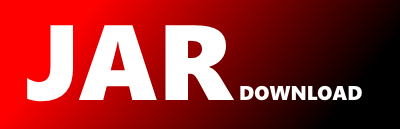
com.pulumi.aws.alb.LoadBalancer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.alb;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.alb.LoadBalancerArgs;
import com.pulumi.aws.alb.inputs.LoadBalancerState;
import com.pulumi.aws.alb.outputs.LoadBalancerAccessLogs;
import com.pulumi.aws.alb.outputs.LoadBalancerConnectionLogs;
import com.pulumi.aws.alb.outputs.LoadBalancerSubnetMapping;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides a Load Balancer resource.
*
* > **Note:** `aws.alb.LoadBalancer` is known as `aws.lb.LoadBalancer`. The functionality is identical.
*
* ## Example Usage
*
* ### Application Load Balancer
*
* <!--Start PulumiCodeChooser -->
* <!--End PulumiCodeChooser -->
*
* ### Network Load Balancer
*
* <!--Start PulumiCodeChooser -->
* <!--End PulumiCodeChooser -->
*
* ### Specifying Elastic IPs
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.lb.LoadBalancer;
* import com.pulumi.aws.lb.LoadBalancerArgs;
* import com.pulumi.aws.lb.inputs.LoadBalancerSubnetMappingArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new LoadBalancer("example", LoadBalancerArgs.builder()
* .name("example")
* .loadBalancerType("network")
* .subnetMappings(
* LoadBalancerSubnetMappingArgs.builder()
* .subnetId(example1AwsSubnet.id())
* .allocationId(example1.id())
* .build(),
* LoadBalancerSubnetMappingArgs.builder()
* .subnetId(example2AwsSubnet.id())
* .allocationId(example2.id())
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Specifying private IP addresses for an internal-facing load balancer
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.lb.LoadBalancer;
* import com.pulumi.aws.lb.LoadBalancerArgs;
* import com.pulumi.aws.lb.inputs.LoadBalancerSubnetMappingArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new LoadBalancer("example", LoadBalancerArgs.builder()
* .name("example")
* .loadBalancerType("network")
* .subnetMappings(
* LoadBalancerSubnetMappingArgs.builder()
* .subnetId(example1.id())
* .privateIpv4Address("10.0.1.15")
* .build(),
* LoadBalancerSubnetMappingArgs.builder()
* .subnetId(example2.id())
* .privateIpv4Address("10.0.2.15")
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import LBs using their ARN. For example:
*
* ```sh
* $ pulumi import aws:alb/loadBalancer:LoadBalancer bar arn:aws:elasticloadbalancing:us-west-2:123456789012:loadbalancer/app/my-load-balancer/50dc6c495c0c9188
* ```
*
*/
@ResourceType(type="aws:alb/loadBalancer:LoadBalancer")
public class LoadBalancer extends com.pulumi.resources.CustomResource {
/**
* Access Logs block. See below.
*
*/
@Export(name="accessLogs", refs={LoadBalancerAccessLogs.class}, tree="[0]")
private Output* @Nullable */ LoadBalancerAccessLogs> accessLogs;
/**
* @return Access Logs block. See below.
*
*/
public Output> accessLogs() {
return Codegen.optional(this.accessLogs);
}
/**
* ARN of the load balancer (matches `id`).
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return ARN of the load balancer (matches `id`).
*
*/
public Output arn() {
return this.arn;
}
/**
* ARN suffix for use with CloudWatch Metrics.
*
*/
@Export(name="arnSuffix", refs={String.class}, tree="[0]")
private Output arnSuffix;
/**
* @return ARN suffix for use with CloudWatch Metrics.
*
*/
public Output arnSuffix() {
return this.arnSuffix;
}
/**
* Client keep alive value in seconds. The valid range is 60-604800 seconds. The default is 3600 seconds.
*
*/
@Export(name="clientKeepAlive", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> clientKeepAlive;
/**
* @return Client keep alive value in seconds. The valid range is 60-604800 seconds. The default is 3600 seconds.
*
*/
public Output> clientKeepAlive() {
return Codegen.optional(this.clientKeepAlive);
}
/**
* Connection Logs block. See below. Only valid for Load Balancers of type `application`.
*
*/
@Export(name="connectionLogs", refs={LoadBalancerConnectionLogs.class}, tree="[0]")
private Output* @Nullable */ LoadBalancerConnectionLogs> connectionLogs;
/**
* @return Connection Logs block. See below. Only valid for Load Balancers of type `application`.
*
*/
public Output> connectionLogs() {
return Codegen.optional(this.connectionLogs);
}
/**
* ID of the customer owned ipv4 pool to use for this load balancer.
*
*/
@Export(name="customerOwnedIpv4Pool", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> customerOwnedIpv4Pool;
/**
* @return ID of the customer owned ipv4 pool to use for this load balancer.
*
*/
public Output> customerOwnedIpv4Pool() {
return Codegen.optional(this.customerOwnedIpv4Pool);
}
/**
* How the load balancer handles requests that might pose a security risk to an application due to HTTP desync. Valid values are `monitor`, `defensive` (default), `strictest`.
*
*/
@Export(name="desyncMitigationMode", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> desyncMitigationMode;
/**
* @return How the load balancer handles requests that might pose a security risk to an application due to HTTP desync. Valid values are `monitor`, `defensive` (default), `strictest`.
*
*/
public Output> desyncMitigationMode() {
return Codegen.optional(this.desyncMitigationMode);
}
/**
* DNS name of the load balancer.
*
*/
@Export(name="dnsName", refs={String.class}, tree="[0]")
private Output dnsName;
/**
* @return DNS name of the load balancer.
*
*/
public Output dnsName() {
return this.dnsName;
}
/**
* How traffic is distributed among the load balancer Availability Zones. Possible values are `any_availability_zone` (default), `availability_zone_affinity`, or `partial_availability_zone_affinity`. See [Availability Zone DNS affinity](https://docs.aws.amazon.com/elasticloadbalancing/latest/network/network-load-balancers.html#zonal-dns-affinity) for additional details. Only valid for `network` type load balancers.
*
*/
@Export(name="dnsRecordClientRoutingPolicy", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> dnsRecordClientRoutingPolicy;
/**
* @return How traffic is distributed among the load balancer Availability Zones. Possible values are `any_availability_zone` (default), `availability_zone_affinity`, or `partial_availability_zone_affinity`. See [Availability Zone DNS affinity](https://docs.aws.amazon.com/elasticloadbalancing/latest/network/network-load-balancers.html#zonal-dns-affinity) for additional details. Only valid for `network` type load balancers.
*
*/
public Output> dnsRecordClientRoutingPolicy() {
return Codegen.optional(this.dnsRecordClientRoutingPolicy);
}
/**
* Whether HTTP headers with header fields that are not valid are removed by the load balancer (true) or routed to targets (false). The default is false. Elastic Load Balancing requires that message header names contain only alphanumeric characters and hyphens. Only valid for Load Balancers of type `application`.
*
*/
@Export(name="dropInvalidHeaderFields", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> dropInvalidHeaderFields;
/**
* @return Whether HTTP headers with header fields that are not valid are removed by the load balancer (true) or routed to targets (false). The default is false. Elastic Load Balancing requires that message header names contain only alphanumeric characters and hyphens. Only valid for Load Balancers of type `application`.
*
*/
public Output> dropInvalidHeaderFields() {
return Codegen.optional(this.dropInvalidHeaderFields);
}
/**
* If true, cross-zone load balancing of the load balancer will be enabled. For `network` and `gateway` type load balancers, this feature is disabled by default (`false`). For `application` load balancer this feature is always enabled (`true`) and cannot be disabled. Defaults to `false`.
*
*/
@Export(name="enableCrossZoneLoadBalancing", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableCrossZoneLoadBalancing;
/**
* @return If true, cross-zone load balancing of the load balancer will be enabled. For `network` and `gateway` type load balancers, this feature is disabled by default (`false`). For `application` load balancer this feature is always enabled (`true`) and cannot be disabled. Defaults to `false`.
*
*/
public Output> enableCrossZoneLoadBalancing() {
return Codegen.optional(this.enableCrossZoneLoadBalancing);
}
/**
* If true, deletion of the load balancer will be disabled via the AWS API. This will prevent this provider from deleting the load balancer. Defaults to `false`.
*
*/
@Export(name="enableDeletionProtection", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableDeletionProtection;
/**
* @return If true, deletion of the load balancer will be disabled via the AWS API. This will prevent this provider from deleting the load balancer. Defaults to `false`.
*
*/
public Output> enableDeletionProtection() {
return Codegen.optional(this.enableDeletionProtection);
}
/**
* Whether HTTP/2 is enabled in `application` load balancers. Defaults to `true`.
*
*/
@Export(name="enableHttp2", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableHttp2;
/**
* @return Whether HTTP/2 is enabled in `application` load balancers. Defaults to `true`.
*
*/
public Output> enableHttp2() {
return Codegen.optional(this.enableHttp2);
}
/**
* Whether the two headers (`x-amzn-tls-version` and `x-amzn-tls-cipher-suite`), which contain information about the negotiated TLS version and cipher suite, are added to the client request before sending it to the target. Only valid for Load Balancers of type `application`. Defaults to `false`
*
*/
@Export(name="enableTlsVersionAndCipherSuiteHeaders", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableTlsVersionAndCipherSuiteHeaders;
/**
* @return Whether the two headers (`x-amzn-tls-version` and `x-amzn-tls-cipher-suite`), which contain information about the negotiated TLS version and cipher suite, are added to the client request before sending it to the target. Only valid for Load Balancers of type `application`. Defaults to `false`
*
*/
public Output> enableTlsVersionAndCipherSuiteHeaders() {
return Codegen.optional(this.enableTlsVersionAndCipherSuiteHeaders);
}
/**
* Whether to allow a WAF-enabled load balancer to route requests to targets if it is unable to forward the request to AWS WAF. Defaults to `false`.
*
*/
@Export(name="enableWafFailOpen", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableWafFailOpen;
/**
* @return Whether to allow a WAF-enabled load balancer to route requests to targets if it is unable to forward the request to AWS WAF. Defaults to `false`.
*
*/
public Output> enableWafFailOpen() {
return Codegen.optional(this.enableWafFailOpen);
}
/**
* Whether the X-Forwarded-For header should preserve the source port that the client used to connect to the load balancer in `application` load balancers. Defaults to `false`.
*
*/
@Export(name="enableXffClientPort", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableXffClientPort;
/**
* @return Whether the X-Forwarded-For header should preserve the source port that the client used to connect to the load balancer in `application` load balancers. Defaults to `false`.
*
*/
public Output> enableXffClientPort() {
return Codegen.optional(this.enableXffClientPort);
}
/**
* Whether inbound security group rules are enforced for traffic originating from a PrivateLink. Only valid for Load Balancers of type `network`. The possible values are `on` and `off`.
*
*/
@Export(name="enforceSecurityGroupInboundRulesOnPrivateLinkTraffic", refs={String.class}, tree="[0]")
private Output enforceSecurityGroupInboundRulesOnPrivateLinkTraffic;
/**
* @return Whether inbound security group rules are enforced for traffic originating from a PrivateLink. Only valid for Load Balancers of type `network`. The possible values are `on` and `off`.
*
*/
public Output enforceSecurityGroupInboundRulesOnPrivateLinkTraffic() {
return this.enforceSecurityGroupInboundRulesOnPrivateLinkTraffic;
}
/**
* Time in seconds that the connection is allowed to be idle. Only valid for Load Balancers of type `application`. Default: 60.
*
*/
@Export(name="idleTimeout", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> idleTimeout;
/**
* @return Time in seconds that the connection is allowed to be idle. Only valid for Load Balancers of type `application`. Default: 60.
*
*/
public Output> idleTimeout() {
return Codegen.optional(this.idleTimeout);
}
/**
* If true, the LB will be internal. Defaults to `false`.
*
*/
@Export(name="internal", refs={Boolean.class}, tree="[0]")
private Output internal;
/**
* @return If true, the LB will be internal. Defaults to `false`.
*
*/
public Output internal() {
return this.internal;
}
/**
* Type of IP addresses used by the subnets for your load balancer. The possible values depend upon the load balancer type: `ipv4` (all load balancer types), `dualstack` (all load balancer types), and `dualstack-without-public-ipv4` (type `application` only).
*
*/
@Export(name="ipAddressType", refs={String.class}, tree="[0]")
private Output ipAddressType;
/**
* @return Type of IP addresses used by the subnets for your load balancer. The possible values depend upon the load balancer type: `ipv4` (all load balancer types), `dualstack` (all load balancer types), and `dualstack-without-public-ipv4` (type `application` only).
*
*/
public Output ipAddressType() {
return this.ipAddressType;
}
/**
* Type of load balancer to create. Possible values are `application`, `gateway`, or `network`. The default value is `application`.
*
*/
@Export(name="loadBalancerType", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> loadBalancerType;
/**
* @return Type of load balancer to create. Possible values are `application`, `gateway`, or `network`. The default value is `application`.
*
*/
public Output> loadBalancerType() {
return Codegen.optional(this.loadBalancerType);
}
/**
* Name of the LB. This name must be unique within your AWS account, can have a maximum of 32 characters, must contain only alphanumeric characters or hyphens, and must not begin or end with a hyphen. If not specified, this provider will autogenerate a name beginning with `tf-lb`.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Name of the LB. This name must be unique within your AWS account, can have a maximum of 32 characters, must contain only alphanumeric characters or hyphens, and must not begin or end with a hyphen. If not specified, this provider will autogenerate a name beginning with `tf-lb`.
*
*/
public Output name() {
return this.name;
}
/**
* Creates a unique name beginning with the specified prefix. Conflicts with `name`.
*
*/
@Export(name="namePrefix", refs={String.class}, tree="[0]")
private Output namePrefix;
/**
* @return Creates a unique name beginning with the specified prefix. Conflicts with `name`.
*
*/
public Output namePrefix() {
return this.namePrefix;
}
/**
* Whether the Application Load Balancer should preserve the Host header in the HTTP request and send it to the target without any change. Defaults to `false`.
*
*/
@Export(name="preserveHostHeader", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> preserveHostHeader;
/**
* @return Whether the Application Load Balancer should preserve the Host header in the HTTP request and send it to the target without any change. Defaults to `false`.
*
*/
public Output> preserveHostHeader() {
return Codegen.optional(this.preserveHostHeader);
}
/**
* List of security group IDs to assign to the LB. Only valid for Load Balancers of type `application` or `network`. For load balancers of type `network` security groups cannot be added if none are currently present, and cannot all be removed once added. If either of these conditions are met, this will force a recreation of the resource.
*
*/
@Export(name="securityGroups", refs={List.class,String.class}, tree="[0,1]")
private Output> securityGroups;
/**
* @return List of security group IDs to assign to the LB. Only valid for Load Balancers of type `application` or `network`. For load balancers of type `network` security groups cannot be added if none are currently present, and cannot all be removed once added. If either of these conditions are met, this will force a recreation of the resource.
*
*/
public Output> securityGroups() {
return this.securityGroups;
}
/**
* Subnet mapping block. See below. For Load Balancers of type `network` subnet mappings can only be added.
*
*/
@Export(name="subnetMappings", refs={List.class,LoadBalancerSubnetMapping.class}, tree="[0,1]")
private Output> subnetMappings;
/**
* @return Subnet mapping block. See below. For Load Balancers of type `network` subnet mappings can only be added.
*
*/
public Output> subnetMappings() {
return this.subnetMappings;
}
/**
* List of subnet IDs to attach to the LB. For Load Balancers of type `network` subnets can only be added (see [Availability Zones](https://docs.aws.amazon.com/elasticloadbalancing/latest/network/network-load-balancers.html#availability-zones)), deleting a subnet for load balancers of type `network` will force a recreation of the resource.
*
*/
@Export(name="subnets", refs={List.class,String.class}, tree="[0,1]")
private Output> subnets;
/**
* @return List of subnet IDs to attach to the LB. For Load Balancers of type `network` subnets can only be added (see [Availability Zones](https://docs.aws.amazon.com/elasticloadbalancing/latest/network/network-load-balancers.html#availability-zones)), deleting a subnet for load balancers of type `network` will force a recreation of the resource.
*
*/
public Output> subnets() {
return this.subnets;
}
/**
* Map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* Map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy