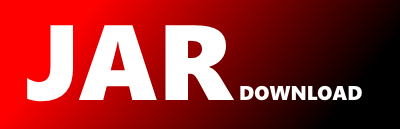
com.pulumi.aws.networkfirewall.outputs.FirewallPolicyFirewallPolicy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.networkfirewall.outputs;
import com.pulumi.aws.networkfirewall.outputs.FirewallPolicyFirewallPolicyPolicyVariables;
import com.pulumi.aws.networkfirewall.outputs.FirewallPolicyFirewallPolicyStatefulEngineOptions;
import com.pulumi.aws.networkfirewall.outputs.FirewallPolicyFirewallPolicyStatefulRuleGroupReference;
import com.pulumi.aws.networkfirewall.outputs.FirewallPolicyFirewallPolicyStatelessCustomAction;
import com.pulumi.aws.networkfirewall.outputs.FirewallPolicyFirewallPolicyStatelessRuleGroupReference;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class FirewallPolicyFirewallPolicy {
/**
* @return . Contains variables that you can use to override default Suricata settings in your firewall policy. See Rule Variables for details.
*
*/
private @Nullable FirewallPolicyFirewallPolicyPolicyVariables policyVariables;
/**
* @return Set of actions to take on a packet if it does not match any stateful rules in the policy. This can only be specified if the policy has a `stateful_engine_options` block with a `rule_order` value of `STRICT_ORDER`. You can specify one of either or neither values of `aws:drop_strict` or `aws:drop_established`, as well as any combination of `aws:alert_strict` and `aws:alert_established`.
*
*/
private @Nullable List statefulDefaultActions;
/**
* @return A configuration block that defines options on how the policy handles stateful rules. See Stateful Engine Options below for details.
*
*/
private @Nullable FirewallPolicyFirewallPolicyStatefulEngineOptions statefulEngineOptions;
/**
* @return Set of configuration blocks containing references to the stateful rule groups that are used in the policy. See Stateful Rule Group Reference below for details.
*
*/
private @Nullable List statefulRuleGroupReferences;
/**
* @return Set of configuration blocks describing the custom action definitions that are available for use in the firewall policy's `stateless_default_actions`. See Stateless Custom Action below for details.
*
*/
private @Nullable List statelessCustomActions;
/**
* @return Set of actions to take on a packet if it does not match any of the stateless rules in the policy. You must specify one of the standard actions including: `aws:drop`, `aws:pass`, or `aws:forward_to_sfe`.
* In addition, you can specify custom actions that are compatible with your standard action choice. If you want non-matching packets to be forwarded for stateful inspection, specify `aws:forward_to_sfe`.
*
*/
private List statelessDefaultActions;
/**
* @return Set of actions to take on a fragmented packet if it does not match any of the stateless rules in the policy. You must specify one of the standard actions including: `aws:drop`, `aws:pass`, or `aws:forward_to_sfe`.
* In addition, you can specify custom actions that are compatible with your standard action choice. If you want non-matching packets to be forwarded for stateful inspection, specify `aws:forward_to_sfe`.
*
*/
private List statelessFragmentDefaultActions;
/**
* @return Set of configuration blocks containing references to the stateless rule groups that are used in the policy. See Stateless Rule Group Reference below for details.
*
*/
private @Nullable List statelessRuleGroupReferences;
/**
* @return The (ARN) of the TLS Inspection policy to attach to the FW Policy. This must be added at creation of the resource per AWS documentation. "You can only add a TLS inspection configuration to a new policy, not to an existing policy." This cannot be removed from a FW Policy.
*
*/
private @Nullable String tlsInspectionConfigurationArn;
private FirewallPolicyFirewallPolicy() {}
/**
* @return . Contains variables that you can use to override default Suricata settings in your firewall policy. See Rule Variables for details.
*
*/
public Optional policyVariables() {
return Optional.ofNullable(this.policyVariables);
}
/**
* @return Set of actions to take on a packet if it does not match any stateful rules in the policy. This can only be specified if the policy has a `stateful_engine_options` block with a `rule_order` value of `STRICT_ORDER`. You can specify one of either or neither values of `aws:drop_strict` or `aws:drop_established`, as well as any combination of `aws:alert_strict` and `aws:alert_established`.
*
*/
public List statefulDefaultActions() {
return this.statefulDefaultActions == null ? List.of() : this.statefulDefaultActions;
}
/**
* @return A configuration block that defines options on how the policy handles stateful rules. See Stateful Engine Options below for details.
*
*/
public Optional statefulEngineOptions() {
return Optional.ofNullable(this.statefulEngineOptions);
}
/**
* @return Set of configuration blocks containing references to the stateful rule groups that are used in the policy. See Stateful Rule Group Reference below for details.
*
*/
public List statefulRuleGroupReferences() {
return this.statefulRuleGroupReferences == null ? List.of() : this.statefulRuleGroupReferences;
}
/**
* @return Set of configuration blocks describing the custom action definitions that are available for use in the firewall policy's `stateless_default_actions`. See Stateless Custom Action below for details.
*
*/
public List statelessCustomActions() {
return this.statelessCustomActions == null ? List.of() : this.statelessCustomActions;
}
/**
* @return Set of actions to take on a packet if it does not match any of the stateless rules in the policy. You must specify one of the standard actions including: `aws:drop`, `aws:pass`, or `aws:forward_to_sfe`.
* In addition, you can specify custom actions that are compatible with your standard action choice. If you want non-matching packets to be forwarded for stateful inspection, specify `aws:forward_to_sfe`.
*
*/
public List statelessDefaultActions() {
return this.statelessDefaultActions;
}
/**
* @return Set of actions to take on a fragmented packet if it does not match any of the stateless rules in the policy. You must specify one of the standard actions including: `aws:drop`, `aws:pass`, or `aws:forward_to_sfe`.
* In addition, you can specify custom actions that are compatible with your standard action choice. If you want non-matching packets to be forwarded for stateful inspection, specify `aws:forward_to_sfe`.
*
*/
public List statelessFragmentDefaultActions() {
return this.statelessFragmentDefaultActions;
}
/**
* @return Set of configuration blocks containing references to the stateless rule groups that are used in the policy. See Stateless Rule Group Reference below for details.
*
*/
public List statelessRuleGroupReferences() {
return this.statelessRuleGroupReferences == null ? List.of() : this.statelessRuleGroupReferences;
}
/**
* @return The (ARN) of the TLS Inspection policy to attach to the FW Policy. This must be added at creation of the resource per AWS documentation. "You can only add a TLS inspection configuration to a new policy, not to an existing policy." This cannot be removed from a FW Policy.
*
*/
public Optional tlsInspectionConfigurationArn() {
return Optional.ofNullable(this.tlsInspectionConfigurationArn);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(FirewallPolicyFirewallPolicy defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable FirewallPolicyFirewallPolicyPolicyVariables policyVariables;
private @Nullable List statefulDefaultActions;
private @Nullable FirewallPolicyFirewallPolicyStatefulEngineOptions statefulEngineOptions;
private @Nullable List statefulRuleGroupReferences;
private @Nullable List statelessCustomActions;
private List statelessDefaultActions;
private List statelessFragmentDefaultActions;
private @Nullable List statelessRuleGroupReferences;
private @Nullable String tlsInspectionConfigurationArn;
public Builder() {}
public Builder(FirewallPolicyFirewallPolicy defaults) {
Objects.requireNonNull(defaults);
this.policyVariables = defaults.policyVariables;
this.statefulDefaultActions = defaults.statefulDefaultActions;
this.statefulEngineOptions = defaults.statefulEngineOptions;
this.statefulRuleGroupReferences = defaults.statefulRuleGroupReferences;
this.statelessCustomActions = defaults.statelessCustomActions;
this.statelessDefaultActions = defaults.statelessDefaultActions;
this.statelessFragmentDefaultActions = defaults.statelessFragmentDefaultActions;
this.statelessRuleGroupReferences = defaults.statelessRuleGroupReferences;
this.tlsInspectionConfigurationArn = defaults.tlsInspectionConfigurationArn;
}
@CustomType.Setter
public Builder policyVariables(@Nullable FirewallPolicyFirewallPolicyPolicyVariables policyVariables) {
this.policyVariables = policyVariables;
return this;
}
@CustomType.Setter
public Builder statefulDefaultActions(@Nullable List statefulDefaultActions) {
this.statefulDefaultActions = statefulDefaultActions;
return this;
}
public Builder statefulDefaultActions(String... statefulDefaultActions) {
return statefulDefaultActions(List.of(statefulDefaultActions));
}
@CustomType.Setter
public Builder statefulEngineOptions(@Nullable FirewallPolicyFirewallPolicyStatefulEngineOptions statefulEngineOptions) {
this.statefulEngineOptions = statefulEngineOptions;
return this;
}
@CustomType.Setter
public Builder statefulRuleGroupReferences(@Nullable List statefulRuleGroupReferences) {
this.statefulRuleGroupReferences = statefulRuleGroupReferences;
return this;
}
public Builder statefulRuleGroupReferences(FirewallPolicyFirewallPolicyStatefulRuleGroupReference... statefulRuleGroupReferences) {
return statefulRuleGroupReferences(List.of(statefulRuleGroupReferences));
}
@CustomType.Setter
public Builder statelessCustomActions(@Nullable List statelessCustomActions) {
this.statelessCustomActions = statelessCustomActions;
return this;
}
public Builder statelessCustomActions(FirewallPolicyFirewallPolicyStatelessCustomAction... statelessCustomActions) {
return statelessCustomActions(List.of(statelessCustomActions));
}
@CustomType.Setter
public Builder statelessDefaultActions(List statelessDefaultActions) {
if (statelessDefaultActions == null) {
throw new MissingRequiredPropertyException("FirewallPolicyFirewallPolicy", "statelessDefaultActions");
}
this.statelessDefaultActions = statelessDefaultActions;
return this;
}
public Builder statelessDefaultActions(String... statelessDefaultActions) {
return statelessDefaultActions(List.of(statelessDefaultActions));
}
@CustomType.Setter
public Builder statelessFragmentDefaultActions(List statelessFragmentDefaultActions) {
if (statelessFragmentDefaultActions == null) {
throw new MissingRequiredPropertyException("FirewallPolicyFirewallPolicy", "statelessFragmentDefaultActions");
}
this.statelessFragmentDefaultActions = statelessFragmentDefaultActions;
return this;
}
public Builder statelessFragmentDefaultActions(String... statelessFragmentDefaultActions) {
return statelessFragmentDefaultActions(List.of(statelessFragmentDefaultActions));
}
@CustomType.Setter
public Builder statelessRuleGroupReferences(@Nullable List statelessRuleGroupReferences) {
this.statelessRuleGroupReferences = statelessRuleGroupReferences;
return this;
}
public Builder statelessRuleGroupReferences(FirewallPolicyFirewallPolicyStatelessRuleGroupReference... statelessRuleGroupReferences) {
return statelessRuleGroupReferences(List.of(statelessRuleGroupReferences));
}
@CustomType.Setter
public Builder tlsInspectionConfigurationArn(@Nullable String tlsInspectionConfigurationArn) {
this.tlsInspectionConfigurationArn = tlsInspectionConfigurationArn;
return this;
}
public FirewallPolicyFirewallPolicy build() {
final var _resultValue = new FirewallPolicyFirewallPolicy();
_resultValue.policyVariables = policyVariables;
_resultValue.statefulDefaultActions = statefulDefaultActions;
_resultValue.statefulEngineOptions = statefulEngineOptions;
_resultValue.statefulRuleGroupReferences = statefulRuleGroupReferences;
_resultValue.statelessCustomActions = statelessCustomActions;
_resultValue.statelessDefaultActions = statelessDefaultActions;
_resultValue.statelessFragmentDefaultActions = statelessFragmentDefaultActions;
_resultValue.statelessRuleGroupReferences = statelessRuleGroupReferences;
_resultValue.tlsInspectionConfigurationArn = tlsInspectionConfigurationArn;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy