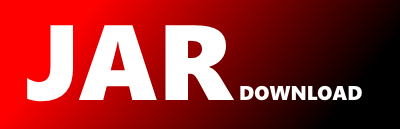
com.pulumi.aws.transcribe.MedicalVocabulary Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.transcribe;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.transcribe.MedicalVocabularyArgs;
import com.pulumi.aws.transcribe.inputs.MedicalVocabularyState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Resource for managing an AWS Transcribe MedicalVocabulary.
*
* ## Example Usage
*
* ### Basic Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.s3.BucketV2;
* import com.pulumi.aws.s3.BucketV2Args;
* import com.pulumi.aws.s3.BucketObjectv2;
* import com.pulumi.aws.s3.BucketObjectv2Args;
* import com.pulumi.aws.transcribe.MedicalVocabulary;
* import com.pulumi.aws.transcribe.MedicalVocabularyArgs;
* import com.pulumi.resources.CustomResourceOptions;
* import com.pulumi.asset.FileAsset;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new BucketV2("example", BucketV2Args.builder()
* .bucket("example-medical-vocab-123")
* .forceDestroy(true)
* .build());
*
* var object = new BucketObjectv2("object", BucketObjectv2Args.builder()
* .bucket(example.id())
* .key("transcribe/test1.txt")
* .source(new FileAsset("test.txt"))
* .build());
*
* var exampleMedicalVocabulary = new MedicalVocabulary("exampleMedicalVocabulary", MedicalVocabularyArgs.builder()
* .vocabularyName("example")
* .languageCode("en-US")
* .vocabularyFileUri(Output.tuple(example.id(), object.key()).applyValue(values -> {
* var id = values.t1;
* var key = values.t2;
* return String.format("s3://%s/%s", id,key);
* }))
* .tags(Map.ofEntries(
* Map.entry("tag1", "value1"),
* Map.entry("tag2", "value3")
* ))
* .build(), CustomResourceOptions.builder()
* .dependsOn(object)
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import Transcribe MedicalVocabulary using the `vocabulary_name`. For example:
*
* ```sh
* $ pulumi import aws:transcribe/medicalVocabulary:MedicalVocabulary example example-name
* ```
*
*/
@ResourceType(type="aws:transcribe/medicalVocabulary:MedicalVocabulary")
public class MedicalVocabulary extends com.pulumi.resources.CustomResource {
/**
* ARN of the MedicalVocabulary.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return ARN of the MedicalVocabulary.
*
*/
public Output arn() {
return this.arn;
}
/**
* Generated download URI.
*
*/
@Export(name="downloadUri", refs={String.class}, tree="[0]")
private Output downloadUri;
/**
* @return Generated download URI.
*
*/
public Output downloadUri() {
return this.downloadUri;
}
/**
* The language code you selected for your medical vocabulary. US English (en-US) is the only language supported with Amazon Transcribe Medical.
*
*/
@Export(name="languageCode", refs={String.class}, tree="[0]")
private Output languageCode;
/**
* @return The language code you selected for your medical vocabulary. US English (en-US) is the only language supported with Amazon Transcribe Medical.
*
*/
public Output languageCode() {
return this.languageCode;
}
/**
* A map of tags to assign to the MedicalVocabulary. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return A map of tags to assign to the MedicalVocabulary. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy