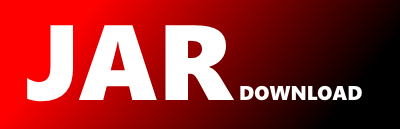
com.pulumi.aws.route53.inputs.GetResolverRulesPlainArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.route53.inputs;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class GetResolverRulesPlainArgs extends com.pulumi.resources.InvokeArgs {
public static final GetResolverRulesPlainArgs Empty = new GetResolverRulesPlainArgs();
/**
* Regex string to filter resolver rule names.
* The filtering is done locally, so could have a performance impact if the result is large.
* This argument should be used along with other arguments to limit the number of results returned.
*
*/
@Import(name="nameRegex")
private @Nullable String nameRegex;
/**
* @return Regex string to filter resolver rule names.
* The filtering is done locally, so could have a performance impact if the result is large.
* This argument should be used along with other arguments to limit the number of results returned.
*
*/
public Optional nameRegex() {
return Optional.ofNullable(this.nameRegex);
}
/**
* When the desired resolver rules are shared with another AWS account, the account ID of the account that the rules are shared with.
*
*/
@Import(name="ownerId")
private @Nullable String ownerId;
/**
* @return When the desired resolver rules are shared with another AWS account, the account ID of the account that the rules are shared with.
*
*/
public Optional ownerId() {
return Optional.ofNullable(this.ownerId);
}
/**
* ID of the outbound resolver endpoint for the desired resolver rules.
*
*/
@Import(name="resolverEndpointId")
private @Nullable String resolverEndpointId;
/**
* @return ID of the outbound resolver endpoint for the desired resolver rules.
*
*/
public Optional resolverEndpointId() {
return Optional.ofNullable(this.resolverEndpointId);
}
/**
* Rule type of the desired resolver rules. Valid values are `FORWARD`, `SYSTEM` and `RECURSIVE`.
*
*/
@Import(name="ruleType")
private @Nullable String ruleType;
/**
* @return Rule type of the desired resolver rules. Valid values are `FORWARD`, `SYSTEM` and `RECURSIVE`.
*
*/
public Optional ruleType() {
return Optional.ofNullable(this.ruleType);
}
/**
* Whether the desired resolver rules are shared and, if so, whether the current account is sharing the rules with another account, or another account is sharing the rules with the current account. Valid values are `NOT_SHARED`, `SHARED_BY_ME` or `SHARED_WITH_ME`
*
*/
@Import(name="shareStatus")
private @Nullable String shareStatus;
/**
* @return Whether the desired resolver rules are shared and, if so, whether the current account is sharing the rules with another account, or another account is sharing the rules with the current account. Valid values are `NOT_SHARED`, `SHARED_BY_ME` or `SHARED_WITH_ME`
*
*/
public Optional shareStatus() {
return Optional.ofNullable(this.shareStatus);
}
private GetResolverRulesPlainArgs() {}
private GetResolverRulesPlainArgs(GetResolverRulesPlainArgs $) {
this.nameRegex = $.nameRegex;
this.ownerId = $.ownerId;
this.resolverEndpointId = $.resolverEndpointId;
this.ruleType = $.ruleType;
this.shareStatus = $.shareStatus;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetResolverRulesPlainArgs defaults) {
return new Builder(defaults);
}
public static final class Builder {
private GetResolverRulesPlainArgs $;
public Builder() {
$ = new GetResolverRulesPlainArgs();
}
public Builder(GetResolverRulesPlainArgs defaults) {
$ = new GetResolverRulesPlainArgs(Objects.requireNonNull(defaults));
}
/**
* @param nameRegex Regex string to filter resolver rule names.
* The filtering is done locally, so could have a performance impact if the result is large.
* This argument should be used along with other arguments to limit the number of results returned.
*
* @return builder
*
*/
public Builder nameRegex(@Nullable String nameRegex) {
$.nameRegex = nameRegex;
return this;
}
/**
* @param ownerId When the desired resolver rules are shared with another AWS account, the account ID of the account that the rules are shared with.
*
* @return builder
*
*/
public Builder ownerId(@Nullable String ownerId) {
$.ownerId = ownerId;
return this;
}
/**
* @param resolverEndpointId ID of the outbound resolver endpoint for the desired resolver rules.
*
* @return builder
*
*/
public Builder resolverEndpointId(@Nullable String resolverEndpointId) {
$.resolverEndpointId = resolverEndpointId;
return this;
}
/**
* @param ruleType Rule type of the desired resolver rules. Valid values are `FORWARD`, `SYSTEM` and `RECURSIVE`.
*
* @return builder
*
*/
public Builder ruleType(@Nullable String ruleType) {
$.ruleType = ruleType;
return this;
}
/**
* @param shareStatus Whether the desired resolver rules are shared and, if so, whether the current account is sharing the rules with another account, or another account is sharing the rules with the current account. Valid values are `NOT_SHARED`, `SHARED_BY_ME` or `SHARED_WITH_ME`
*
* @return builder
*
*/
public Builder shareStatus(@Nullable String shareStatus) {
$.shareStatus = shareStatus;
return this;
}
public GetResolverRulesPlainArgs build() {
return $;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy