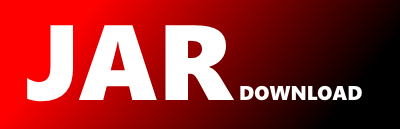
com.pulumi.aws.appconfig.Extension Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.appconfig;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.appconfig.ExtensionArgs;
import com.pulumi.aws.appconfig.inputs.ExtensionState;
import com.pulumi.aws.appconfig.outputs.ExtensionActionPoint;
import com.pulumi.aws.appconfig.outputs.ExtensionParameter;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides an AppConfig Extension resource.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.sns.Topic;
* import com.pulumi.aws.sns.TopicArgs;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyDocumentArgs;
* import com.pulumi.aws.iam.Role;
* import com.pulumi.aws.iam.RoleArgs;
* import com.pulumi.aws.appconfig.Extension;
* import com.pulumi.aws.appconfig.ExtensionArgs;
* import com.pulumi.aws.appconfig.inputs.ExtensionActionPointArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var testTopic = new Topic("testTopic", TopicArgs.builder()
* .name("test")
* .build());
*
* final var test = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .actions("sts:AssumeRole")
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .type("Service")
* .identifiers("appconfig.amazonaws.com")
* .build())
* .build())
* .build());
*
* var testRole = new Role("testRole", RoleArgs.builder()
* .name("test")
* .assumeRolePolicy(test.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json()))
* .build());
*
* var testExtension = new Extension("testExtension", ExtensionArgs.builder()
* .name("test")
* .description("test description")
* .actionPoints(ExtensionActionPointArgs.builder()
* .point("ON_DEPLOYMENT_COMPLETE")
* .actions(ExtensionActionPointActionArgs.builder()
* .name("test")
* .roleArn(testRole.arn())
* .uri(testTopic.arn())
* .build())
* .build())
* .tags(Map.of("Type", "AppConfig Extension"))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import AppConfig Extensions using their extension ID. For example:
*
* ```sh
* $ pulumi import aws:appconfig/extension:Extension example 71rxuzt
* ```
*
*/
@ResourceType(type="aws:appconfig/extension:Extension")
public class Extension extends com.pulumi.resources.CustomResource {
/**
* The action points defined in the extension. Detailed below.
*
*/
@Export(name="actionPoints", refs={List.class,ExtensionActionPoint.class}, tree="[0,1]")
private Output> actionPoints;
/**
* @return The action points defined in the extension. Detailed below.
*
*/
public Output> actionPoints() {
return this.actionPoints;
}
/**
* ARN of the AppConfig Extension.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return ARN of the AppConfig Extension.
*
*/
public Output arn() {
return this.arn;
}
/**
* Information about the extension.
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output description;
/**
* @return Information about the extension.
*
*/
public Output description() {
return this.description;
}
/**
* A name for the extension. Each extension name in your account must be unique. Extension versions use the same name.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return A name for the extension. Each extension name in your account must be unique. Extension versions use the same name.
*
*/
public Output name() {
return this.name;
}
/**
* The parameters accepted by the extension. You specify parameter values when you associate the extension to an AppConfig resource by using the CreateExtensionAssociation API action. For Lambda extension actions, these parameters are included in the Lambda request object. Detailed below.
*
*/
@Export(name="parameters", refs={List.class,ExtensionParameter.class}, tree="[0,1]")
private Output> parameters;
/**
* @return The parameters accepted by the extension. You specify parameter values when you associate the extension to an AppConfig resource by using the CreateExtensionAssociation API action. For Lambda extension actions, these parameters are included in the Lambda request object. Detailed below.
*
*/
public Output> parameters() {
return this.parameters;
}
/**
* Map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy