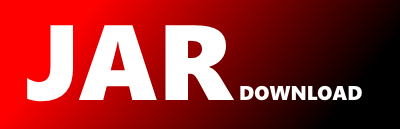
com.pulumi.aws.lambda.outputs.FunctionUrlCors Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.lambda.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class FunctionUrlCors {
/**
* @return Whether to allow cookies or other credentials in requests to the function URL. The default is `false`.
*
*/
private @Nullable Boolean allowCredentials;
/**
* @return The HTTP headers that origins can include in requests to the function URL. For example: `["date", "keep-alive", "x-custom-header"]`.
*
*/
private @Nullable List allowHeaders;
/**
* @return The HTTP methods that are allowed when calling the function URL. For example: `["GET", "POST", "DELETE"]`, or the wildcard character (`["*"]`).
*
*/
private @Nullable List allowMethods;
/**
* @return The origins that can access the function URL. You can list any number of specific origins (or the wildcard character (`"*"`)), separated by a comma. For example: `["https://www.example.com", "http://localhost:60905"]`.
*
*/
private @Nullable List allowOrigins;
/**
* @return The HTTP headers in your function response that you want to expose to origins that call the function URL.
*
*/
private @Nullable List exposeHeaders;
/**
* @return The maximum amount of time, in seconds, that web browsers can cache results of a preflight request. By default, this is set to `0`, which means that the browser doesn't cache results. The maximum value is `86400`.
*
*/
private @Nullable Integer maxAge;
private FunctionUrlCors() {}
/**
* @return Whether to allow cookies or other credentials in requests to the function URL. The default is `false`.
*
*/
public Optional allowCredentials() {
return Optional.ofNullable(this.allowCredentials);
}
/**
* @return The HTTP headers that origins can include in requests to the function URL. For example: `["date", "keep-alive", "x-custom-header"]`.
*
*/
public List allowHeaders() {
return this.allowHeaders == null ? List.of() : this.allowHeaders;
}
/**
* @return The HTTP methods that are allowed when calling the function URL. For example: `["GET", "POST", "DELETE"]`, or the wildcard character (`["*"]`).
*
*/
public List allowMethods() {
return this.allowMethods == null ? List.of() : this.allowMethods;
}
/**
* @return The origins that can access the function URL. You can list any number of specific origins (or the wildcard character (`"*"`)), separated by a comma. For example: `["https://www.example.com", "http://localhost:60905"]`.
*
*/
public List allowOrigins() {
return this.allowOrigins == null ? List.of() : this.allowOrigins;
}
/**
* @return The HTTP headers in your function response that you want to expose to origins that call the function URL.
*
*/
public List exposeHeaders() {
return this.exposeHeaders == null ? List.of() : this.exposeHeaders;
}
/**
* @return The maximum amount of time, in seconds, that web browsers can cache results of a preflight request. By default, this is set to `0`, which means that the browser doesn't cache results. The maximum value is `86400`.
*
*/
public Optional maxAge() {
return Optional.ofNullable(this.maxAge);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(FunctionUrlCors defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean allowCredentials;
private @Nullable List allowHeaders;
private @Nullable List allowMethods;
private @Nullable List allowOrigins;
private @Nullable List exposeHeaders;
private @Nullable Integer maxAge;
public Builder() {}
public Builder(FunctionUrlCors defaults) {
Objects.requireNonNull(defaults);
this.allowCredentials = defaults.allowCredentials;
this.allowHeaders = defaults.allowHeaders;
this.allowMethods = defaults.allowMethods;
this.allowOrigins = defaults.allowOrigins;
this.exposeHeaders = defaults.exposeHeaders;
this.maxAge = defaults.maxAge;
}
@CustomType.Setter
public Builder allowCredentials(@Nullable Boolean allowCredentials) {
this.allowCredentials = allowCredentials;
return this;
}
@CustomType.Setter
public Builder allowHeaders(@Nullable List allowHeaders) {
this.allowHeaders = allowHeaders;
return this;
}
public Builder allowHeaders(String... allowHeaders) {
return allowHeaders(List.of(allowHeaders));
}
@CustomType.Setter
public Builder allowMethods(@Nullable List allowMethods) {
this.allowMethods = allowMethods;
return this;
}
public Builder allowMethods(String... allowMethods) {
return allowMethods(List.of(allowMethods));
}
@CustomType.Setter
public Builder allowOrigins(@Nullable List allowOrigins) {
this.allowOrigins = allowOrigins;
return this;
}
public Builder allowOrigins(String... allowOrigins) {
return allowOrigins(List.of(allowOrigins));
}
@CustomType.Setter
public Builder exposeHeaders(@Nullable List exposeHeaders) {
this.exposeHeaders = exposeHeaders;
return this;
}
public Builder exposeHeaders(String... exposeHeaders) {
return exposeHeaders(List.of(exposeHeaders));
}
@CustomType.Setter
public Builder maxAge(@Nullable Integer maxAge) {
this.maxAge = maxAge;
return this;
}
public FunctionUrlCors build() {
final var _resultValue = new FunctionUrlCors();
_resultValue.allowCredentials = allowCredentials;
_resultValue.allowHeaders = allowHeaders;
_resultValue.allowMethods = allowMethods;
_resultValue.allowOrigins = allowOrigins;
_resultValue.exposeHeaders = exposeHeaders;
_resultValue.maxAge = maxAge;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy