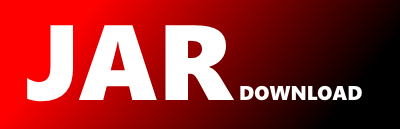
com.pulumi.aws.amp.inputs.WorkspaceState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.amp.inputs;
import com.pulumi.aws.amp.inputs.WorkspaceLoggingConfigurationArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class WorkspaceState extends com.pulumi.resources.ResourceArgs {
public static final WorkspaceState Empty = new WorkspaceState();
/**
* The alias of the prometheus workspace. See more [in AWS Docs](https://docs.aws.amazon.com/prometheus/latest/userguide/AMP-onboard-create-workspace.html).
*
*/
@Import(name="alias")
private @Nullable Output alias;
/**
* @return The alias of the prometheus workspace. See more [in AWS Docs](https://docs.aws.amazon.com/prometheus/latest/userguide/AMP-onboard-create-workspace.html).
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy