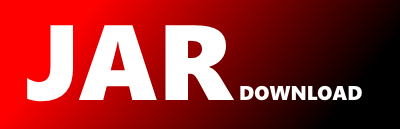
com.pulumi.aws.ssmincidents.outputs.ResponsePlanIncidentTemplate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ssmincidents.outputs;
import com.pulumi.aws.ssmincidents.outputs.ResponsePlanIncidentTemplateNotificationTarget;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ResponsePlanIncidentTemplate {
/**
* @return A string used to stop Incident Manager from creating multiple incident records for the same incident.
*
*/
private @Nullable String dedupeString;
/**
* @return The impact value of a generated incident. The following values are supported:
*
*/
private Integer impact;
/**
* @return The tags assigned to an incident template. When an incident starts, Incident Manager assigns the tags specified in the template to the incident.
*
*/
private @Nullable Map incidentTags;
/**
* @return The Amazon Simple Notification Service (Amazon SNS) targets that this incident notifies when it is updated. The `notification_target` configuration block supports the following argument:
*
*/
private @Nullable List notificationTargets;
/**
* @return The summary of an incident.
*
*/
private @Nullable String summary;
/**
* @return The title of a generated incident.
*
*/
private String title;
private ResponsePlanIncidentTemplate() {}
/**
* @return A string used to stop Incident Manager from creating multiple incident records for the same incident.
*
*/
public Optional dedupeString() {
return Optional.ofNullable(this.dedupeString);
}
/**
* @return The impact value of a generated incident. The following values are supported:
*
*/
public Integer impact() {
return this.impact;
}
/**
* @return The tags assigned to an incident template. When an incident starts, Incident Manager assigns the tags specified in the template to the incident.
*
*/
public Map incidentTags() {
return this.incidentTags == null ? Map.of() : this.incidentTags;
}
/**
* @return The Amazon Simple Notification Service (Amazon SNS) targets that this incident notifies when it is updated. The `notification_target` configuration block supports the following argument:
*
*/
public List notificationTargets() {
return this.notificationTargets == null ? List.of() : this.notificationTargets;
}
/**
* @return The summary of an incident.
*
*/
public Optional summary() {
return Optional.ofNullable(this.summary);
}
/**
* @return The title of a generated incident.
*
*/
public String title() {
return this.title;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ResponsePlanIncidentTemplate defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String dedupeString;
private Integer impact;
private @Nullable Map incidentTags;
private @Nullable List notificationTargets;
private @Nullable String summary;
private String title;
public Builder() {}
public Builder(ResponsePlanIncidentTemplate defaults) {
Objects.requireNonNull(defaults);
this.dedupeString = defaults.dedupeString;
this.impact = defaults.impact;
this.incidentTags = defaults.incidentTags;
this.notificationTargets = defaults.notificationTargets;
this.summary = defaults.summary;
this.title = defaults.title;
}
@CustomType.Setter
public Builder dedupeString(@Nullable String dedupeString) {
this.dedupeString = dedupeString;
return this;
}
@CustomType.Setter
public Builder impact(Integer impact) {
if (impact == null) {
throw new MissingRequiredPropertyException("ResponsePlanIncidentTemplate", "impact");
}
this.impact = impact;
return this;
}
@CustomType.Setter
public Builder incidentTags(@Nullable Map incidentTags) {
this.incidentTags = incidentTags;
return this;
}
@CustomType.Setter
public Builder notificationTargets(@Nullable List notificationTargets) {
this.notificationTargets = notificationTargets;
return this;
}
public Builder notificationTargets(ResponsePlanIncidentTemplateNotificationTarget... notificationTargets) {
return notificationTargets(List.of(notificationTargets));
}
@CustomType.Setter
public Builder summary(@Nullable String summary) {
this.summary = summary;
return this;
}
@CustomType.Setter
public Builder title(String title) {
if (title == null) {
throw new MissingRequiredPropertyException("ResponsePlanIncidentTemplate", "title");
}
this.title = title;
return this;
}
public ResponsePlanIncidentTemplate build() {
final var _resultValue = new ResponsePlanIncidentTemplate();
_resultValue.dedupeString = dedupeString;
_resultValue.impact = impact;
_resultValue.incidentTags = incidentTags;
_resultValue.notificationTargets = notificationTargets;
_resultValue.summary = summary;
_resultValue.title = title;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy