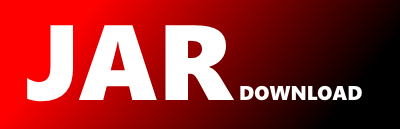
com.pulumi.aws.securityhub.inputs.AccountState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.securityhub.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class AccountState extends com.pulumi.resources.ResourceArgs {
public static final AccountState Empty = new AccountState();
/**
* ARN of the SecurityHub Hub created in the account.
*
*/
@Import(name="arn")
private @Nullable Output arn;
/**
* @return ARN of the SecurityHub Hub created in the account.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy