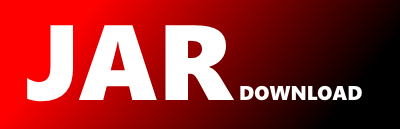
com.pulumi.aws.rds.GlobalCluster Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.rds;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.rds.GlobalClusterArgs;
import com.pulumi.aws.rds.inputs.GlobalClusterState;
import com.pulumi.aws.rds.outputs.GlobalClusterGlobalClusterMember;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Manages an RDS Global Cluster, which is an Aurora global database spread across multiple regions. The global database contains a single primary cluster with read-write capability, and a read-only secondary cluster that receives data from the primary cluster through high-speed replication performed by the Aurora storage subsystem.
*
* More information about Aurora global databases can be found in the [Aurora User Guide](https://docs.aws.amazon.com/AmazonRDS/latest/AuroraUserGuide/aurora-global-database.html#aurora-global-database-creating).
*
* ## Example Usage
*
* ### New MySQL Global Cluster
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.rds.GlobalCluster;
* import com.pulumi.aws.rds.GlobalClusterArgs;
* import com.pulumi.aws.rds.Cluster;
* import com.pulumi.aws.rds.ClusterArgs;
* import com.pulumi.aws.rds.ClusterInstance;
* import com.pulumi.aws.rds.ClusterInstanceArgs;
* import com.pulumi.resources.CustomResourceOptions;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new GlobalCluster("example", GlobalClusterArgs.builder()
* .globalClusterIdentifier("global-test")
* .engine("aurora")
* .engineVersion("5.6.mysql_aurora.1.22.2")
* .databaseName("example_db")
* .build());
*
* var primary = new Cluster("primary", ClusterArgs.builder()
* .engine(example.engine())
* .engineVersion(example.engineVersion())
* .clusterIdentifier("test-primary-cluster")
* .masterUsername("username")
* .masterPassword("somepass123")
* .databaseName("example_db")
* .globalClusterIdentifier(example.id())
* .dbSubnetGroupName("default")
* .build());
*
* var primaryClusterInstance = new ClusterInstance("primaryClusterInstance", ClusterInstanceArgs.builder()
* .engine(example.engine())
* .engineVersion(example.engineVersion())
* .identifier("test-primary-cluster-instance")
* .clusterIdentifier(primary.id())
* .instanceClass("db.r4.large")
* .dbSubnetGroupName("default")
* .build());
*
* var secondary = new Cluster("secondary", ClusterArgs.builder()
* .engine(example.engine())
* .engineVersion(example.engineVersion())
* .clusterIdentifier("test-secondary-cluster")
* .globalClusterIdentifier(example.id())
* .dbSubnetGroupName("default")
* .build(), CustomResourceOptions.builder()
* .dependsOn(primaryClusterInstance)
* .build());
*
* var secondaryClusterInstance = new ClusterInstance("secondaryClusterInstance", ClusterInstanceArgs.builder()
* .engine(example.engine())
* .engineVersion(example.engineVersion())
* .identifier("test-secondary-cluster-instance")
* .clusterIdentifier(secondary.id())
* .instanceClass("db.r4.large")
* .dbSubnetGroupName("default")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### New PostgreSQL Global Cluster
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.rds.GlobalCluster;
* import com.pulumi.aws.rds.GlobalClusterArgs;
* import com.pulumi.aws.rds.Cluster;
* import com.pulumi.aws.rds.ClusterArgs;
* import com.pulumi.aws.rds.ClusterInstance;
* import com.pulumi.aws.rds.ClusterInstanceArgs;
* import com.pulumi.resources.CustomResourceOptions;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new GlobalCluster("example", GlobalClusterArgs.builder()
* .globalClusterIdentifier("global-test")
* .engine("aurora-postgresql")
* .engineVersion("11.9")
* .databaseName("example_db")
* .build());
*
* var primary = new Cluster("primary", ClusterArgs.builder()
* .engine(example.engine())
* .engineVersion(example.engineVersion())
* .clusterIdentifier("test-primary-cluster")
* .masterUsername("username")
* .masterPassword("somepass123")
* .databaseName("example_db")
* .globalClusterIdentifier(example.id())
* .dbSubnetGroupName("default")
* .build());
*
* var primaryClusterInstance = new ClusterInstance("primaryClusterInstance", ClusterInstanceArgs.builder()
* .engine(example.engine())
* .engineVersion(example.engineVersion())
* .identifier("test-primary-cluster-instance")
* .clusterIdentifier(primary.id())
* .instanceClass("db.r4.large")
* .dbSubnetGroupName("default")
* .build());
*
* var secondary = new Cluster("secondary", ClusterArgs.builder()
* .engine(example.engine())
* .engineVersion(example.engineVersion())
* .clusterIdentifier("test-secondary-cluster")
* .globalClusterIdentifier(example.id())
* .skipFinalSnapshot(true)
* .dbSubnetGroupName("default")
* .build(), CustomResourceOptions.builder()
* .dependsOn(primaryClusterInstance)
* .build());
*
* var secondaryClusterInstance = new ClusterInstance("secondaryClusterInstance", ClusterInstanceArgs.builder()
* .engine(example.engine())
* .engineVersion(example.engineVersion())
* .identifier("test-secondary-cluster-instance")
* .clusterIdentifier(secondary.id())
* .instanceClass("db.r4.large")
* .dbSubnetGroupName("default")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### New Global Cluster From Existing DB Cluster
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.rds.Cluster;
* import com.pulumi.aws.rds.GlobalCluster;
* import com.pulumi.aws.rds.GlobalClusterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Cluster("example");
*
* var exampleGlobalCluster = new GlobalCluster("exampleGlobalCluster", GlobalClusterArgs.builder()
* .forceDestroy(true)
* .globalClusterIdentifier("example")
* .sourceDbClusterIdentifier(example.arn())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Upgrading Engine Versions
*
* When you upgrade the version of an `aws.rds.GlobalCluster`, the provider will attempt to in-place upgrade the engine versions of all associated clusters. Since the `aws.rds.Cluster` resource is being updated through the `aws.rds.GlobalCluster`, you are likely to get an error (`Provider produced inconsistent final plan`). To avoid this, use the `lifecycle` `ignore_changes` meta argument as shown below on the `aws.rds.Cluster`.
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.rds.GlobalCluster;
* import com.pulumi.aws.rds.GlobalClusterArgs;
* import com.pulumi.aws.rds.Cluster;
* import com.pulumi.aws.rds.ClusterArgs;
* import com.pulumi.aws.rds.ClusterInstance;
* import com.pulumi.aws.rds.ClusterInstanceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new GlobalCluster("example", GlobalClusterArgs.builder()
* .globalClusterIdentifier("kyivkharkiv")
* .engine("aurora-mysql")
* .engineVersion("5.7.mysql_aurora.2.07.5")
* .build());
*
* var primary = new Cluster("primary", ClusterArgs.builder()
* .allowMajorVersionUpgrade(true)
* .applyImmediately(true)
* .clusterIdentifier("odessadnipro")
* .databaseName("totoro")
* .engine(example.engine())
* .engineVersion(example.engineVersion())
* .globalClusterIdentifier(example.id())
* .masterPassword("satsukimae")
* .masterUsername("maesatsuki")
* .skipFinalSnapshot(true)
* .build());
*
* var primaryClusterInstance = new ClusterInstance("primaryClusterInstance", ClusterInstanceArgs.builder()
* .applyImmediately(true)
* .clusterIdentifier(primary.id())
* .engine(primary.engine())
* .engineVersion(primary.engineVersion())
* .identifier("donetsklviv")
* .instanceClass("db.r4.large")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import `aws_rds_global_cluster` using the RDS Global Cluster identifier. For example:
*
* ```sh
* $ pulumi import aws:rds/globalCluster:GlobalCluster example example
* ```
* Certain resource arguments, like `force_destroy`, only exist within this provider. If the argument is set in the the provider configuration on an imported resource, This provider will show a difference on the first plan after import to update the state value. This change is safe to apply immediately so the state matches the desired configuration.
*
* Certain resource arguments, like `source_db_cluster_identifier`, do not have an API method for reading the information after creation. If the argument is set in the Pulumi program on an imported resource, Pulumi will always show a difference. To workaround this behavior, either omit the argument from the Pulumi program or use `ignore_changes` to hide the difference. For example:
*
*/
@ResourceType(type="aws:rds/globalCluster:GlobalCluster")
public class GlobalCluster extends com.pulumi.resources.CustomResource {
/**
* RDS Global Cluster Amazon Resource Name (ARN)
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return RDS Global Cluster Amazon Resource Name (ARN)
*
*/
public Output arn() {
return this.arn;
}
/**
* Name for an automatically created database on cluster creation.
*
*/
@Export(name="databaseName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> databaseName;
/**
* @return Name for an automatically created database on cluster creation.
*
*/
public Output> databaseName() {
return Codegen.optional(this.databaseName);
}
/**
* If the Global Cluster should have deletion protection enabled. The database can't be deleted when this value is set to `true`. The default is `false`.
*
*/
@Export(name="deletionProtection", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> deletionProtection;
/**
* @return If the Global Cluster should have deletion protection enabled. The database can't be deleted when this value is set to `true`. The default is `false`.
*
*/
public Output> deletionProtection() {
return Codegen.optional(this.deletionProtection);
}
/**
* Name of the database engine to be used for this DB cluster. The provider will only perform drift detection if a configuration value is provided. Valid values: `aurora`, `aurora-mysql`, `aurora-postgresql`. Defaults to `aurora`. Conflicts with `source_db_cluster_identifier`.
*
*/
@Export(name="engine", refs={String.class}, tree="[0]")
private Output engine;
/**
* @return Name of the database engine to be used for this DB cluster. The provider will only perform drift detection if a configuration value is provided. Valid values: `aurora`, `aurora-mysql`, `aurora-postgresql`. Defaults to `aurora`. Conflicts with `source_db_cluster_identifier`.
*
*/
public Output engine() {
return this.engine;
}
/**
* Engine version of the Aurora global database. The `engine`, `engine_version`, and `instance_class` (on the `aws.rds.ClusterInstance`) must together support global databases. See [Using Amazon Aurora global databases](https://docs.aws.amazon.com/AmazonRDS/latest/AuroraUserGuide/aurora-global-database.html) for more information. By upgrading the engine version, the provider will upgrade cluster members. **NOTE:** To avoid an `inconsistent final plan` error while upgrading, use the `lifecycle` `ignore_changes` for `engine_version` meta argument on the associated `aws.rds.Cluster` resource as shown above in Upgrading Engine Versions example.
*
*/
@Export(name="engineVersion", refs={String.class}, tree="[0]")
private Output engineVersion;
/**
* @return Engine version of the Aurora global database. The `engine`, `engine_version`, and `instance_class` (on the `aws.rds.ClusterInstance`) must together support global databases. See [Using Amazon Aurora global databases](https://docs.aws.amazon.com/AmazonRDS/latest/AuroraUserGuide/aurora-global-database.html) for more information. By upgrading the engine version, the provider will upgrade cluster members. **NOTE:** To avoid an `inconsistent final plan` error while upgrading, use the `lifecycle` `ignore_changes` for `engine_version` meta argument on the associated `aws.rds.Cluster` resource as shown above in Upgrading Engine Versions example.
*
*/
public Output engineVersion() {
return this.engineVersion;
}
@Export(name="engineVersionActual", refs={String.class}, tree="[0]")
private Output engineVersionActual;
public Output engineVersionActual() {
return this.engineVersionActual;
}
/**
* Enable to remove DB Cluster members from Global Cluster on destroy. Required with `source_db_cluster_identifier`.
*
*/
@Export(name="forceDestroy", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> forceDestroy;
/**
* @return Enable to remove DB Cluster members from Global Cluster on destroy. Required with `source_db_cluster_identifier`.
*
*/
public Output> forceDestroy() {
return Codegen.optional(this.forceDestroy);
}
/**
* Global cluster identifier.
*
*/
@Export(name="globalClusterIdentifier", refs={String.class}, tree="[0]")
private Output globalClusterIdentifier;
/**
* @return Global cluster identifier.
*
*/
public Output globalClusterIdentifier() {
return this.globalClusterIdentifier;
}
/**
* Set of objects containing Global Cluster members.
*
*/
@Export(name="globalClusterMembers", refs={List.class,GlobalClusterGlobalClusterMember.class}, tree="[0,1]")
private Output> globalClusterMembers;
/**
* @return Set of objects containing Global Cluster members.
*
*/
public Output> globalClusterMembers() {
return this.globalClusterMembers;
}
/**
* AWS Region-unique, immutable identifier for the global database cluster. This identifier is found in AWS CloudTrail log entries whenever the AWS KMS key for the DB cluster is accessed
*
*/
@Export(name="globalClusterResourceId", refs={String.class}, tree="[0]")
private Output globalClusterResourceId;
/**
* @return AWS Region-unique, immutable identifier for the global database cluster. This identifier is found in AWS CloudTrail log entries whenever the AWS KMS key for the DB cluster is accessed
*
*/
public Output globalClusterResourceId() {
return this.globalClusterResourceId;
}
/**
* Amazon Resource Name (ARN) to use as the primary DB Cluster of the Global Cluster on creation. The provider cannot perform drift detection of this value.
*
*/
@Export(name="sourceDbClusterIdentifier", refs={String.class}, tree="[0]")
private Output sourceDbClusterIdentifier;
/**
* @return Amazon Resource Name (ARN) to use as the primary DB Cluster of the Global Cluster on creation. The provider cannot perform drift detection of this value.
*
*/
public Output sourceDbClusterIdentifier() {
return this.sourceDbClusterIdentifier;
}
/**
* Specifies whether the DB cluster is encrypted. The default is `false` unless `source_db_cluster_identifier` is specified and encrypted. The provider will only perform drift detection if a configuration value is provided.
*
*/
@Export(name="storageEncrypted", refs={Boolean.class}, tree="[0]")
private Output storageEncrypted;
/**
* @return Specifies whether the DB cluster is encrypted. The default is `false` unless `source_db_cluster_identifier` is specified and encrypted. The provider will only perform drift detection if a configuration value is provided.
*
*/
public Output storageEncrypted() {
return this.storageEncrypted;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public GlobalCluster(String name) {
this(name, GlobalClusterArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public GlobalCluster(String name, GlobalClusterArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public GlobalCluster(String name, GlobalClusterArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:rds/globalCluster:GlobalCluster", name, args == null ? GlobalClusterArgs.Empty : args, makeResourceOptions(options, Codegen.empty()));
}
private GlobalCluster(String name, Output id, @Nullable GlobalClusterState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:rds/globalCluster:GlobalCluster", name, state, makeResourceOptions(options, id));
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static GlobalCluster get(String name, Output id, @Nullable GlobalClusterState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new GlobalCluster(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy