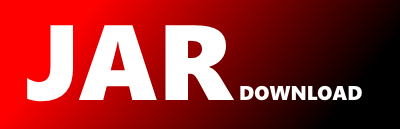
com.pulumi.aws.s3.BucketVersioningV2 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.s3;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.s3.BucketVersioningV2Args;
import com.pulumi.aws.s3.inputs.BucketVersioningV2State;
import com.pulumi.aws.s3.outputs.BucketVersioningV2VersioningConfiguration;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides a resource for controlling versioning on an S3 bucket.
* Deleting this resource will either suspend versioning on the associated S3 bucket or
* simply remove the resource from state if the associated S3 bucket is unversioned.
*
* For more information, see [How S3 versioning works](https://docs.aws.amazon.com/AmazonS3/latest/userguide/manage-versioning-examples.html).
*
* > **NOTE:** If you are enabling versioning on the bucket for the first time, AWS recommends that you wait for 15 minutes after enabling versioning before issuing write operations (PUT or DELETE) on objects in the bucket.
*
* > This resource cannot be used with S3 directory buckets.
*
* ## Example Usage
*
* ### With Versioning Enabled
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.s3.BucketV2;
* import com.pulumi.aws.s3.BucketV2Args;
* import com.pulumi.aws.s3.BucketAclV2;
* import com.pulumi.aws.s3.BucketAclV2Args;
* import com.pulumi.aws.s3.BucketVersioningV2;
* import com.pulumi.aws.s3.BucketVersioningV2Args;
* import com.pulumi.aws.s3.inputs.BucketVersioningV2VersioningConfigurationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new BucketV2("example", BucketV2Args.builder()
* .bucket("example-bucket")
* .build());
*
* var exampleBucketAclV2 = new BucketAclV2("exampleBucketAclV2", BucketAclV2Args.builder()
* .bucket(example.id())
* .acl("private")
* .build());
*
* var versioningExample = new BucketVersioningV2("versioningExample", BucketVersioningV2Args.builder()
* .bucket(example.id())
* .versioningConfiguration(BucketVersioningV2VersioningConfigurationArgs.builder()
* .status("Enabled")
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### With Versioning Disabled
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.s3.BucketV2;
* import com.pulumi.aws.s3.BucketV2Args;
* import com.pulumi.aws.s3.BucketAclV2;
* import com.pulumi.aws.s3.BucketAclV2Args;
* import com.pulumi.aws.s3.BucketVersioningV2;
* import com.pulumi.aws.s3.BucketVersioningV2Args;
* import com.pulumi.aws.s3.inputs.BucketVersioningV2VersioningConfigurationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new BucketV2("example", BucketV2Args.builder()
* .bucket("example-bucket")
* .build());
*
* var exampleBucketAclV2 = new BucketAclV2("exampleBucketAclV2", BucketAclV2Args.builder()
* .bucket(example.id())
* .acl("private")
* .build());
*
* var versioningExample = new BucketVersioningV2("versioningExample", BucketVersioningV2Args.builder()
* .bucket(example.id())
* .versioningConfiguration(BucketVersioningV2VersioningConfigurationArgs.builder()
* .status("Disabled")
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Object Dependency On Versioning
*
* When you create an object whose `version_id` you need and an `aws.s3.BucketVersioningV2` resource in the same configuration, you are more likely to have success by ensuring the `s3_object` depends either implicitly (see below) or explicitly (i.e., using `depends_on = [aws_s3_bucket_versioning.example]`) on the `aws.s3.BucketVersioningV2` resource.
*
* > **NOTE:** For critical and/or production S3 objects, do not create a bucket, enable versioning, and create an object in the bucket within the same configuration. Doing so will not allow the AWS-recommended 15 minutes between enabling versioning and writing to the bucket.
*
* This example shows the `aws_s3_object.example` depending implicitly on the versioning resource through the reference to `aws_s3_bucket_versioning.example.bucket` to define `bucket`:
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.s3.BucketV2;
* import com.pulumi.aws.s3.BucketV2Args;
* import com.pulumi.aws.s3.BucketVersioningV2;
* import com.pulumi.aws.s3.BucketVersioningV2Args;
* import com.pulumi.aws.s3.inputs.BucketVersioningV2VersioningConfigurationArgs;
* import com.pulumi.aws.s3.BucketObjectv2;
* import com.pulumi.aws.s3.BucketObjectv2Args;
* import com.pulumi.asset.FileAsset;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new BucketV2("example", BucketV2Args.builder()
* .bucket("yotto")
* .build());
*
* var exampleBucketVersioningV2 = new BucketVersioningV2("exampleBucketVersioningV2", BucketVersioningV2Args.builder()
* .bucket(example.id())
* .versioningConfiguration(BucketVersioningV2VersioningConfigurationArgs.builder()
* .status("Enabled")
* .build())
* .build());
*
* var exampleBucketObjectv2 = new BucketObjectv2("exampleBucketObjectv2", BucketObjectv2Args.builder()
* .bucket(exampleBucketVersioningV2.id())
* .key("droeloe")
* .source(new FileAsset("example.txt"))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* If the owner (account ID) of the source bucket differs from the account used to configure the AWS Provider, import using the `bucket` and `expected_bucket_owner` separated by a comma (`,`):
*
* __Using `pulumi import` to import__ S3 bucket versioning using the `bucket` or using the `bucket` and `expected_bucket_owner` separated by a comma (`,`). For example:
*
* If the owner (account ID) of the source bucket is the same account used to configure the AWS Provider, import using the `bucket`:
*
* ```sh
* $ pulumi import aws:s3/bucketVersioningV2:BucketVersioningV2 example bucket-name
* ```
* If the owner (account ID) of the source bucket differs from the account used to configure the AWS Provider, import using the `bucket` and `expected_bucket_owner` separated by a comma (`,`):
*
* ```sh
* $ pulumi import aws:s3/bucketVersioningV2:BucketVersioningV2 example bucket-name,123456789012
* ```
*
*/
@ResourceType(type="aws:s3/bucketVersioningV2:BucketVersioningV2")
public class BucketVersioningV2 extends com.pulumi.resources.CustomResource {
/**
* Name of the S3 bucket.
*
*/
@Export(name="bucket", refs={String.class}, tree="[0]")
private Output bucket;
/**
* @return Name of the S3 bucket.
*
*/
public Output bucket() {
return this.bucket;
}
/**
* Account ID of the expected bucket owner.
*
*/
@Export(name="expectedBucketOwner", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> expectedBucketOwner;
/**
* @return Account ID of the expected bucket owner.
*
*/
public Output> expectedBucketOwner() {
return Codegen.optional(this.expectedBucketOwner);
}
/**
* Concatenation of the authentication device's serial number, a space, and the value that is displayed on your authentication device.
*
*/
@Export(name="mfa", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> mfa;
/**
* @return Concatenation of the authentication device's serial number, a space, and the value that is displayed on your authentication device.
*
*/
public Output> mfa() {
return Codegen.optional(this.mfa);
}
/**
* Configuration block for the versioning parameters. See below.
*
*/
@Export(name="versioningConfiguration", refs={BucketVersioningV2VersioningConfiguration.class}, tree="[0]")
private Output versioningConfiguration;
/**
* @return Configuration block for the versioning parameters. See below.
*
*/
public Output versioningConfiguration() {
return this.versioningConfiguration;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public BucketVersioningV2(String name) {
this(name, BucketVersioningV2Args.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public BucketVersioningV2(String name, BucketVersioningV2Args args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public BucketVersioningV2(String name, BucketVersioningV2Args args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:s3/bucketVersioningV2:BucketVersioningV2", name, args == null ? BucketVersioningV2Args.Empty : args, makeResourceOptions(options, Codegen.empty()));
}
private BucketVersioningV2(String name, Output id, @Nullable BucketVersioningV2State state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:s3/bucketVersioningV2:BucketVersioningV2", name, state, makeResourceOptions(options, id));
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static BucketVersioningV2 get(String name, Output id, @Nullable BucketVersioningV2State state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new BucketVersioningV2(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy