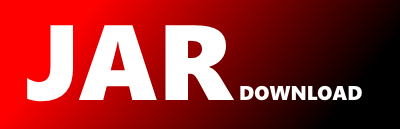
com.pulumi.aws.memorydb.outputs.SnapshotClusterConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.memorydb.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class SnapshotClusterConfiguration {
/**
* @return Description for the cluster.
*
*/
private @Nullable String description;
/**
* @return Version number of the Redis engine used by the cluster.
*
*/
private @Nullable String engineVersion;
/**
* @return The weekly time range during which maintenance on the cluster is performed.
*
*/
private @Nullable String maintenanceWindow;
/**
* @return Name of the snapshot. If omitted, the provider will assign a random, unique name. Conflicts with `name_prefix`.
*
*/
private @Nullable String name;
/**
* @return Compute and memory capacity of the nodes in the cluster.
*
*/
private @Nullable String nodeType;
/**
* @return Number of shards in the cluster.
*
*/
private @Nullable Integer numShards;
/**
* @return Name of the parameter group associated with the cluster.
*
*/
private @Nullable String parameterGroupName;
/**
* @return Port number on which the cluster accepts connections.
*
*/
private @Nullable Integer port;
/**
* @return Number of days for which MemoryDB retains automatic snapshots before deleting them.
*
*/
private @Nullable Integer snapshotRetentionLimit;
/**
* @return The daily time range (in UTC) during which MemoryDB begins taking a daily snapshot of the shard.
*
*/
private @Nullable String snapshotWindow;
/**
* @return Name of the subnet group used by the cluster.
*
*/
private @Nullable String subnetGroupName;
/**
* @return ARN of the SNS topic to which cluster notifications are sent.
*
*/
private @Nullable String topicArn;
/**
* @return The VPC in which the cluster exists.
*
*/
private @Nullable String vpcId;
private SnapshotClusterConfiguration() {}
/**
* @return Description for the cluster.
*
*/
public Optional description() {
return Optional.ofNullable(this.description);
}
/**
* @return Version number of the Redis engine used by the cluster.
*
*/
public Optional engineVersion() {
return Optional.ofNullable(this.engineVersion);
}
/**
* @return The weekly time range during which maintenance on the cluster is performed.
*
*/
public Optional maintenanceWindow() {
return Optional.ofNullable(this.maintenanceWindow);
}
/**
* @return Name of the snapshot. If omitted, the provider will assign a random, unique name. Conflicts with `name_prefix`.
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
/**
* @return Compute and memory capacity of the nodes in the cluster.
*
*/
public Optional nodeType() {
return Optional.ofNullable(this.nodeType);
}
/**
* @return Number of shards in the cluster.
*
*/
public Optional numShards() {
return Optional.ofNullable(this.numShards);
}
/**
* @return Name of the parameter group associated with the cluster.
*
*/
public Optional parameterGroupName() {
return Optional.ofNullable(this.parameterGroupName);
}
/**
* @return Port number on which the cluster accepts connections.
*
*/
public Optional port() {
return Optional.ofNullable(this.port);
}
/**
* @return Number of days for which MemoryDB retains automatic snapshots before deleting them.
*
*/
public Optional snapshotRetentionLimit() {
return Optional.ofNullable(this.snapshotRetentionLimit);
}
/**
* @return The daily time range (in UTC) during which MemoryDB begins taking a daily snapshot of the shard.
*
*/
public Optional snapshotWindow() {
return Optional.ofNullable(this.snapshotWindow);
}
/**
* @return Name of the subnet group used by the cluster.
*
*/
public Optional subnetGroupName() {
return Optional.ofNullable(this.subnetGroupName);
}
/**
* @return ARN of the SNS topic to which cluster notifications are sent.
*
*/
public Optional topicArn() {
return Optional.ofNullable(this.topicArn);
}
/**
* @return The VPC in which the cluster exists.
*
*/
public Optional vpcId() {
return Optional.ofNullable(this.vpcId);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(SnapshotClusterConfiguration defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String description;
private @Nullable String engineVersion;
private @Nullable String maintenanceWindow;
private @Nullable String name;
private @Nullable String nodeType;
private @Nullable Integer numShards;
private @Nullable String parameterGroupName;
private @Nullable Integer port;
private @Nullable Integer snapshotRetentionLimit;
private @Nullable String snapshotWindow;
private @Nullable String subnetGroupName;
private @Nullable String topicArn;
private @Nullable String vpcId;
public Builder() {}
public Builder(SnapshotClusterConfiguration defaults) {
Objects.requireNonNull(defaults);
this.description = defaults.description;
this.engineVersion = defaults.engineVersion;
this.maintenanceWindow = defaults.maintenanceWindow;
this.name = defaults.name;
this.nodeType = defaults.nodeType;
this.numShards = defaults.numShards;
this.parameterGroupName = defaults.parameterGroupName;
this.port = defaults.port;
this.snapshotRetentionLimit = defaults.snapshotRetentionLimit;
this.snapshotWindow = defaults.snapshotWindow;
this.subnetGroupName = defaults.subnetGroupName;
this.topicArn = defaults.topicArn;
this.vpcId = defaults.vpcId;
}
@CustomType.Setter
public Builder description(@Nullable String description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder engineVersion(@Nullable String engineVersion) {
this.engineVersion = engineVersion;
return this;
}
@CustomType.Setter
public Builder maintenanceWindow(@Nullable String maintenanceWindow) {
this.maintenanceWindow = maintenanceWindow;
return this;
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
@CustomType.Setter
public Builder nodeType(@Nullable String nodeType) {
this.nodeType = nodeType;
return this;
}
@CustomType.Setter
public Builder numShards(@Nullable Integer numShards) {
this.numShards = numShards;
return this;
}
@CustomType.Setter
public Builder parameterGroupName(@Nullable String parameterGroupName) {
this.parameterGroupName = parameterGroupName;
return this;
}
@CustomType.Setter
public Builder port(@Nullable Integer port) {
this.port = port;
return this;
}
@CustomType.Setter
public Builder snapshotRetentionLimit(@Nullable Integer snapshotRetentionLimit) {
this.snapshotRetentionLimit = snapshotRetentionLimit;
return this;
}
@CustomType.Setter
public Builder snapshotWindow(@Nullable String snapshotWindow) {
this.snapshotWindow = snapshotWindow;
return this;
}
@CustomType.Setter
public Builder subnetGroupName(@Nullable String subnetGroupName) {
this.subnetGroupName = subnetGroupName;
return this;
}
@CustomType.Setter
public Builder topicArn(@Nullable String topicArn) {
this.topicArn = topicArn;
return this;
}
@CustomType.Setter
public Builder vpcId(@Nullable String vpcId) {
this.vpcId = vpcId;
return this;
}
public SnapshotClusterConfiguration build() {
final var _resultValue = new SnapshotClusterConfiguration();
_resultValue.description = description;
_resultValue.engineVersion = engineVersion;
_resultValue.maintenanceWindow = maintenanceWindow;
_resultValue.name = name;
_resultValue.nodeType = nodeType;
_resultValue.numShards = numShards;
_resultValue.parameterGroupName = parameterGroupName;
_resultValue.port = port;
_resultValue.snapshotRetentionLimit = snapshotRetentionLimit;
_resultValue.snapshotWindow = snapshotWindow;
_resultValue.subnetGroupName = subnetGroupName;
_resultValue.topicArn = topicArn;
_resultValue.vpcId = vpcId;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy