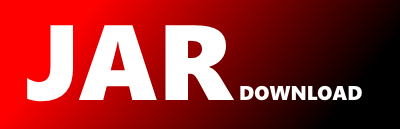
com.pulumi.aws.ssm.Parameter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ssm;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.ssm.ParameterArgs;
import com.pulumi.aws.ssm.inputs.ParameterState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides an SSM Parameter resource.
*
* > **Note:** `overwrite` also makes it possible to overwrite an existing SSM Parameter that's not created by the provider before. This argument has been deprecated and will be removed in v6.0.0 of the provider. For more information on how this affects the behavior of this resource, see this issue comment.
*
* ## Example Usage
*
* ### Basic example
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ssm.Parameter;
* import com.pulumi.aws.ssm.ParameterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var foo = new Parameter("foo", ParameterArgs.builder()
* .name("foo")
* .type("String")
* .value("bar")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Encrypted string using default SSM KMS key
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.rds.Instance;
* import com.pulumi.aws.rds.InstanceArgs;
* import com.pulumi.aws.ssm.Parameter;
* import com.pulumi.aws.ssm.ParameterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var default_ = new Instance("default", InstanceArgs.builder()
* .allocatedStorage(10)
* .storageType("gp2")
* .engine("mysql")
* .engineVersion("5.7.16")
* .instanceClass("db.t2.micro")
* .dbName("mydb")
* .username("foo")
* .password(databaseMasterPassword)
* .dbSubnetGroupName("my_database_subnet_group")
* .parameterGroupName("default.mysql5.7")
* .build());
*
* var secret = new Parameter("secret", ParameterArgs.builder()
* .name("/production/database/password/master")
* .description("The parameter description")
* .type("SecureString")
* .value(databaseMasterPassword)
* .tags(Map.of("environment", "production"))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import SSM Parameters using the parameter store `name`. For example:
*
* ```sh
* $ pulumi import aws:ssm/parameter:Parameter my_param /my_path/my_paramname
* ```
*
*/
@ResourceType(type="aws:ssm/parameter:Parameter")
public class Parameter extends com.pulumi.resources.CustomResource {
/**
* Regular expression used to validate the parameter value.
*
*/
@Export(name="allowedPattern", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> allowedPattern;
/**
* @return Regular expression used to validate the parameter value.
*
*/
public Output> allowedPattern() {
return Codegen.optional(this.allowedPattern);
}
/**
* ARN of the parameter.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return ARN of the parameter.
*
*/
public Output arn() {
return this.arn;
}
/**
* Data type of the parameter. Valid values: `text`, `aws:ssm:integration` and `aws:ec2:image` for AMI format, see the [Native parameter support for Amazon Machine Image IDs](https://docs.aws.amazon.com/systems-manager/latest/userguide/parameter-store-ec2-aliases.html).
*
*/
@Export(name="dataType", refs={String.class}, tree="[0]")
private Output dataType;
/**
* @return Data type of the parameter. Valid values: `text`, `aws:ssm:integration` and `aws:ec2:image` for AMI format, see the [Native parameter support for Amazon Machine Image IDs](https://docs.aws.amazon.com/systems-manager/latest/userguide/parameter-store-ec2-aliases.html).
*
*/
public Output dataType() {
return this.dataType;
}
/**
* Description of the parameter.
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> description;
/**
* @return Description of the parameter.
*
*/
public Output> description() {
return Codegen.optional(this.description);
}
/**
* Value of the parameter. **Use caution:** This value is _never_ marked as sensitive in the pulumi preview output. This argument is not valid with a `type` of `SecureString`.
*
*/
@Export(name="insecureValue", refs={String.class}, tree="[0]")
private Output insecureValue;
/**
* @return Value of the parameter. **Use caution:** This value is _never_ marked as sensitive in the pulumi preview output. This argument is not valid with a `type` of `SecureString`.
*
*/
public Output insecureValue() {
return this.insecureValue;
}
/**
* KMS key ID or ARN for encrypting a SecureString.
*
*/
@Export(name="keyId", refs={String.class}, tree="[0]")
private Output keyId;
/**
* @return KMS key ID or ARN for encrypting a SecureString.
*
*/
public Output keyId() {
return this.keyId;
}
/**
* Name of the parameter. If the name contains a path (e.g., any forward slashes (`/`)), it must be fully qualified with a leading forward slash (`/`). For additional requirements and constraints, see the [AWS SSM User Guide](https://docs.aws.amazon.com/systems-manager/latest/userguide/sysman-parameter-name-constraints.html).
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Name of the parameter. If the name contains a path (e.g., any forward slashes (`/`)), it must be fully qualified with a leading forward slash (`/`). For additional requirements and constraints, see the [AWS SSM User Guide](https://docs.aws.amazon.com/systems-manager/latest/userguide/sysman-parameter-name-constraints.html).
*
*/
public Output name() {
return this.name;
}
/**
* Overwrite an existing parameter. If not specified, defaults to `false` if the resource has not been created by Pulumi to avoid overwrite of existing resource, and will default to `true` otherwise (Pulumi lifecycle rules should then be used to manage the update behavior).
*
* @deprecated
* this attribute has been deprecated
*
*/
@Deprecated /* this attribute has been deprecated */
@Export(name="overwrite", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> overwrite;
/**
* @return Overwrite an existing parameter. If not specified, defaults to `false` if the resource has not been created by Pulumi to avoid overwrite of existing resource, and will default to `true` otherwise (Pulumi lifecycle rules should then be used to manage the update behavior).
*
*/
public Output> overwrite() {
return Codegen.optional(this.overwrite);
}
/**
* Map of tags to assign to the object. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Map of tags to assign to the object. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* Map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy