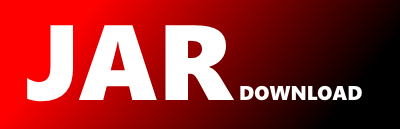
com.pulumi.aws.apigatewayv2.inputs.GetExportArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.apigatewayv2.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class GetExportArgs extends com.pulumi.resources.InvokeArgs {
public static final GetExportArgs Empty = new GetExportArgs();
/**
* API identifier.
*
*/
@Import(name="apiId", required=true)
private Output apiId;
/**
* @return API identifier.
*
*/
public Output apiId() {
return this.apiId;
}
/**
* Version of the API Gateway export algorithm. API Gateway uses the latest version by default. Currently, the only supported version is `1.0`.
*
*/
@Import(name="exportVersion")
private @Nullable Output exportVersion;
/**
* @return Version of the API Gateway export algorithm. API Gateway uses the latest version by default. Currently, the only supported version is `1.0`.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy