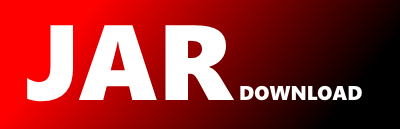
com.pulumi.aws.sns.inputs.PlatformApplicationState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.sns.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class PlatformApplicationState extends com.pulumi.resources.ResourceArgs {
public static final PlatformApplicationState Empty = new PlatformApplicationState();
/**
* The bundle identifier that's assigned to your iOS app. May only include alphanumeric characters, hyphens (-), and periods (.).
*
*/
@Import(name="applePlatformBundleId")
private @Nullable Output applePlatformBundleId;
/**
* @return The bundle identifier that's assigned to your iOS app. May only include alphanumeric characters, hyphens (-), and periods (.).
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy