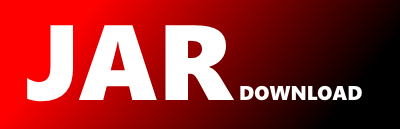
com.pulumi.aws.lightsail.inputs.DistributionState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.lightsail.inputs;
import com.pulumi.aws.lightsail.inputs.DistributionCacheBehaviorArgs;
import com.pulumi.aws.lightsail.inputs.DistributionCacheBehaviorSettingsArgs;
import com.pulumi.aws.lightsail.inputs.DistributionDefaultCacheBehaviorArgs;
import com.pulumi.aws.lightsail.inputs.DistributionLocationArgs;
import com.pulumi.aws.lightsail.inputs.DistributionOriginArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class DistributionState extends com.pulumi.resources.ResourceArgs {
public static final DistributionState Empty = new DistributionState();
/**
* The alternate domain names of the distribution.
*
*/
@Import(name="alternativeDomainNames")
private @Nullable Output> alternativeDomainNames;
/**
* @return The alternate domain names of the distribution.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy