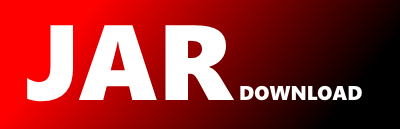
com.pulumi.aws.rds.outputs.GetInstanceResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.rds.outputs;
import com.pulumi.aws.rds.outputs.GetInstanceMasterUserSecret;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class GetInstanceResult {
/**
* @return Hostname of the RDS instance. See also `endpoint` and `port`.
*
*/
private String address;
/**
* @return Allocated storage size specified in gigabytes.
*
*/
private Integer allocatedStorage;
/**
* @return Indicates that minor version patches are applied automatically.
*
*/
private Boolean autoMinorVersionUpgrade;
/**
* @return Name of the Availability Zone the DB instance is located in.
*
*/
private String availabilityZone;
/**
* @return Specifies the number of days for which automatic DB snapshots are retained.
*
*/
private Integer backupRetentionPeriod;
/**
* @return Identifier of the CA certificate for the DB instance.
*
*/
private String caCertIdentifier;
/**
* @return If the DB instance is a member of a DB cluster, contains the name of the DB cluster that the DB instance is a member of.
*
*/
private String dbClusterIdentifier;
/**
* @return ARN for the DB instance.
*
*/
private String dbInstanceArn;
/**
* @return Contains the name of the compute and memory capacity class of the DB instance.
*
*/
private String dbInstanceClass;
private String dbInstanceIdentifier;
/**
* @return Port that the DB instance listens on.
*
*/
private Integer dbInstancePort;
/**
* @return Contains the name of the initial database of this instance that was provided at create time, if one was specified when the DB instance was created. This same name is returned for the life of the DB instance.
*
*/
private String dbName;
/**
* @return Provides the list of DB parameter groups applied to this DB instance.
*
*/
private List dbParameterGroups;
/**
* @return Name of the subnet group associated with the DB instance.
*
*/
private String dbSubnetGroup;
/**
* @return List of log types to export to cloudwatch.
*
*/
private List enabledCloudwatchLogsExports;
/**
* @return Connection endpoint in `address:port` format.
*
*/
private String endpoint;
/**
* @return Provides the name of the database engine to be used for this DB instance.
*
*/
private String engine;
/**
* @return Database engine version.
*
*/
private String engineVersion;
/**
* @return Canonical hosted zone ID of the DB instance (to be used in a Route 53 Alias record).
*
*/
private String hostedZoneId;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
/**
* @return Provisioned IOPS (I/O operations per second) value.
*
*/
private Integer iops;
/**
* @return The Amazon Web Services KMS key identifier that is used to encrypt the secret.
*
*/
private String kmsKeyId;
/**
* @return License model information for this DB instance.
*
*/
private String licenseModel;
/**
* @return Provides the master user secret. Only available when `manage_master_user_password` is set to true. Documented below.
*
*/
private List masterUserSecrets;
/**
* @return Contains the master username for the DB instance.
*
*/
private String masterUsername;
/**
* @return The upper limit to which Amazon RDS can automatically scale the storage of the DB instance.
*
*/
private Integer maxAllocatedStorage;
/**
* @return Interval, in seconds, between points when Enhanced Monitoring metrics are collected for the DB instance.
*
*/
private Integer monitoringInterval;
/**
* @return ARN for the IAM role that permits RDS to send Enhanced Monitoring metrics to CloudWatch Logs.
*
*/
private String monitoringRoleArn;
/**
* @return If the DB instance is a Multi-AZ deployment.
*
*/
private Boolean multiAz;
/**
* @return Network type of the DB instance.
*
*/
private String networkType;
/**
* @return Provides the list of option group memberships for this DB instance.
*
*/
private List optionGroupMemberships;
/**
* @return Database endpoint port, primarily used by an Aurora DB cluster. For a conventional RDS DB instance, the `db_instance_port` is typically the preferred choice.
*
*/
private Integer port;
/**
* @return Specifies the daily time range during which automated backups are created.
*
*/
private String preferredBackupWindow;
/**
* @return Specifies the weekly time range during which system maintenance can occur in UTC.
*
*/
private String preferredMaintenanceWindow;
/**
* @return Accessibility options for the DB instance.
*
*/
private Boolean publiclyAccessible;
/**
* @return Identifier of the source DB that this is a replica of.
*
*/
private String replicateSourceDb;
/**
* @return RDS Resource ID of this instance.
*
*/
private String resourceId;
/**
* @return Whether the DB instance is encrypted.
*
*/
private Boolean storageEncrypted;
/**
* @return Storage throughput value for the DB instance.
*
*/
private Integer storageThroughput;
/**
* @return Storage type associated with DB instance.
*
*/
private String storageType;
private Map tags;
/**
* @return Time zone of the DB instance.
*
*/
private String timezone;
/**
* @return Provides a list of VPC security group elements that the DB instance belongs to.
*
*/
private List vpcSecurityGroups;
private GetInstanceResult() {}
/**
* @return Hostname of the RDS instance. See also `endpoint` and `port`.
*
*/
public String address() {
return this.address;
}
/**
* @return Allocated storage size specified in gigabytes.
*
*/
public Integer allocatedStorage() {
return this.allocatedStorage;
}
/**
* @return Indicates that minor version patches are applied automatically.
*
*/
public Boolean autoMinorVersionUpgrade() {
return this.autoMinorVersionUpgrade;
}
/**
* @return Name of the Availability Zone the DB instance is located in.
*
*/
public String availabilityZone() {
return this.availabilityZone;
}
/**
* @return Specifies the number of days for which automatic DB snapshots are retained.
*
*/
public Integer backupRetentionPeriod() {
return this.backupRetentionPeriod;
}
/**
* @return Identifier of the CA certificate for the DB instance.
*
*/
public String caCertIdentifier() {
return this.caCertIdentifier;
}
/**
* @return If the DB instance is a member of a DB cluster, contains the name of the DB cluster that the DB instance is a member of.
*
*/
public String dbClusterIdentifier() {
return this.dbClusterIdentifier;
}
/**
* @return ARN for the DB instance.
*
*/
public String dbInstanceArn() {
return this.dbInstanceArn;
}
/**
* @return Contains the name of the compute and memory capacity class of the DB instance.
*
*/
public String dbInstanceClass() {
return this.dbInstanceClass;
}
public String dbInstanceIdentifier() {
return this.dbInstanceIdentifier;
}
/**
* @return Port that the DB instance listens on.
*
*/
public Integer dbInstancePort() {
return this.dbInstancePort;
}
/**
* @return Contains the name of the initial database of this instance that was provided at create time, if one was specified when the DB instance was created. This same name is returned for the life of the DB instance.
*
*/
public String dbName() {
return this.dbName;
}
/**
* @return Provides the list of DB parameter groups applied to this DB instance.
*
*/
public List dbParameterGroups() {
return this.dbParameterGroups;
}
/**
* @return Name of the subnet group associated with the DB instance.
*
*/
public String dbSubnetGroup() {
return this.dbSubnetGroup;
}
/**
* @return List of log types to export to cloudwatch.
*
*/
public List enabledCloudwatchLogsExports() {
return this.enabledCloudwatchLogsExports;
}
/**
* @return Connection endpoint in `address:port` format.
*
*/
public String endpoint() {
return this.endpoint;
}
/**
* @return Provides the name of the database engine to be used for this DB instance.
*
*/
public String engine() {
return this.engine;
}
/**
* @return Database engine version.
*
*/
public String engineVersion() {
return this.engineVersion;
}
/**
* @return Canonical hosted zone ID of the DB instance (to be used in a Route 53 Alias record).
*
*/
public String hostedZoneId() {
return this.hostedZoneId;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
/**
* @return Provisioned IOPS (I/O operations per second) value.
*
*/
public Integer iops() {
return this.iops;
}
/**
* @return The Amazon Web Services KMS key identifier that is used to encrypt the secret.
*
*/
public String kmsKeyId() {
return this.kmsKeyId;
}
/**
* @return License model information for this DB instance.
*
*/
public String licenseModel() {
return this.licenseModel;
}
/**
* @return Provides the master user secret. Only available when `manage_master_user_password` is set to true. Documented below.
*
*/
public List masterUserSecrets() {
return this.masterUserSecrets;
}
/**
* @return Contains the master username for the DB instance.
*
*/
public String masterUsername() {
return this.masterUsername;
}
/**
* @return The upper limit to which Amazon RDS can automatically scale the storage of the DB instance.
*
*/
public Integer maxAllocatedStorage() {
return this.maxAllocatedStorage;
}
/**
* @return Interval, in seconds, between points when Enhanced Monitoring metrics are collected for the DB instance.
*
*/
public Integer monitoringInterval() {
return this.monitoringInterval;
}
/**
* @return ARN for the IAM role that permits RDS to send Enhanced Monitoring metrics to CloudWatch Logs.
*
*/
public String monitoringRoleArn() {
return this.monitoringRoleArn;
}
/**
* @return If the DB instance is a Multi-AZ deployment.
*
*/
public Boolean multiAz() {
return this.multiAz;
}
/**
* @return Network type of the DB instance.
*
*/
public String networkType() {
return this.networkType;
}
/**
* @return Provides the list of option group memberships for this DB instance.
*
*/
public List optionGroupMemberships() {
return this.optionGroupMemberships;
}
/**
* @return Database endpoint port, primarily used by an Aurora DB cluster. For a conventional RDS DB instance, the `db_instance_port` is typically the preferred choice.
*
*/
public Integer port() {
return this.port;
}
/**
* @return Specifies the daily time range during which automated backups are created.
*
*/
public String preferredBackupWindow() {
return this.preferredBackupWindow;
}
/**
* @return Specifies the weekly time range during which system maintenance can occur in UTC.
*
*/
public String preferredMaintenanceWindow() {
return this.preferredMaintenanceWindow;
}
/**
* @return Accessibility options for the DB instance.
*
*/
public Boolean publiclyAccessible() {
return this.publiclyAccessible;
}
/**
* @return Identifier of the source DB that this is a replica of.
*
*/
public String replicateSourceDb() {
return this.replicateSourceDb;
}
/**
* @return RDS Resource ID of this instance.
*
*/
public String resourceId() {
return this.resourceId;
}
/**
* @return Whether the DB instance is encrypted.
*
*/
public Boolean storageEncrypted() {
return this.storageEncrypted;
}
/**
* @return Storage throughput value for the DB instance.
*
*/
public Integer storageThroughput() {
return this.storageThroughput;
}
/**
* @return Storage type associated with DB instance.
*
*/
public String storageType() {
return this.storageType;
}
public Map tags() {
return this.tags;
}
/**
* @return Time zone of the DB instance.
*
*/
public String timezone() {
return this.timezone;
}
/**
* @return Provides a list of VPC security group elements that the DB instance belongs to.
*
*/
public List vpcSecurityGroups() {
return this.vpcSecurityGroups;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetInstanceResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String address;
private Integer allocatedStorage;
private Boolean autoMinorVersionUpgrade;
private String availabilityZone;
private Integer backupRetentionPeriod;
private String caCertIdentifier;
private String dbClusterIdentifier;
private String dbInstanceArn;
private String dbInstanceClass;
private String dbInstanceIdentifier;
private Integer dbInstancePort;
private String dbName;
private List dbParameterGroups;
private String dbSubnetGroup;
private List enabledCloudwatchLogsExports;
private String endpoint;
private String engine;
private String engineVersion;
private String hostedZoneId;
private String id;
private Integer iops;
private String kmsKeyId;
private String licenseModel;
private List masterUserSecrets;
private String masterUsername;
private Integer maxAllocatedStorage;
private Integer monitoringInterval;
private String monitoringRoleArn;
private Boolean multiAz;
private String networkType;
private List optionGroupMemberships;
private Integer port;
private String preferredBackupWindow;
private String preferredMaintenanceWindow;
private Boolean publiclyAccessible;
private String replicateSourceDb;
private String resourceId;
private Boolean storageEncrypted;
private Integer storageThroughput;
private String storageType;
private Map tags;
private String timezone;
private List vpcSecurityGroups;
public Builder() {}
public Builder(GetInstanceResult defaults) {
Objects.requireNonNull(defaults);
this.address = defaults.address;
this.allocatedStorage = defaults.allocatedStorage;
this.autoMinorVersionUpgrade = defaults.autoMinorVersionUpgrade;
this.availabilityZone = defaults.availabilityZone;
this.backupRetentionPeriod = defaults.backupRetentionPeriod;
this.caCertIdentifier = defaults.caCertIdentifier;
this.dbClusterIdentifier = defaults.dbClusterIdentifier;
this.dbInstanceArn = defaults.dbInstanceArn;
this.dbInstanceClass = defaults.dbInstanceClass;
this.dbInstanceIdentifier = defaults.dbInstanceIdentifier;
this.dbInstancePort = defaults.dbInstancePort;
this.dbName = defaults.dbName;
this.dbParameterGroups = defaults.dbParameterGroups;
this.dbSubnetGroup = defaults.dbSubnetGroup;
this.enabledCloudwatchLogsExports = defaults.enabledCloudwatchLogsExports;
this.endpoint = defaults.endpoint;
this.engine = defaults.engine;
this.engineVersion = defaults.engineVersion;
this.hostedZoneId = defaults.hostedZoneId;
this.id = defaults.id;
this.iops = defaults.iops;
this.kmsKeyId = defaults.kmsKeyId;
this.licenseModel = defaults.licenseModel;
this.masterUserSecrets = defaults.masterUserSecrets;
this.masterUsername = defaults.masterUsername;
this.maxAllocatedStorage = defaults.maxAllocatedStorage;
this.monitoringInterval = defaults.monitoringInterval;
this.monitoringRoleArn = defaults.monitoringRoleArn;
this.multiAz = defaults.multiAz;
this.networkType = defaults.networkType;
this.optionGroupMemberships = defaults.optionGroupMemberships;
this.port = defaults.port;
this.preferredBackupWindow = defaults.preferredBackupWindow;
this.preferredMaintenanceWindow = defaults.preferredMaintenanceWindow;
this.publiclyAccessible = defaults.publiclyAccessible;
this.replicateSourceDb = defaults.replicateSourceDb;
this.resourceId = defaults.resourceId;
this.storageEncrypted = defaults.storageEncrypted;
this.storageThroughput = defaults.storageThroughput;
this.storageType = defaults.storageType;
this.tags = defaults.tags;
this.timezone = defaults.timezone;
this.vpcSecurityGroups = defaults.vpcSecurityGroups;
}
@CustomType.Setter
public Builder address(String address) {
if (address == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "address");
}
this.address = address;
return this;
}
@CustomType.Setter
public Builder allocatedStorage(Integer allocatedStorage) {
if (allocatedStorage == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "allocatedStorage");
}
this.allocatedStorage = allocatedStorage;
return this;
}
@CustomType.Setter
public Builder autoMinorVersionUpgrade(Boolean autoMinorVersionUpgrade) {
if (autoMinorVersionUpgrade == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "autoMinorVersionUpgrade");
}
this.autoMinorVersionUpgrade = autoMinorVersionUpgrade;
return this;
}
@CustomType.Setter
public Builder availabilityZone(String availabilityZone) {
if (availabilityZone == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "availabilityZone");
}
this.availabilityZone = availabilityZone;
return this;
}
@CustomType.Setter
public Builder backupRetentionPeriod(Integer backupRetentionPeriod) {
if (backupRetentionPeriod == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "backupRetentionPeriod");
}
this.backupRetentionPeriod = backupRetentionPeriod;
return this;
}
@CustomType.Setter
public Builder caCertIdentifier(String caCertIdentifier) {
if (caCertIdentifier == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "caCertIdentifier");
}
this.caCertIdentifier = caCertIdentifier;
return this;
}
@CustomType.Setter
public Builder dbClusterIdentifier(String dbClusterIdentifier) {
if (dbClusterIdentifier == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "dbClusterIdentifier");
}
this.dbClusterIdentifier = dbClusterIdentifier;
return this;
}
@CustomType.Setter
public Builder dbInstanceArn(String dbInstanceArn) {
if (dbInstanceArn == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "dbInstanceArn");
}
this.dbInstanceArn = dbInstanceArn;
return this;
}
@CustomType.Setter
public Builder dbInstanceClass(String dbInstanceClass) {
if (dbInstanceClass == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "dbInstanceClass");
}
this.dbInstanceClass = dbInstanceClass;
return this;
}
@CustomType.Setter
public Builder dbInstanceIdentifier(String dbInstanceIdentifier) {
if (dbInstanceIdentifier == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "dbInstanceIdentifier");
}
this.dbInstanceIdentifier = dbInstanceIdentifier;
return this;
}
@CustomType.Setter
public Builder dbInstancePort(Integer dbInstancePort) {
if (dbInstancePort == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "dbInstancePort");
}
this.dbInstancePort = dbInstancePort;
return this;
}
@CustomType.Setter
public Builder dbName(String dbName) {
if (dbName == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "dbName");
}
this.dbName = dbName;
return this;
}
@CustomType.Setter
public Builder dbParameterGroups(List dbParameterGroups) {
if (dbParameterGroups == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "dbParameterGroups");
}
this.dbParameterGroups = dbParameterGroups;
return this;
}
public Builder dbParameterGroups(String... dbParameterGroups) {
return dbParameterGroups(List.of(dbParameterGroups));
}
@CustomType.Setter
public Builder dbSubnetGroup(String dbSubnetGroup) {
if (dbSubnetGroup == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "dbSubnetGroup");
}
this.dbSubnetGroup = dbSubnetGroup;
return this;
}
@CustomType.Setter
public Builder enabledCloudwatchLogsExports(List enabledCloudwatchLogsExports) {
if (enabledCloudwatchLogsExports == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "enabledCloudwatchLogsExports");
}
this.enabledCloudwatchLogsExports = enabledCloudwatchLogsExports;
return this;
}
public Builder enabledCloudwatchLogsExports(String... enabledCloudwatchLogsExports) {
return enabledCloudwatchLogsExports(List.of(enabledCloudwatchLogsExports));
}
@CustomType.Setter
public Builder endpoint(String endpoint) {
if (endpoint == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "endpoint");
}
this.endpoint = endpoint;
return this;
}
@CustomType.Setter
public Builder engine(String engine) {
if (engine == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "engine");
}
this.engine = engine;
return this;
}
@CustomType.Setter
public Builder engineVersion(String engineVersion) {
if (engineVersion == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "engineVersion");
}
this.engineVersion = engineVersion;
return this;
}
@CustomType.Setter
public Builder hostedZoneId(String hostedZoneId) {
if (hostedZoneId == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "hostedZoneId");
}
this.hostedZoneId = hostedZoneId;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder iops(Integer iops) {
if (iops == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "iops");
}
this.iops = iops;
return this;
}
@CustomType.Setter
public Builder kmsKeyId(String kmsKeyId) {
if (kmsKeyId == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "kmsKeyId");
}
this.kmsKeyId = kmsKeyId;
return this;
}
@CustomType.Setter
public Builder licenseModel(String licenseModel) {
if (licenseModel == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "licenseModel");
}
this.licenseModel = licenseModel;
return this;
}
@CustomType.Setter
public Builder masterUserSecrets(List masterUserSecrets) {
if (masterUserSecrets == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "masterUserSecrets");
}
this.masterUserSecrets = masterUserSecrets;
return this;
}
public Builder masterUserSecrets(GetInstanceMasterUserSecret... masterUserSecrets) {
return masterUserSecrets(List.of(masterUserSecrets));
}
@CustomType.Setter
public Builder masterUsername(String masterUsername) {
if (masterUsername == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "masterUsername");
}
this.masterUsername = masterUsername;
return this;
}
@CustomType.Setter
public Builder maxAllocatedStorage(Integer maxAllocatedStorage) {
if (maxAllocatedStorage == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "maxAllocatedStorage");
}
this.maxAllocatedStorage = maxAllocatedStorage;
return this;
}
@CustomType.Setter
public Builder monitoringInterval(Integer monitoringInterval) {
if (monitoringInterval == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "monitoringInterval");
}
this.monitoringInterval = monitoringInterval;
return this;
}
@CustomType.Setter
public Builder monitoringRoleArn(String monitoringRoleArn) {
if (monitoringRoleArn == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "monitoringRoleArn");
}
this.monitoringRoleArn = monitoringRoleArn;
return this;
}
@CustomType.Setter
public Builder multiAz(Boolean multiAz) {
if (multiAz == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "multiAz");
}
this.multiAz = multiAz;
return this;
}
@CustomType.Setter
public Builder networkType(String networkType) {
if (networkType == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "networkType");
}
this.networkType = networkType;
return this;
}
@CustomType.Setter
public Builder optionGroupMemberships(List optionGroupMemberships) {
if (optionGroupMemberships == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "optionGroupMemberships");
}
this.optionGroupMemberships = optionGroupMemberships;
return this;
}
public Builder optionGroupMemberships(String... optionGroupMemberships) {
return optionGroupMemberships(List.of(optionGroupMemberships));
}
@CustomType.Setter
public Builder port(Integer port) {
if (port == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "port");
}
this.port = port;
return this;
}
@CustomType.Setter
public Builder preferredBackupWindow(String preferredBackupWindow) {
if (preferredBackupWindow == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "preferredBackupWindow");
}
this.preferredBackupWindow = preferredBackupWindow;
return this;
}
@CustomType.Setter
public Builder preferredMaintenanceWindow(String preferredMaintenanceWindow) {
if (preferredMaintenanceWindow == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "preferredMaintenanceWindow");
}
this.preferredMaintenanceWindow = preferredMaintenanceWindow;
return this;
}
@CustomType.Setter
public Builder publiclyAccessible(Boolean publiclyAccessible) {
if (publiclyAccessible == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "publiclyAccessible");
}
this.publiclyAccessible = publiclyAccessible;
return this;
}
@CustomType.Setter
public Builder replicateSourceDb(String replicateSourceDb) {
if (replicateSourceDb == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "replicateSourceDb");
}
this.replicateSourceDb = replicateSourceDb;
return this;
}
@CustomType.Setter
public Builder resourceId(String resourceId) {
if (resourceId == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "resourceId");
}
this.resourceId = resourceId;
return this;
}
@CustomType.Setter
public Builder storageEncrypted(Boolean storageEncrypted) {
if (storageEncrypted == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "storageEncrypted");
}
this.storageEncrypted = storageEncrypted;
return this;
}
@CustomType.Setter
public Builder storageThroughput(Integer storageThroughput) {
if (storageThroughput == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "storageThroughput");
}
this.storageThroughput = storageThroughput;
return this;
}
@CustomType.Setter
public Builder storageType(String storageType) {
if (storageType == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "storageType");
}
this.storageType = storageType;
return this;
}
@CustomType.Setter
public Builder tags(Map tags) {
if (tags == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "tags");
}
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder timezone(String timezone) {
if (timezone == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "timezone");
}
this.timezone = timezone;
return this;
}
@CustomType.Setter
public Builder vpcSecurityGroups(List vpcSecurityGroups) {
if (vpcSecurityGroups == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "vpcSecurityGroups");
}
this.vpcSecurityGroups = vpcSecurityGroups;
return this;
}
public Builder vpcSecurityGroups(String... vpcSecurityGroups) {
return vpcSecurityGroups(List.of(vpcSecurityGroups));
}
public GetInstanceResult build() {
final var _resultValue = new GetInstanceResult();
_resultValue.address = address;
_resultValue.allocatedStorage = allocatedStorage;
_resultValue.autoMinorVersionUpgrade = autoMinorVersionUpgrade;
_resultValue.availabilityZone = availabilityZone;
_resultValue.backupRetentionPeriod = backupRetentionPeriod;
_resultValue.caCertIdentifier = caCertIdentifier;
_resultValue.dbClusterIdentifier = dbClusterIdentifier;
_resultValue.dbInstanceArn = dbInstanceArn;
_resultValue.dbInstanceClass = dbInstanceClass;
_resultValue.dbInstanceIdentifier = dbInstanceIdentifier;
_resultValue.dbInstancePort = dbInstancePort;
_resultValue.dbName = dbName;
_resultValue.dbParameterGroups = dbParameterGroups;
_resultValue.dbSubnetGroup = dbSubnetGroup;
_resultValue.enabledCloudwatchLogsExports = enabledCloudwatchLogsExports;
_resultValue.endpoint = endpoint;
_resultValue.engine = engine;
_resultValue.engineVersion = engineVersion;
_resultValue.hostedZoneId = hostedZoneId;
_resultValue.id = id;
_resultValue.iops = iops;
_resultValue.kmsKeyId = kmsKeyId;
_resultValue.licenseModel = licenseModel;
_resultValue.masterUserSecrets = masterUserSecrets;
_resultValue.masterUsername = masterUsername;
_resultValue.maxAllocatedStorage = maxAllocatedStorage;
_resultValue.monitoringInterval = monitoringInterval;
_resultValue.monitoringRoleArn = monitoringRoleArn;
_resultValue.multiAz = multiAz;
_resultValue.networkType = networkType;
_resultValue.optionGroupMemberships = optionGroupMemberships;
_resultValue.port = port;
_resultValue.preferredBackupWindow = preferredBackupWindow;
_resultValue.preferredMaintenanceWindow = preferredMaintenanceWindow;
_resultValue.publiclyAccessible = publiclyAccessible;
_resultValue.replicateSourceDb = replicateSourceDb;
_resultValue.resourceId = resourceId;
_resultValue.storageEncrypted = storageEncrypted;
_resultValue.storageThroughput = storageThroughput;
_resultValue.storageType = storageType;
_resultValue.tags = tags;
_resultValue.timezone = timezone;
_resultValue.vpcSecurityGroups = vpcSecurityGroups;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy