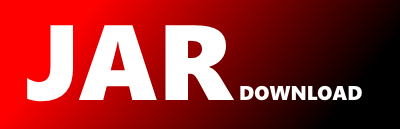
com.pulumi.aws.apigatewayv2.Integration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.apigatewayv2;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.apigatewayv2.IntegrationArgs;
import com.pulumi.aws.apigatewayv2.inputs.IntegrationState;
import com.pulumi.aws.apigatewayv2.outputs.IntegrationResponseParameter;
import com.pulumi.aws.apigatewayv2.outputs.IntegrationTlsConfig;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Manages an Amazon API Gateway Version 2 integration.
* More information can be found in the [Amazon API Gateway Developer Guide](https://docs.aws.amazon.com/apigateway/latest/developerguide/apigateway-websocket-api.html).
*
* ## Example Usage
*
* ### Basic
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.apigatewayv2.Integration;
* import com.pulumi.aws.apigatewayv2.IntegrationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Integration("example", IntegrationArgs.builder()
* .apiId(exampleAwsApigatewayv2Api.id())
* .integrationType("MOCK")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Lambda Integration
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.lambda.Function;
* import com.pulumi.aws.lambda.FunctionArgs;
* import com.pulumi.aws.apigatewayv2.Integration;
* import com.pulumi.aws.apigatewayv2.IntegrationArgs;
* import com.pulumi.asset.FileArchive;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Function("example", FunctionArgs.builder()
* .code(new FileArchive("example.zip"))
* .name("Example")
* .role(exampleAwsIamRole.arn())
* .handler("index.handler")
* .runtime("nodejs16.x")
* .build());
*
* var exampleIntegration = new Integration("exampleIntegration", IntegrationArgs.builder()
* .apiId(exampleAwsApigatewayv2Api.id())
* .integrationType("AWS_PROXY")
* .connectionType("INTERNET")
* .contentHandlingStrategy("CONVERT_TO_TEXT")
* .description("Lambda example")
* .integrationMethod("POST")
* .integrationUri(example.invokeArn())
* .passthroughBehavior("WHEN_NO_MATCH")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### AWS Service Integration
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.apigatewayv2.Integration;
* import com.pulumi.aws.apigatewayv2.IntegrationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Integration("example", IntegrationArgs.builder()
* .apiId(exampleAwsApigatewayv2Api.id())
* .credentialsArn(exampleAwsIamRole.arn())
* .description("SQS example")
* .integrationType("AWS_PROXY")
* .integrationSubtype("SQS-SendMessage")
* .requestParameters(Map.ofEntries(
* Map.entry("QueueUrl", "$request.header.queueUrl"),
* Map.entry("MessageBody", "$request.body.message")
* ))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Private Integration
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.apigatewayv2.Integration;
* import com.pulumi.aws.apigatewayv2.IntegrationArgs;
* import com.pulumi.aws.apigatewayv2.inputs.IntegrationTlsConfigArgs;
* import com.pulumi.aws.apigatewayv2.inputs.IntegrationResponseParameterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Integration("example", IntegrationArgs.builder()
* .apiId(exampleAwsApigatewayv2Api.id())
* .credentialsArn(exampleAwsIamRole.arn())
* .description("Example with a load balancer")
* .integrationType("HTTP_PROXY")
* .integrationUri(exampleAwsLbListener.arn())
* .integrationMethod("ANY")
* .connectionType("VPC_LINK")
* .connectionId(exampleAwsApigatewayv2VpcLink.id())
* .tlsConfig(IntegrationTlsConfigArgs.builder()
* .serverNameToVerify("example.com")
* .build())
* .requestParameters(Map.ofEntries(
* Map.entry("append:header.authforintegration", "$context.authorizer.authorizerResponse"),
* Map.entry("overwrite:path", "staticValueForIntegration")
* ))
* .responseParameters(
* IntegrationResponseParameterArgs.builder()
* .statusCode(403)
* .mappings(Map.of("append:header.auth", "$context.authorizer.authorizerResponse"))
* .build(),
* IntegrationResponseParameterArgs.builder()
* .statusCode(200)
* .mappings(Map.of("overwrite:statuscode", "204"))
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import `aws_apigatewayv2_integration` using the API identifier and integration identifier. For example:
*
* ```sh
* $ pulumi import aws:apigatewayv2/integration:Integration example aabbccddee/1122334
* ```
* -> __Note:__ The API Gateway managed integration created as part of [_quick_create_](https://docs.aws.amazon.com/apigateway/latest/developerguide/api-gateway-basic-concept.html#apigateway-definition-quick-create) cannot be imported.
*
*/
@ResourceType(type="aws:apigatewayv2/integration:Integration")
public class Integration extends com.pulumi.resources.CustomResource {
/**
* API identifier.
*
*/
@Export(name="apiId", refs={String.class}, tree="[0]")
private Output apiId;
/**
* @return API identifier.
*
*/
public Output apiId() {
return this.apiId;
}
/**
* ID of the VPC link for a private integration. Supported only for HTTP APIs. Must be between 1 and 1024 characters in length.
*
*/
@Export(name="connectionId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> connectionId;
/**
* @return ID of the VPC link for a private integration. Supported only for HTTP APIs. Must be between 1 and 1024 characters in length.
*
*/
public Output> connectionId() {
return Codegen.optional(this.connectionId);
}
/**
* Type of the network connection to the integration endpoint. Valid values: `INTERNET`, `VPC_LINK`. Default is `INTERNET`.
*
*/
@Export(name="connectionType", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> connectionType;
/**
* @return Type of the network connection to the integration endpoint. Valid values: `INTERNET`, `VPC_LINK`. Default is `INTERNET`.
*
*/
public Output> connectionType() {
return Codegen.optional(this.connectionType);
}
/**
* How to handle response payload content type conversions. Valid values: `CONVERT_TO_BINARY`, `CONVERT_TO_TEXT`. Supported only for WebSocket APIs.
*
*/
@Export(name="contentHandlingStrategy", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> contentHandlingStrategy;
/**
* @return How to handle response payload content type conversions. Valid values: `CONVERT_TO_BINARY`, `CONVERT_TO_TEXT`. Supported only for WebSocket APIs.
*
*/
public Output> contentHandlingStrategy() {
return Codegen.optional(this.contentHandlingStrategy);
}
/**
* Credentials required for the integration, if any.
*
*/
@Export(name="credentialsArn", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> credentialsArn;
/**
* @return Credentials required for the integration, if any.
*
*/
public Output> credentialsArn() {
return Codegen.optional(this.credentialsArn);
}
/**
* Description of the integration.
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> description;
/**
* @return Description of the integration.
*
*/
public Output> description() {
return Codegen.optional(this.description);
}
/**
* Integration's HTTP method. Must be specified if `integration_type` is not `MOCK`.
*
*/
@Export(name="integrationMethod", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> integrationMethod;
/**
* @return Integration's HTTP method. Must be specified if `integration_type` is not `MOCK`.
*
*/
public Output> integrationMethod() {
return Codegen.optional(this.integrationMethod);
}
/**
* The [integration response selection expression](https://docs.aws.amazon.com/apigateway/latest/developerguide/apigateway-websocket-api-selection-expressions.html#apigateway-websocket-api-integration-response-selection-expressions) for the integration.
*
*/
@Export(name="integrationResponseSelectionExpression", refs={String.class}, tree="[0]")
private Output integrationResponseSelectionExpression;
/**
* @return The [integration response selection expression](https://docs.aws.amazon.com/apigateway/latest/developerguide/apigateway-websocket-api-selection-expressions.html#apigateway-websocket-api-integration-response-selection-expressions) for the integration.
*
*/
public Output integrationResponseSelectionExpression() {
return this.integrationResponseSelectionExpression;
}
/**
* AWS service action to invoke. Supported only for HTTP APIs when `integration_type` is `AWS_PROXY`. See the [AWS service integration reference](https://docs.aws.amazon.com/apigateway/latest/developerguide/http-api-develop-integrations-aws-services-reference.html) documentation for supported values. Must be between 1 and 128 characters in length.
*
*/
@Export(name="integrationSubtype", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> integrationSubtype;
/**
* @return AWS service action to invoke. Supported only for HTTP APIs when `integration_type` is `AWS_PROXY`. See the [AWS service integration reference](https://docs.aws.amazon.com/apigateway/latest/developerguide/http-api-develop-integrations-aws-services-reference.html) documentation for supported values. Must be between 1 and 128 characters in length.
*
*/
public Output> integrationSubtype() {
return Codegen.optional(this.integrationSubtype);
}
/**
* Integration type of an integration.
* Valid values: `AWS` (supported only for WebSocket APIs), `AWS_PROXY`, `HTTP` (supported only for WebSocket APIs), `HTTP_PROXY`, `MOCK` (supported only for WebSocket APIs). For an HTTP API private integration, use `HTTP_PROXY`.
*
*/
@Export(name="integrationType", refs={String.class}, tree="[0]")
private Output integrationType;
/**
* @return Integration type of an integration.
* Valid values: `AWS` (supported only for WebSocket APIs), `AWS_PROXY`, `HTTP` (supported only for WebSocket APIs), `HTTP_PROXY`, `MOCK` (supported only for WebSocket APIs). For an HTTP API private integration, use `HTTP_PROXY`.
*
*/
public Output integrationType() {
return this.integrationType;
}
/**
* URI of the Lambda function for a Lambda proxy integration, when `integration_type` is `AWS_PROXY`.
* For an `HTTP` integration, specify a fully-qualified URL. For an HTTP API private integration, specify the ARN of an Application Load Balancer listener, Network Load Balancer listener, or AWS Cloud Map service.
*
*/
@Export(name="integrationUri", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> integrationUri;
/**
* @return URI of the Lambda function for a Lambda proxy integration, when `integration_type` is `AWS_PROXY`.
* For an `HTTP` integration, specify a fully-qualified URL. For an HTTP API private integration, specify the ARN of an Application Load Balancer listener, Network Load Balancer listener, or AWS Cloud Map service.
*
*/
public Output> integrationUri() {
return Codegen.optional(this.integrationUri);
}
/**
* Pass-through behavior for incoming requests based on the Content-Type header in the request, and the available mapping templates specified as the `request_templates` attribute.
* Valid values: `WHEN_NO_MATCH`, `WHEN_NO_TEMPLATES`, `NEVER`. Default is `WHEN_NO_MATCH`. Supported only for WebSocket APIs.
*
*/
@Export(name="passthroughBehavior", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> passthroughBehavior;
/**
* @return Pass-through behavior for incoming requests based on the Content-Type header in the request, and the available mapping templates specified as the `request_templates` attribute.
* Valid values: `WHEN_NO_MATCH`, `WHEN_NO_TEMPLATES`, `NEVER`. Default is `WHEN_NO_MATCH`. Supported only for WebSocket APIs.
*
*/
public Output> passthroughBehavior() {
return Codegen.optional(this.passthroughBehavior);
}
/**
* The [format of the payload](https://docs.aws.amazon.com/apigateway/latest/developerguide/http-api-develop-integrations-lambda.html#http-api-develop-integrations-lambda.proxy-format) sent to an integration. Valid values: `1.0`, `2.0`. Default is `1.0`.
*
*/
@Export(name="payloadFormatVersion", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> payloadFormatVersion;
/**
* @return The [format of the payload](https://docs.aws.amazon.com/apigateway/latest/developerguide/http-api-develop-integrations-lambda.html#http-api-develop-integrations-lambda.proxy-format) sent to an integration. Valid values: `1.0`, `2.0`. Default is `1.0`.
*
*/
public Output> payloadFormatVersion() {
return Codegen.optional(this.payloadFormatVersion);
}
/**
* For WebSocket APIs, a key-value map specifying request parameters that are passed from the method request to the backend.
* For HTTP APIs with a specified `integration_subtype`, a key-value map specifying parameters that are passed to `AWS_PROXY` integrations.
* For HTTP APIs without a specified `integration_subtype`, a key-value map specifying how to transform HTTP requests before sending them to the backend.
* See the [Amazon API Gateway Developer Guide](https://docs.aws.amazon.com/apigateway/latest/developerguide/http-api-parameter-mapping.html) for details.
*
*/
@Export(name="requestParameters", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> requestParameters;
/**
* @return For WebSocket APIs, a key-value map specifying request parameters that are passed from the method request to the backend.
* For HTTP APIs with a specified `integration_subtype`, a key-value map specifying parameters that are passed to `AWS_PROXY` integrations.
* For HTTP APIs without a specified `integration_subtype`, a key-value map specifying how to transform HTTP requests before sending them to the backend.
* See the [Amazon API Gateway Developer Guide](https://docs.aws.amazon.com/apigateway/latest/developerguide/http-api-parameter-mapping.html) for details.
*
*/
public Output>> requestParameters() {
return Codegen.optional(this.requestParameters);
}
/**
* Map of [Velocity](https://velocity.apache.org/) templates that are applied on the request payload based on the value of the Content-Type header sent by the client. Supported only for WebSocket APIs.
*
*/
@Export(name="requestTemplates", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> requestTemplates;
/**
* @return Map of [Velocity](https://velocity.apache.org/) templates that are applied on the request payload based on the value of the Content-Type header sent by the client. Supported only for WebSocket APIs.
*
*/
public Output>> requestTemplates() {
return Codegen.optional(this.requestTemplates);
}
/**
* Mappings to transform the HTTP response from a backend integration before returning the response to clients. Supported only for HTTP APIs.
*
*/
@Export(name="responseParameters", refs={List.class,IntegrationResponseParameter.class}, tree="[0,1]")
private Output* @Nullable */ List> responseParameters;
/**
* @return Mappings to transform the HTTP response from a backend integration before returning the response to clients. Supported only for HTTP APIs.
*
*/
public Output>> responseParameters() {
return Codegen.optional(this.responseParameters);
}
/**
* The [template selection expression](https://docs.aws.amazon.com/apigateway/latest/developerguide/apigateway-websocket-api-selection-expressions.html#apigateway-websocket-api-template-selection-expressions) for the integration.
*
*/
@Export(name="templateSelectionExpression", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> templateSelectionExpression;
/**
* @return The [template selection expression](https://docs.aws.amazon.com/apigateway/latest/developerguide/apigateway-websocket-api-selection-expressions.html#apigateway-websocket-api-template-selection-expressions) for the integration.
*
*/
public Output> templateSelectionExpression() {
return Codegen.optional(this.templateSelectionExpression);
}
/**
* Custom timeout between 50 and 29,000 milliseconds for WebSocket APIs and between 50 and 30,000 milliseconds for HTTP APIs.
* The default timeout is 29 seconds for WebSocket APIs and 30 seconds for HTTP APIs.
* this provider will only perform drift detection of its value when present in a configuration.
*
*/
@Export(name="timeoutMilliseconds", refs={Integer.class}, tree="[0]")
private Output timeoutMilliseconds;
/**
* @return Custom timeout between 50 and 29,000 milliseconds for WebSocket APIs and between 50 and 30,000 milliseconds for HTTP APIs.
* The default timeout is 29 seconds for WebSocket APIs and 30 seconds for HTTP APIs.
* this provider will only perform drift detection of its value when present in a configuration.
*
*/
public Output timeoutMilliseconds() {
return this.timeoutMilliseconds;
}
/**
* TLS configuration for a private integration. Supported only for HTTP APIs.
*
*/
@Export(name="tlsConfig", refs={IntegrationTlsConfig.class}, tree="[0]")
private Output* @Nullable */ IntegrationTlsConfig> tlsConfig;
/**
* @return TLS configuration for a private integration. Supported only for HTTP APIs.
*
*/
public Output> tlsConfig() {
return Codegen.optional(this.tlsConfig);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Integration(java.lang.String name) {
this(name, IntegrationArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Integration(java.lang.String name, IntegrationArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Integration(java.lang.String name, IntegrationArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:apigatewayv2/integration:Integration", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Integration(java.lang.String name, Output id, @Nullable IntegrationState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:apigatewayv2/integration:Integration", name, state, makeResourceOptions(options, id), false);
}
private static IntegrationArgs makeArgs(IntegrationArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? IntegrationArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Integration get(java.lang.String name, Output id, @Nullable IntegrationState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Integration(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy