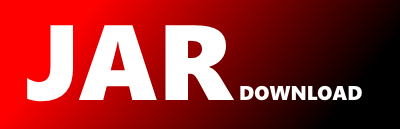
com.pulumi.aws.wafv2.outputs.WebAclRuleStatementSqliMatchStatementFieldToMatch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.wafv2.outputs;
import com.pulumi.aws.wafv2.outputs.WebAclRuleStatementSqliMatchStatementFieldToMatchAllQueryArguments;
import com.pulumi.aws.wafv2.outputs.WebAclRuleStatementSqliMatchStatementFieldToMatchBody;
import com.pulumi.aws.wafv2.outputs.WebAclRuleStatementSqliMatchStatementFieldToMatchCookies;
import com.pulumi.aws.wafv2.outputs.WebAclRuleStatementSqliMatchStatementFieldToMatchHeader;
import com.pulumi.aws.wafv2.outputs.WebAclRuleStatementSqliMatchStatementFieldToMatchHeaderOrder;
import com.pulumi.aws.wafv2.outputs.WebAclRuleStatementSqliMatchStatementFieldToMatchJa3Fingerprint;
import com.pulumi.aws.wafv2.outputs.WebAclRuleStatementSqliMatchStatementFieldToMatchJsonBody;
import com.pulumi.aws.wafv2.outputs.WebAclRuleStatementSqliMatchStatementFieldToMatchMethod;
import com.pulumi.aws.wafv2.outputs.WebAclRuleStatementSqliMatchStatementFieldToMatchQueryString;
import com.pulumi.aws.wafv2.outputs.WebAclRuleStatementSqliMatchStatementFieldToMatchSingleHeader;
import com.pulumi.aws.wafv2.outputs.WebAclRuleStatementSqliMatchStatementFieldToMatchSingleQueryArgument;
import com.pulumi.aws.wafv2.outputs.WebAclRuleStatementSqliMatchStatementFieldToMatchUriPath;
import com.pulumi.core.annotations.CustomType;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class WebAclRuleStatementSqliMatchStatementFieldToMatch {
/**
* @return Inspect all query arguments.
*
*/
private @Nullable WebAclRuleStatementSqliMatchStatementFieldToMatchAllQueryArguments allQueryArguments;
/**
* @return Inspect the request body, which immediately follows the request headers. See `body` below for details.
*
*/
private @Nullable WebAclRuleStatementSqliMatchStatementFieldToMatchBody body;
/**
* @return Inspect the cookies in the web request. See `cookies` below for details.
*
*/
private @Nullable WebAclRuleStatementSqliMatchStatementFieldToMatchCookies cookies;
/**
* @return Inspect a string containing the list of the request's header names, ordered as they appear in the web request that AWS WAF receives for inspection. See `header_order` below for details.
*
*/
private @Nullable List headerOrders;
/**
* @return Inspect the request headers. See `headers` below for details.
*
*/
private @Nullable List headers;
/**
* @return Inspect the JA3 fingerprint. See `ja3_fingerprint` below for details.
*
*/
private @Nullable WebAclRuleStatementSqliMatchStatementFieldToMatchJa3Fingerprint ja3Fingerprint;
/**
* @return Inspect the request body as JSON. See `json_body` for details.
*
*/
private @Nullable WebAclRuleStatementSqliMatchStatementFieldToMatchJsonBody jsonBody;
/**
* @return Inspect the HTTP method. The method indicates the type of operation that the request is asking the origin to perform.
*
*/
private @Nullable WebAclRuleStatementSqliMatchStatementFieldToMatchMethod method;
/**
* @return Inspect the query string. This is the part of a URL that appears after a `?` character, if any.
*
*/
private @Nullable WebAclRuleStatementSqliMatchStatementFieldToMatchQueryString queryString;
/**
* @return Inspect a single header. See `single_header` below for details.
*
*/
private @Nullable WebAclRuleStatementSqliMatchStatementFieldToMatchSingleHeader singleHeader;
/**
* @return Inspect a single query argument. See `single_query_argument` below for details.
*
*/
private @Nullable WebAclRuleStatementSqliMatchStatementFieldToMatchSingleQueryArgument singleQueryArgument;
/**
* @return Inspect the request URI path. This is the part of a web request that identifies a resource, for example, `/images/daily-ad.jpg`.
*
*/
private @Nullable WebAclRuleStatementSqliMatchStatementFieldToMatchUriPath uriPath;
private WebAclRuleStatementSqliMatchStatementFieldToMatch() {}
/**
* @return Inspect all query arguments.
*
*/
public Optional allQueryArguments() {
return Optional.ofNullable(this.allQueryArguments);
}
/**
* @return Inspect the request body, which immediately follows the request headers. See `body` below for details.
*
*/
public Optional body() {
return Optional.ofNullable(this.body);
}
/**
* @return Inspect the cookies in the web request. See `cookies` below for details.
*
*/
public Optional cookies() {
return Optional.ofNullable(this.cookies);
}
/**
* @return Inspect a string containing the list of the request's header names, ordered as they appear in the web request that AWS WAF receives for inspection. See `header_order` below for details.
*
*/
public List headerOrders() {
return this.headerOrders == null ? List.of() : this.headerOrders;
}
/**
* @return Inspect the request headers. See `headers` below for details.
*
*/
public List headers() {
return this.headers == null ? List.of() : this.headers;
}
/**
* @return Inspect the JA3 fingerprint. See `ja3_fingerprint` below for details.
*
*/
public Optional ja3Fingerprint() {
return Optional.ofNullable(this.ja3Fingerprint);
}
/**
* @return Inspect the request body as JSON. See `json_body` for details.
*
*/
public Optional jsonBody() {
return Optional.ofNullable(this.jsonBody);
}
/**
* @return Inspect the HTTP method. The method indicates the type of operation that the request is asking the origin to perform.
*
*/
public Optional method() {
return Optional.ofNullable(this.method);
}
/**
* @return Inspect the query string. This is the part of a URL that appears after a `?` character, if any.
*
*/
public Optional queryString() {
return Optional.ofNullable(this.queryString);
}
/**
* @return Inspect a single header. See `single_header` below for details.
*
*/
public Optional singleHeader() {
return Optional.ofNullable(this.singleHeader);
}
/**
* @return Inspect a single query argument. See `single_query_argument` below for details.
*
*/
public Optional singleQueryArgument() {
return Optional.ofNullable(this.singleQueryArgument);
}
/**
* @return Inspect the request URI path. This is the part of a web request that identifies a resource, for example, `/images/daily-ad.jpg`.
*
*/
public Optional uriPath() {
return Optional.ofNullable(this.uriPath);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(WebAclRuleStatementSqliMatchStatementFieldToMatch defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable WebAclRuleStatementSqliMatchStatementFieldToMatchAllQueryArguments allQueryArguments;
private @Nullable WebAclRuleStatementSqliMatchStatementFieldToMatchBody body;
private @Nullable WebAclRuleStatementSqliMatchStatementFieldToMatchCookies cookies;
private @Nullable List headerOrders;
private @Nullable List headers;
private @Nullable WebAclRuleStatementSqliMatchStatementFieldToMatchJa3Fingerprint ja3Fingerprint;
private @Nullable WebAclRuleStatementSqliMatchStatementFieldToMatchJsonBody jsonBody;
private @Nullable WebAclRuleStatementSqliMatchStatementFieldToMatchMethod method;
private @Nullable WebAclRuleStatementSqliMatchStatementFieldToMatchQueryString queryString;
private @Nullable WebAclRuleStatementSqliMatchStatementFieldToMatchSingleHeader singleHeader;
private @Nullable WebAclRuleStatementSqliMatchStatementFieldToMatchSingleQueryArgument singleQueryArgument;
private @Nullable WebAclRuleStatementSqliMatchStatementFieldToMatchUriPath uriPath;
public Builder() {}
public Builder(WebAclRuleStatementSqliMatchStatementFieldToMatch defaults) {
Objects.requireNonNull(defaults);
this.allQueryArguments = defaults.allQueryArguments;
this.body = defaults.body;
this.cookies = defaults.cookies;
this.headerOrders = defaults.headerOrders;
this.headers = defaults.headers;
this.ja3Fingerprint = defaults.ja3Fingerprint;
this.jsonBody = defaults.jsonBody;
this.method = defaults.method;
this.queryString = defaults.queryString;
this.singleHeader = defaults.singleHeader;
this.singleQueryArgument = defaults.singleQueryArgument;
this.uriPath = defaults.uriPath;
}
@CustomType.Setter
public Builder allQueryArguments(@Nullable WebAclRuleStatementSqliMatchStatementFieldToMatchAllQueryArguments allQueryArguments) {
this.allQueryArguments = allQueryArguments;
return this;
}
@CustomType.Setter
public Builder body(@Nullable WebAclRuleStatementSqliMatchStatementFieldToMatchBody body) {
this.body = body;
return this;
}
@CustomType.Setter
public Builder cookies(@Nullable WebAclRuleStatementSqliMatchStatementFieldToMatchCookies cookies) {
this.cookies = cookies;
return this;
}
@CustomType.Setter
public Builder headerOrders(@Nullable List headerOrders) {
this.headerOrders = headerOrders;
return this;
}
public Builder headerOrders(WebAclRuleStatementSqliMatchStatementFieldToMatchHeaderOrder... headerOrders) {
return headerOrders(List.of(headerOrders));
}
@CustomType.Setter
public Builder headers(@Nullable List headers) {
this.headers = headers;
return this;
}
public Builder headers(WebAclRuleStatementSqliMatchStatementFieldToMatchHeader... headers) {
return headers(List.of(headers));
}
@CustomType.Setter
public Builder ja3Fingerprint(@Nullable WebAclRuleStatementSqliMatchStatementFieldToMatchJa3Fingerprint ja3Fingerprint) {
this.ja3Fingerprint = ja3Fingerprint;
return this;
}
@CustomType.Setter
public Builder jsonBody(@Nullable WebAclRuleStatementSqliMatchStatementFieldToMatchJsonBody jsonBody) {
this.jsonBody = jsonBody;
return this;
}
@CustomType.Setter
public Builder method(@Nullable WebAclRuleStatementSqliMatchStatementFieldToMatchMethod method) {
this.method = method;
return this;
}
@CustomType.Setter
public Builder queryString(@Nullable WebAclRuleStatementSqliMatchStatementFieldToMatchQueryString queryString) {
this.queryString = queryString;
return this;
}
@CustomType.Setter
public Builder singleHeader(@Nullable WebAclRuleStatementSqliMatchStatementFieldToMatchSingleHeader singleHeader) {
this.singleHeader = singleHeader;
return this;
}
@CustomType.Setter
public Builder singleQueryArgument(@Nullable WebAclRuleStatementSqliMatchStatementFieldToMatchSingleQueryArgument singleQueryArgument) {
this.singleQueryArgument = singleQueryArgument;
return this;
}
@CustomType.Setter
public Builder uriPath(@Nullable WebAclRuleStatementSqliMatchStatementFieldToMatchUriPath uriPath) {
this.uriPath = uriPath;
return this;
}
public WebAclRuleStatementSqliMatchStatementFieldToMatch build() {
final var _resultValue = new WebAclRuleStatementSqliMatchStatementFieldToMatch();
_resultValue.allQueryArguments = allQueryArguments;
_resultValue.body = body;
_resultValue.cookies = cookies;
_resultValue.headerOrders = headerOrders;
_resultValue.headers = headers;
_resultValue.ja3Fingerprint = ja3Fingerprint;
_resultValue.jsonBody = jsonBody;
_resultValue.method = method;
_resultValue.queryString = queryString;
_resultValue.singleHeader = singleHeader;
_resultValue.singleQueryArgument = singleQueryArgument;
_resultValue.uriPath = uriPath;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy